filmov
tv
GFG Print matrix in diagonal pattern || Easy ||Matrices ||C++ ||POTD
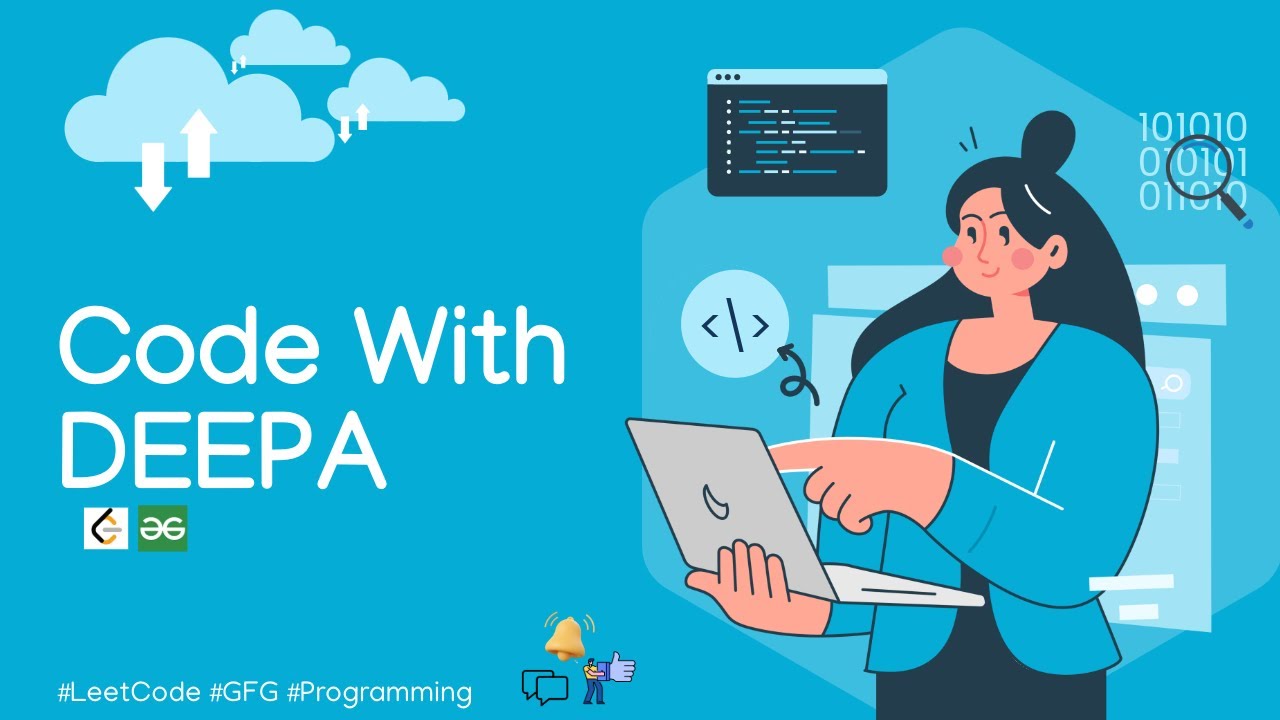
Показать описание
In this video, we will solve a problem that involves determining the diagonal pattern of a square matrix. The diagonal pattern is a linear arrangement of the elements of the matrix, which follows a specific traversal pattern. We will use a two-dimensional vector to represent the matrix and then traverse it to extract the diagonal pattern. 🔍
Steps to Solve:
Initialize Variables: Initialize variables n, ans, k, r, c, and up. n is the size of the matrix, ans is a vector to store the diagonal pattern, k is the index of ans, r and c are the row and column indices, and up is a flag to indicate the traversal direction. 🛠️
Traverse the Matrix: Use a while loop to traverse the matrix while r and c are within bounds. 🚶♂️
Traverse Upwards: If up is true, traverse diagonally upwards until r reaches 0 or c reaches n-1. Store each element in ans and update r and c accordingly. 🔄
Update Direction: If up is true and c reaches n-1, increment r to move to the next row; otherwise, increment c to move to the next column. 🔄
Traverse Downwards: If up is false, traverse diagonally downwards until c reaches 0 or r reaches n-1. Store each element in ans and update r and c accordingly. 🔄
Update Direction Again: If up is false and r reaches n-1, increment c to move to the next column; otherwise, increment r to move to the next row. 🔄
Toggle Direction: Toggle the value of up after each traversal to switch between upwards and downwards traversal. 🔄
Return the Diagonal Pattern: Return the ans vector containing the diagonal pattern of the matrix. 🔄
Code Explanation:
The matrixDiagonally function takes a 2D vector mat as input. 🔄
It initializes variables n, ans, k, r, c, and up. 🔄
It traverses the matrix diagonally, storing elements in ans based on the traversal direction. 🔄
It toggles the traversal direction after each diagonal traversal. 🔄
Finally, it returns the ans vector containing the diagonal pattern. 🔄
#geeksforgeeks #codinginterview #leetcode #like #comment #subscribe #support
Steps to Solve:
Initialize Variables: Initialize variables n, ans, k, r, c, and up. n is the size of the matrix, ans is a vector to store the diagonal pattern, k is the index of ans, r and c are the row and column indices, and up is a flag to indicate the traversal direction. 🛠️
Traverse the Matrix: Use a while loop to traverse the matrix while r and c are within bounds. 🚶♂️
Traverse Upwards: If up is true, traverse diagonally upwards until r reaches 0 or c reaches n-1. Store each element in ans and update r and c accordingly. 🔄
Update Direction: If up is true and c reaches n-1, increment r to move to the next row; otherwise, increment c to move to the next column. 🔄
Traverse Downwards: If up is false, traverse diagonally downwards until c reaches 0 or r reaches n-1. Store each element in ans and update r and c accordingly. 🔄
Update Direction Again: If up is false and r reaches n-1, increment c to move to the next column; otherwise, increment r to move to the next row. 🔄
Toggle Direction: Toggle the value of up after each traversal to switch between upwards and downwards traversal. 🔄
Return the Diagonal Pattern: Return the ans vector containing the diagonal pattern of the matrix. 🔄
Code Explanation:
The matrixDiagonally function takes a 2D vector mat as input. 🔄
It initializes variables n, ans, k, r, c, and up. 🔄
It traverses the matrix diagonally, storing elements in ans based on the traversal direction. 🔄
It toggles the traversal direction after each diagonal traversal. 🔄
Finally, it returns the ans vector containing the diagonal pattern. 🔄
#geeksforgeeks #codinginterview #leetcode #like #comment #subscribe #support