filmov
tv
Python Tutorial v3.2.5 Lesson 14 - Nested Functions
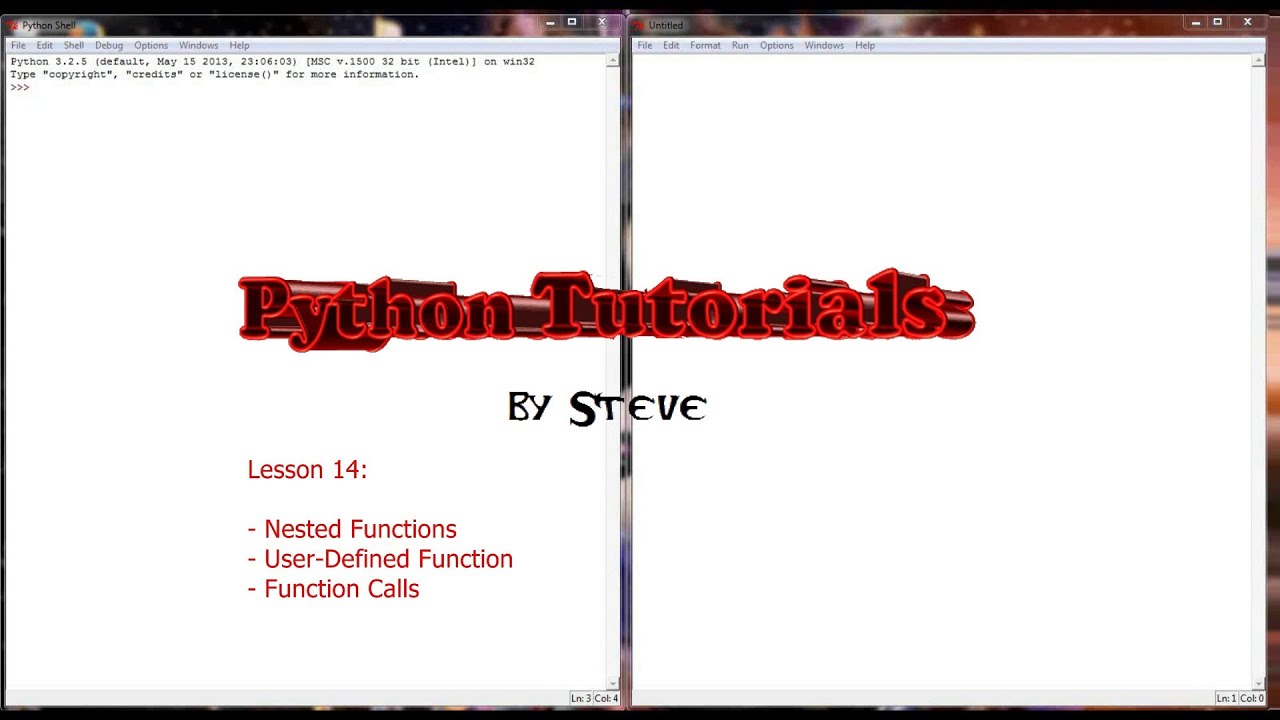
Показать описание
1:24 Click to skip the Introduction to Lesson 14.
10:58 Skip to an important note about using the Python Visualizer.
14:55 Skip directly to the Challenge Program for Lesson 14.
ThePython Visualizer website can be found here:
Lesson 14 discusses nested functions, which is simply calling a user defined function from within another function. The provided example consists of a program that converts temperatures from Fahrenheit to Celsius to Kelvin. The running of this program is then shown in the Python Visualizer from Lesson 13. Finally, a challenge program in which the programmer designs a help system and menu using nested functions is provided.
This is an introductory series of Python tutorials. This course, from start to finish, is designed to help someone who has never programmed before learn the basics of coding in Python. As this series continues, we examine more advanced Python techniques, functions, and methods.
Keep in mind this tutorial is using an older version of Python, v3.2.5. You will need to click on the "View Older Releases" button to use this specific version. Using the newer versions will not be an issue at this point, but when the lesson proceeds to basic graphics, the Pygame Module we'll use does not support v3.3+ at this time.
10:58 Skip to an important note about using the Python Visualizer.
14:55 Skip directly to the Challenge Program for Lesson 14.
ThePython Visualizer website can be found here:
Lesson 14 discusses nested functions, which is simply calling a user defined function from within another function. The provided example consists of a program that converts temperatures from Fahrenheit to Celsius to Kelvin. The running of this program is then shown in the Python Visualizer from Lesson 13. Finally, a challenge program in which the programmer designs a help system and menu using nested functions is provided.
This is an introductory series of Python tutorials. This course, from start to finish, is designed to help someone who has never programmed before learn the basics of coding in Python. As this series continues, we examine more advanced Python techniques, functions, and methods.
Keep in mind this tutorial is using an older version of Python, v3.2.5. You will need to click on the "View Older Releases" button to use this specific version. Using the newer versions will not be an issue at this point, but when the lesson proceeds to basic graphics, the Pygame Module we'll use does not support v3.3+ at this time.
Комментарии