filmov
tv
1979. Find Greatest Common Divisor of Array (Leetcode Easy)
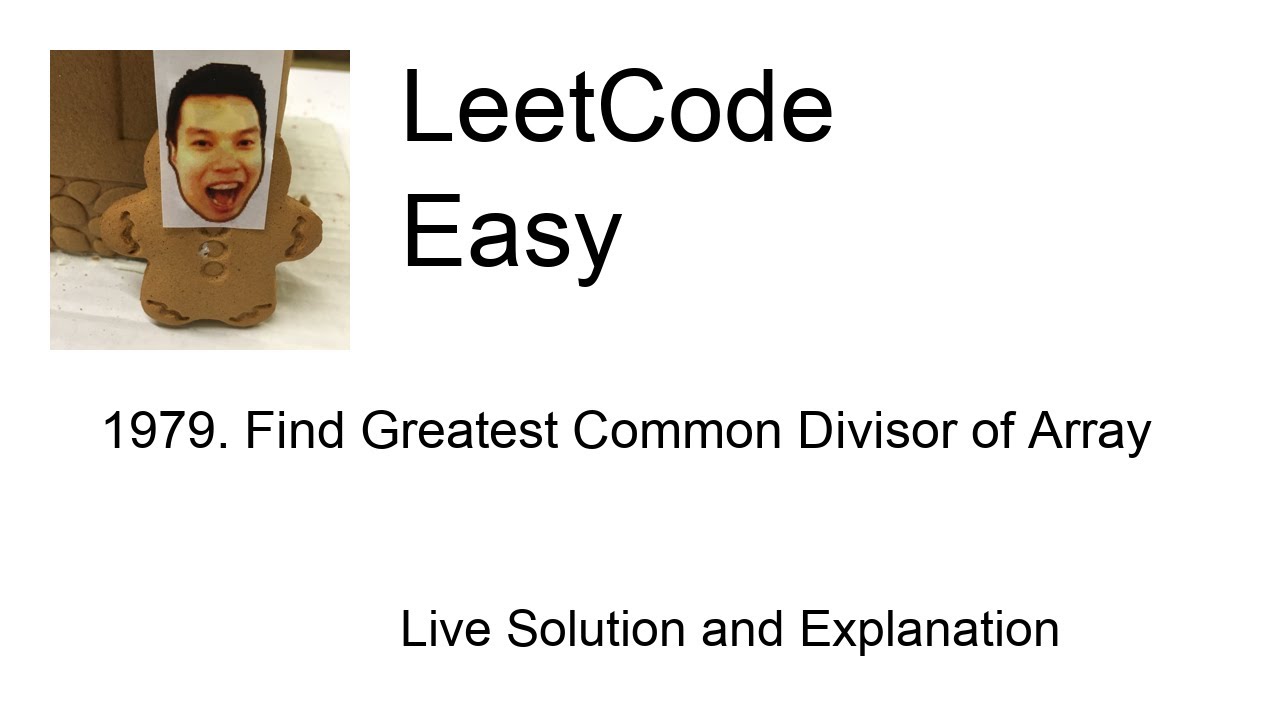
Показать описание
Larry solves and analyzes this Leetcode problem as both an interviewer and an interviewee. This is a live recording of a real engineer solving a problem live - no cuts or edits!
#leetcode #coding #programming
#leetcode #coding #programming
1979 Find Greatest Common Divisor of Array
1979. Find Greatest Common Divisor of Array | Leetcode | Arrays | Fundamentals
1979. Find Greatest Common Divisor of Array (Leetcode Easy)
Find Greatest Common Divisor of Array | LeetCode 1979 | English
leetcode 1979. Find Greatest Common Divisor of Array - elementary math
LeetCode 1979 | Find Greatest Common Divisor of Array | Array | GCD | Java
1979 ) Find Greatest common divisor of an Array | Leetcode | Array | Qn no:1979
Leetcode 1979. Find GCD with Euclid's algorithm
1979. Find Greatest Common Divisor of Array with JavaScript | Leetcode With JavaScript #youtube
Leetcode 1979. Find GCD | Euclid's algorithm #coding #dsa
1979. Find Greatest Common Divisor of Array with Python | Leetcode with Python #technology #leetcode
Intro to Java Chapter 05 Exercise 14 - Compute the Greatest Common Divisor
Greatest Common Divisor of Strings | Leetcode - 1071
1979. Find Greatest Common Divisor of Array - Javascript - Time: O(n) Space: O(1) - Leetcode
Program to find GCD 0f 2 numbers and GCD of N numbers or GCD of an Array
Find the Highest Common Factor (HCF) or Greatest Common Divisor (GCD) of an Array of Numbers in Java
Find Greatest Common Divisor of Array || #Leetcode Solution || link in the Description
JavaScript | find greatest common divisor - gcd #javascript
LeetCode Interview Questions - Greatest Common Divisor (Euclidian's Algorithm)
Python - Greatest Common Divisor W/ Recursion
LeetCode 1998. GCD Sort of an Array
1071. Greatest Common Divisor of Strings - Day 1/28 Leetcode February Challenge
Java program to find the greatest common divisor of given numbers
Javascript (Greatest Common Factor - Remove Duplicated Numbers) Algorithms
Комментарии