filmov
tv
Generate Parentheses - Stack - Leetcode 22
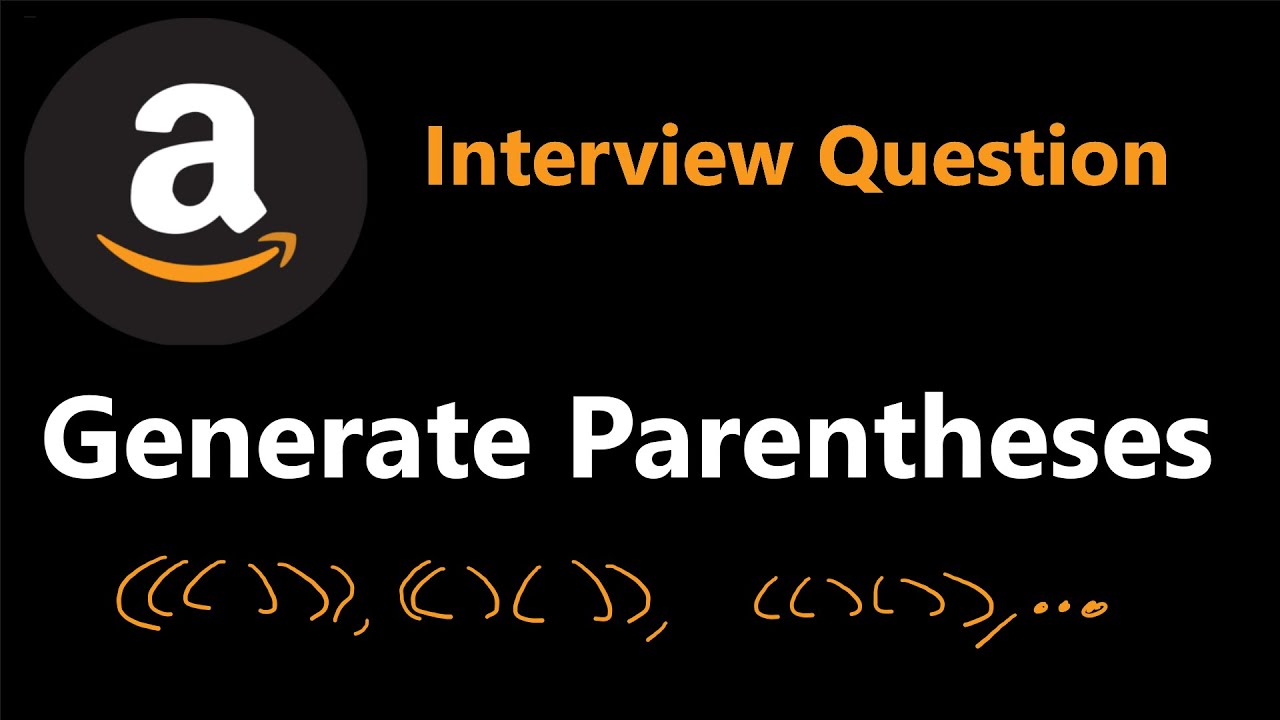
Показать описание
0:00 - Read the problem
1:30 - Drawing Explanation
9:55 - Coding Explanation
leetcode 22
#generate #parentheses #python
Disclosure: Some of the links above may be affiliate links, from which I may earn a small commission.
Generate Parentheses - Stack - Leetcode 22
Intel Coding Interview Question - Generate Parentheses - Leetcode 22
Leetcode 22. Generate Parentheses ||Code+ Explanation + Full recursion flow Walkthrough ||June Daily
Generate Parentheses - Leetcode 22 - Recursive Backtracking (Python)
LeetCode 22. Generate Parentheses
LeetCode 22 - Generate Parenthesis
LeetCode 22 Generate Parentheses with call stack runthrough
Generate Paranthesis 😍
Leetcode - Generate Parentheses (Python)
Valid Parentheses - Stack - Leetcode 20 - Python
Longest Valid Parentheses | Live Coding with Explanation | Leetcode - 32
Generate all Balanced Parentheses
Generate All Strings With n Matched Parentheses - Backtracking ('Generate Parentheses' on ...
Generate Parentheses | 2 Approaches | Magic Of Recursion | Recursion Concepts And Questions
Stacks are ESSENTIAL for Coding Interviews! | Valid Parentheses - Leetcode 20
Generate Parentheses | Leetcode 22 | Recursion
Faang Coding Question! | Generate Parentheses - Leetcode 22
Generate Parentheses: 22 - Dynamic Programming interview @ google, apple, amazon, meta, microsoft
LEETCODE 22 (JAVASCRIPT) | GENERATE PARENTHESES
Generate Parentheses - Stack # 4 - Python - Leetcode 22
Valid Parentheses - Leetcode 20
11. Generate Parantheses | The recursion question that I have asked the most in interviews! 🚀🚀
NeetCode150 - GENERATE PARENTHESES | Stack | Medium Level Question | LEETCODE | DSA in Python
Generate Parentheses - LeetCode 22 - Python #backtracking #recursion #leetcode
Комментарии