filmov
tv
How to Fix the TypeError in Your Python Pandas Script
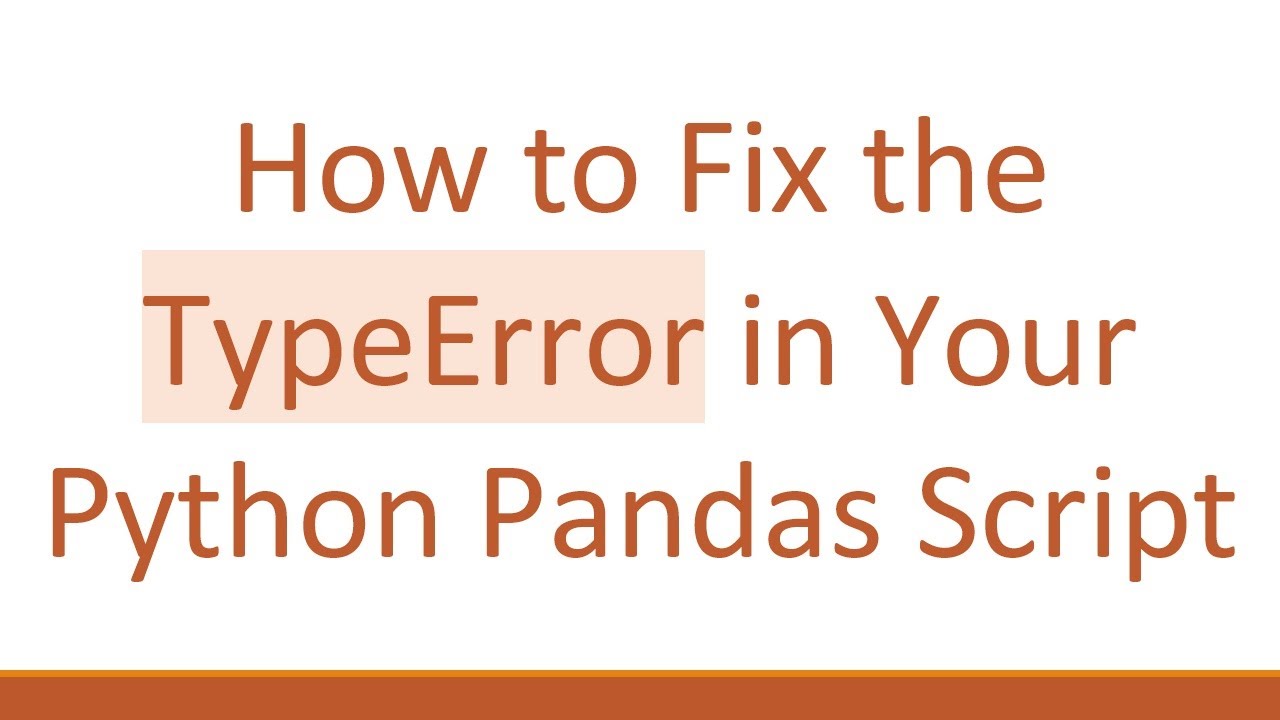
Показать описание
Learn how to solve the `TypeError: Expected file path name or file-like object` in your Python script using Pandas effectively.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: I am having this issue with a script that I wrote that is giving me an error as shown below. Please advise
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Encountering an Error in Your Python Script? Let's Dive into the Solution!
If you are learning Python and working with data processing using Pandas, encountering errors can be a bit frustrating. One common issue many beginners face is related to invalid object types being passed to functions. Recently, a user encountered a TypeError while trying to use Pandas to read a JSON object and save it as a CSV file. Let's break down this problem and explore how you can fix it.
Understanding the Problem
The user reported facing the following error:
[[See Video to Reveal this Text or Code Snippet]]
This type of error occurs when the read_json function from Pandas is provided with an unexpected type of argument. The function is designed to take either a file path or a file-like object, but it received a bytes string instead.
The Cause of the Error
Here’s a closer look at the relevant part of the code:
[[See Video to Reveal this Text or Code Snippet]]
In this line, the script attempts to convert the data (which is a Python object) to a JSON string and then encodes it to bytes. It tries to directly pass the bytes string to read_json, which leads to the TypeError.
Solution: Using io.BytesIO
To resolve this issue, you need to convert the bytes string into a bytes buffer that behaves like a file-like object, which can be done using the io.BytesIO class in Python. Here’s how you can modify your code:
Step-by-Step Fix
Import io: At the start of your script, ensure you have imported the io module.
[[See Video to Reveal this Text or Code Snippet]]
Modify the Problematic Line: Instead of passing the bytes string directly to read_json, wrap it in an io.BytesIO object.
Updated Code Example
Here’s how your updated line should look:
[[See Video to Reveal this Text or Code Snippet]]
Explanation of Changes
io.BytesIO(): This function creates an in-memory buffer that mimics a file object, allowing various file-like operations.
Encoding Handling: You still keep your encoding options intact, ensuring that the CSV is saved correctly.
Conclusion
With these changes in your script, the TypeError should be resolved. Error handling is an important part of coding, especially in Python, where data manipulation with libraries like Pandas is so prevalent.
If you're just starting out, remember that building robust scripts often involves testing and debugging. Don't hesitate to reach out for help, and keep experimenting! Coding is as much about persistence as it is about knowledge.
Happy coding!
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: I am having this issue with a script that I wrote that is giving me an error as shown below. Please advise
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Encountering an Error in Your Python Script? Let's Dive into the Solution!
If you are learning Python and working with data processing using Pandas, encountering errors can be a bit frustrating. One common issue many beginners face is related to invalid object types being passed to functions. Recently, a user encountered a TypeError while trying to use Pandas to read a JSON object and save it as a CSV file. Let's break down this problem and explore how you can fix it.
Understanding the Problem
The user reported facing the following error:
[[See Video to Reveal this Text or Code Snippet]]
This type of error occurs when the read_json function from Pandas is provided with an unexpected type of argument. The function is designed to take either a file path or a file-like object, but it received a bytes string instead.
The Cause of the Error
Here’s a closer look at the relevant part of the code:
[[See Video to Reveal this Text or Code Snippet]]
In this line, the script attempts to convert the data (which is a Python object) to a JSON string and then encodes it to bytes. It tries to directly pass the bytes string to read_json, which leads to the TypeError.
Solution: Using io.BytesIO
To resolve this issue, you need to convert the bytes string into a bytes buffer that behaves like a file-like object, which can be done using the io.BytesIO class in Python. Here’s how you can modify your code:
Step-by-Step Fix
Import io: At the start of your script, ensure you have imported the io module.
[[See Video to Reveal this Text or Code Snippet]]
Modify the Problematic Line: Instead of passing the bytes string directly to read_json, wrap it in an io.BytesIO object.
Updated Code Example
Here’s how your updated line should look:
[[See Video to Reveal this Text or Code Snippet]]
Explanation of Changes
io.BytesIO(): This function creates an in-memory buffer that mimics a file object, allowing various file-like operations.
Encoding Handling: You still keep your encoding options intact, ensuring that the CSV is saved correctly.
Conclusion
With these changes in your script, the TypeError should be resolved. Error handling is an important part of coding, especially in Python, where data manipulation with libraries like Pandas is so prevalent.
If you're just starting out, remember that building robust scripts often involves testing and debugging. Don't hesitate to reach out for help, and keep experimenting! Coding is as much about persistence as it is about knowledge.
Happy coding!