filmov
tv
Backtracking: Permutations - Leetcode 46 - Python
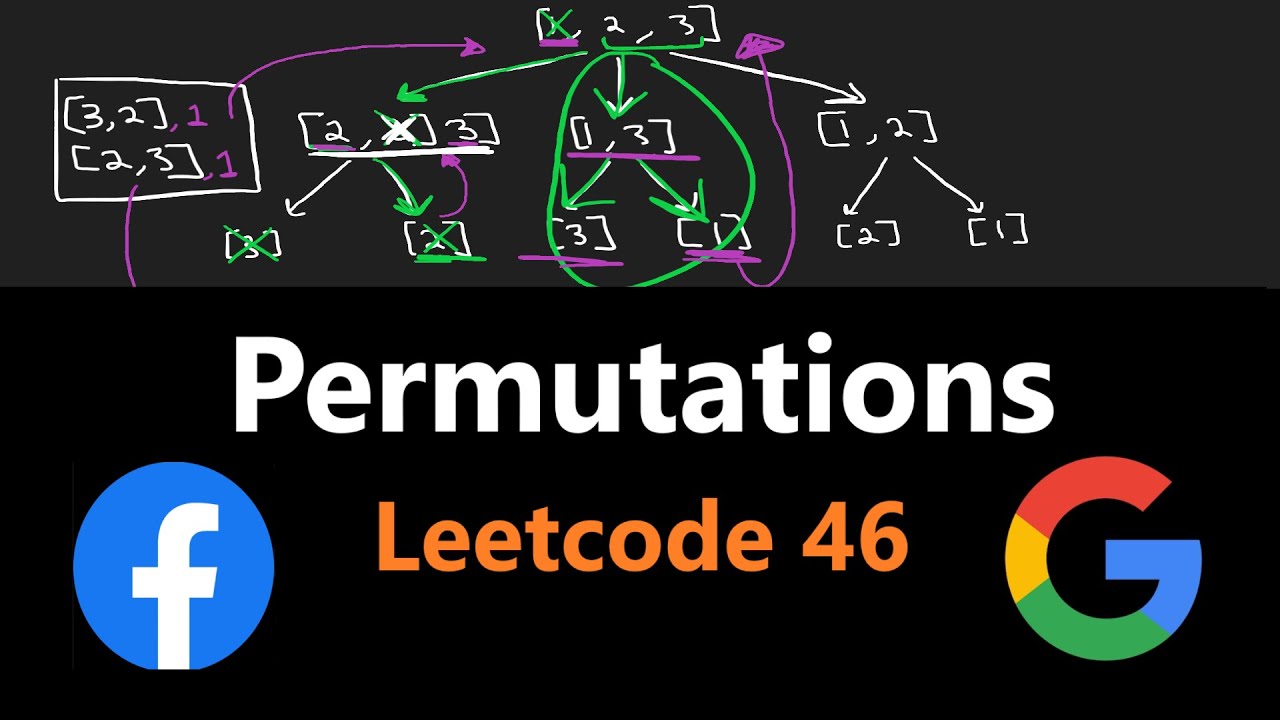
Показать описание
0:00 - Drawing explanation
6:12 - Coding solution
Leetcode 46
#Coding #leetcode #neetcode
Disclosure: Some of the links above may be affiliate links, from which I may earn a small commission.
Backtracking: Permutations - Leetcode 46 - Python
Leetcode 46. Permutations : Introduction to backtracking
Coding Interviews Need Backtracking! | Permutations - Leetcode 46
Google Backtracking Interview Question - Permutations - Leetcode 46
Permutations - Leetcode 46 - Python
Permutations (LeetCode 46) | Full solution with backtracking examples | Interview | Study Algorithms
Permutations - Leetcode 46 - Recursive Backtracking (Python)
PERMUTATIONS | LEETCODE 46 | PYTHON BACKTRACKING SOLUTION
Day 28:100 solving DSA problems | BACKTRACKING
Permutations Leetcode 46
Backtracking: Permutations - Leetcode 46 - Python
Permutations | LeetCode 46 | TopInterview | Google Facebook | Backtracking
[Java] Leetcode 46/47. Permutations I/II [Backtracking #4]
leetCode 46 Permutations(Back Tracking) | JSer - algorithm and JavaScript
Permutations - LeetCode Question 46 - JavaScript
Leetcode 46. Permutations. Backtracking. Python
Leetcode 46. Permutations | Backtrack | Logic Coding
Permutations - Leetcode 46 - Java
LeetCode 46 | Permutations | BackTrack | Java
LEETCODE 46 PERMUTATIONS PYTHON | Backtracking
Permutations | Live Coding with Explanation | Leetcode #46
Permutations - Leetcode 46 - JavaScript
46. Permutations | Backtracking / Recursion Based Solution
Permutations - leetcode 46 - Recursive
Комментарии