filmov
tv
How to Fix AttributeError in Python Classes
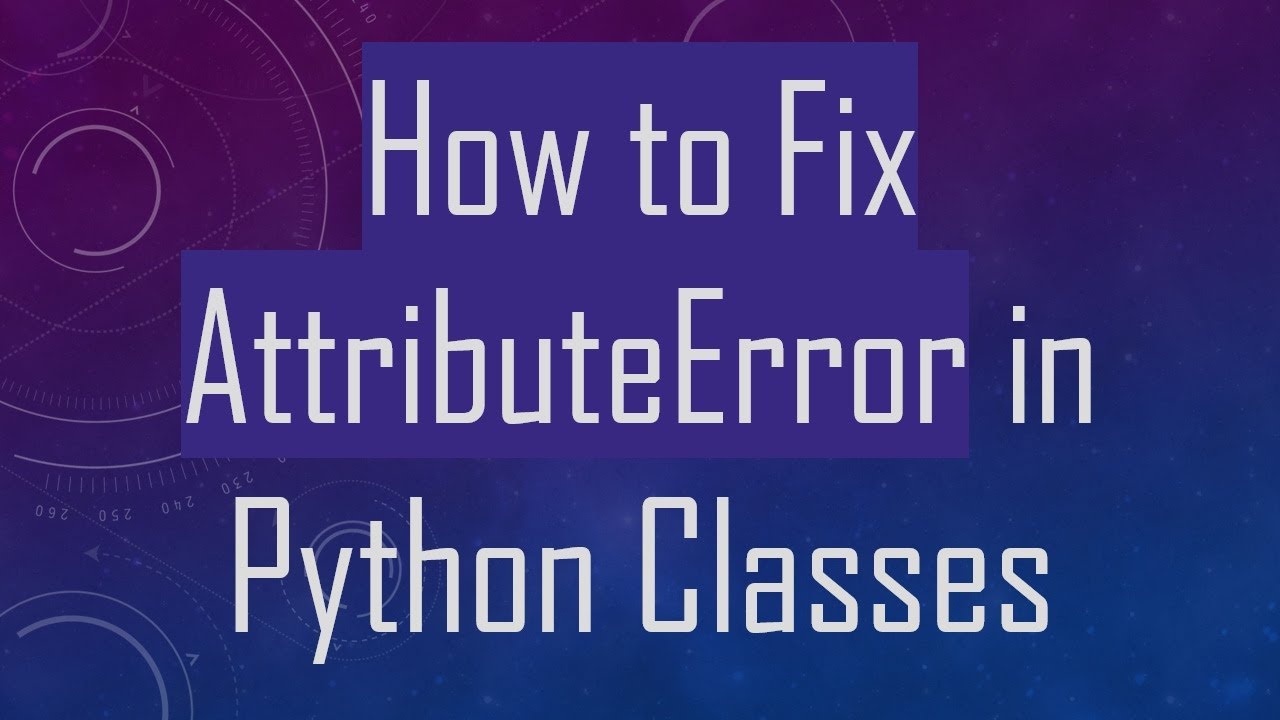
Показать описание
Learn how to resolve the `AttributeError` in Python when using class methods and instance attributes with our detailed guide.
---
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
How to Fix AttributeError in Python Classes: A Beginner's Guide
If you're learning Python, you might encounter various challenges. One common issue beginners face is the AttributeError, especially when working with classes and methods. In this guide, we'll address a specific AttributeError that arises when trying to use class methods to access instance attributes. Specifically, we’ll use a case involving the Spotify API to illustrate the solution.
The Problem Identified
Consider the following scenario: You're building a class to interact with the Spotify API and you encounter the error message:
[[See Video to Reveal this Text or Code Snippet]]
Understanding the Error
What Causes the Error?
The error derives from a misunderstanding of the difference between class methods and instance methods in Python:
Class Method: A method defined with @ classmethod decorator, bound to the class rather than an instance of the class. This means it doesn't have access to instance-specific data (attributes).
Instance Method: A traditional method (without the class decorator) that can access the instance (using self), allowing interaction with the attributes that belong to that specific instance.
The Solution
To resolve this issue, we need to convert the get_spapi method from a class method to an instance method. Here’s how to do that:
Step 1: Remove the @ classmethod Decorator
By removing the @ classmethod decorator, you declare get_spapi as an instance method that has access to self.
Here’s the updated method:
[[See Video to Reveal this Text or Code Snippet]]
Step 2: Use the Updated Method
You can then create your instance of the spapi class and call the method without any issues:
[[See Video to Reveal this Text or Code Snippet]]
Conclusion
The crucial takeaway from this lesson is understanding the distinction between class methods and instance methods in Python. Class methods cannot access instance attributes, which is why you encountered the AttributeError. By converting get_spapi from a class method to an instance method, you enable it to access the attributes stored in the instance.
Keep practicing with classes, and soon enough, these concepts will become second nature. Happy coding!
---
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
How to Fix AttributeError in Python Classes: A Beginner's Guide
If you're learning Python, you might encounter various challenges. One common issue beginners face is the AttributeError, especially when working with classes and methods. In this guide, we'll address a specific AttributeError that arises when trying to use class methods to access instance attributes. Specifically, we’ll use a case involving the Spotify API to illustrate the solution.
The Problem Identified
Consider the following scenario: You're building a class to interact with the Spotify API and you encounter the error message:
[[See Video to Reveal this Text or Code Snippet]]
Understanding the Error
What Causes the Error?
The error derives from a misunderstanding of the difference between class methods and instance methods in Python:
Class Method: A method defined with @ classmethod decorator, bound to the class rather than an instance of the class. This means it doesn't have access to instance-specific data (attributes).
Instance Method: A traditional method (without the class decorator) that can access the instance (using self), allowing interaction with the attributes that belong to that specific instance.
The Solution
To resolve this issue, we need to convert the get_spapi method from a class method to an instance method. Here’s how to do that:
Step 1: Remove the @ classmethod Decorator
By removing the @ classmethod decorator, you declare get_spapi as an instance method that has access to self.
Here’s the updated method:
[[See Video to Reveal this Text or Code Snippet]]
Step 2: Use the Updated Method
You can then create your instance of the spapi class and call the method without any issues:
[[See Video to Reveal this Text or Code Snippet]]
Conclusion
The crucial takeaway from this lesson is understanding the distinction between class methods and instance methods in Python. Class methods cannot access instance attributes, which is why you encountered the AttributeError. By converting get_spapi from a class method to an instance method, you enable it to access the attributes stored in the instance.
Keep practicing with classes, and soon enough, these concepts will become second nature. Happy coding!