filmov
tv
Grouping Objects in JavaScript: How to Combine Array Data by Property
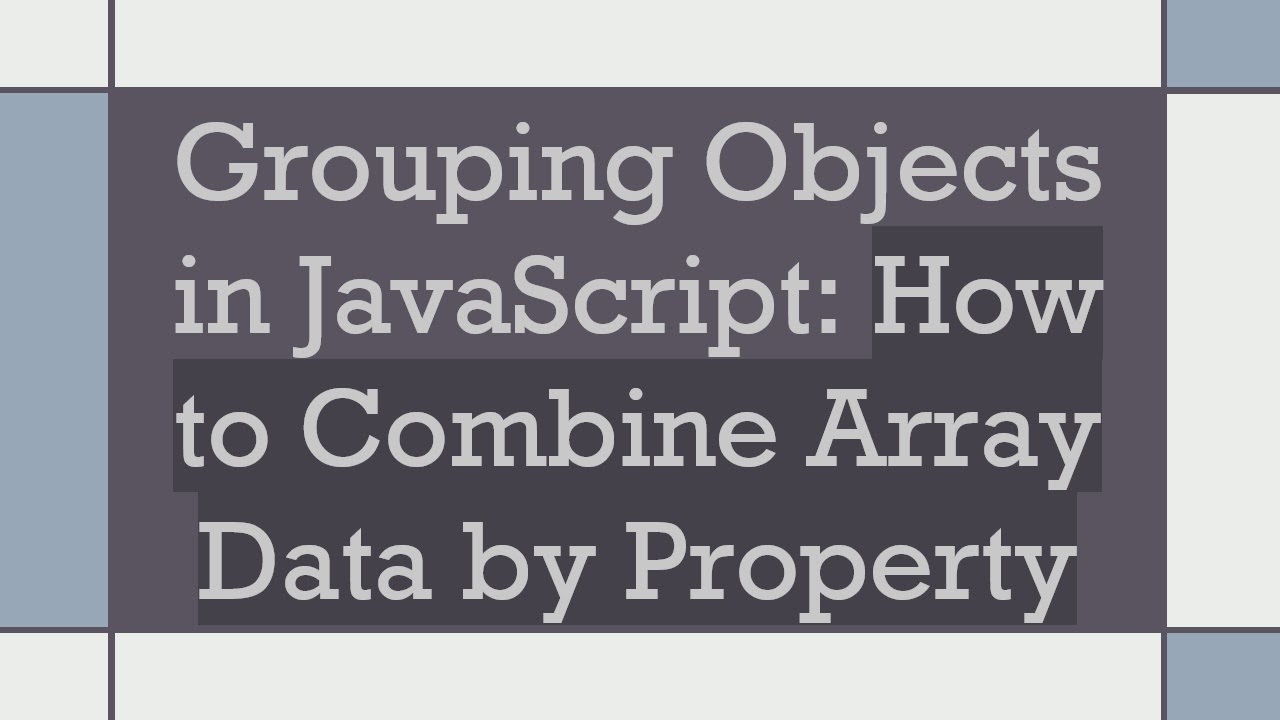
Показать описание
Learn how to easily group and squash an array of objects by property in JavaScript for cleaner data management.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Array of objects - how to group items by property and squash data from each of grouped items into 1
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Grouping Objects in JavaScript: How to Combine Array Data by Property
Handling arrays of objects is a common task in JavaScript programming. However, manipulating this data structure can often be more complicated than expected, especially when it comes to grouping and restructuring the data based on specific properties. If you’ve ever found yourself wondering how to group items by a property and then combine data from those grouped items into one, you are definitely not alone! In this guide, we’ll delve into this tricky aspect of JavaScript arrays and outline a clean solution.
The Problem: Grouping and Squashing Data
Consider an array of objects structured like this:
[[See Video to Reveal this Text or Code Snippet]]
The challenge here is to group this data by the property y and squash the data label values from each of the grouped items. The desired output format should look like this:
[[See Video to Reveal this Text or Code Snippet]]
Solution: Using reduce and map
To achieve this, we can utilize the reduce and map array functions in JavaScript. Here’s a step-by-step breakdown of how we can implement this:
Step 1: Initialize the Restructure Function
Create a function called restructureArr that will accept the array to be restructured:
[[See Video to Reveal this Text or Code Snippet]]
Step 2: Breaking Down the Functionality
Input Parameter: The function takes arrToRestructure, which is the array of objects we want to manipulate.
Reduce Function: The reduce method is called on arrToRestructure:
Accumulator: acc (accumulated result) will collect the values.
Current Values: Each object’s property y determines the key for our final output.
Mapping Data: We extract the label from the data array using map, ensuring to spread the results into the accumulator for squashing.
Step 3: Putting It All Together
Finally, let’s test the function with our original data:
[[See Video to Reveal this Text or Code Snippet]]
By running this code, you should see the desired output that combines all label values under their corresponding properties.
Conclusion
Grouping objects and squashing their data in JavaScript does not have to be daunting. With the use of reduce and map, you can efficiently aggregate this information into a cleaner, more manageable structure. By following the steps outlined in this post, you can apply the same principles to your own arrays of objects to streamline your data handling.
Now that you know how to work with arrays of objects, you can explore more complex data transformations and harness the full power of JavaScript!
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Array of objects - how to group items by property and squash data from each of grouped items into 1
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Grouping Objects in JavaScript: How to Combine Array Data by Property
Handling arrays of objects is a common task in JavaScript programming. However, manipulating this data structure can often be more complicated than expected, especially when it comes to grouping and restructuring the data based on specific properties. If you’ve ever found yourself wondering how to group items by a property and then combine data from those grouped items into one, you are definitely not alone! In this guide, we’ll delve into this tricky aspect of JavaScript arrays and outline a clean solution.
The Problem: Grouping and Squashing Data
Consider an array of objects structured like this:
[[See Video to Reveal this Text or Code Snippet]]
The challenge here is to group this data by the property y and squash the data label values from each of the grouped items. The desired output format should look like this:
[[See Video to Reveal this Text or Code Snippet]]
Solution: Using reduce and map
To achieve this, we can utilize the reduce and map array functions in JavaScript. Here’s a step-by-step breakdown of how we can implement this:
Step 1: Initialize the Restructure Function
Create a function called restructureArr that will accept the array to be restructured:
[[See Video to Reveal this Text or Code Snippet]]
Step 2: Breaking Down the Functionality
Input Parameter: The function takes arrToRestructure, which is the array of objects we want to manipulate.
Reduce Function: The reduce method is called on arrToRestructure:
Accumulator: acc (accumulated result) will collect the values.
Current Values: Each object’s property y determines the key for our final output.
Mapping Data: We extract the label from the data array using map, ensuring to spread the results into the accumulator for squashing.
Step 3: Putting It All Together
Finally, let’s test the function with our original data:
[[See Video to Reveal this Text or Code Snippet]]
By running this code, you should see the desired output that combines all label values under their corresponding properties.
Conclusion
Grouping objects and squashing their data in JavaScript does not have to be daunting. With the use of reduce and map, you can efficiently aggregate this information into a cleaner, more manageable structure. By following the steps outlined in this post, you can apply the same principles to your own arrays of objects to streamline your data handling.
Now that you know how to work with arrays of objects, you can explore more complex data transformations and harness the full power of JavaScript!