filmov
tv
contiguous array leetcode 525 python
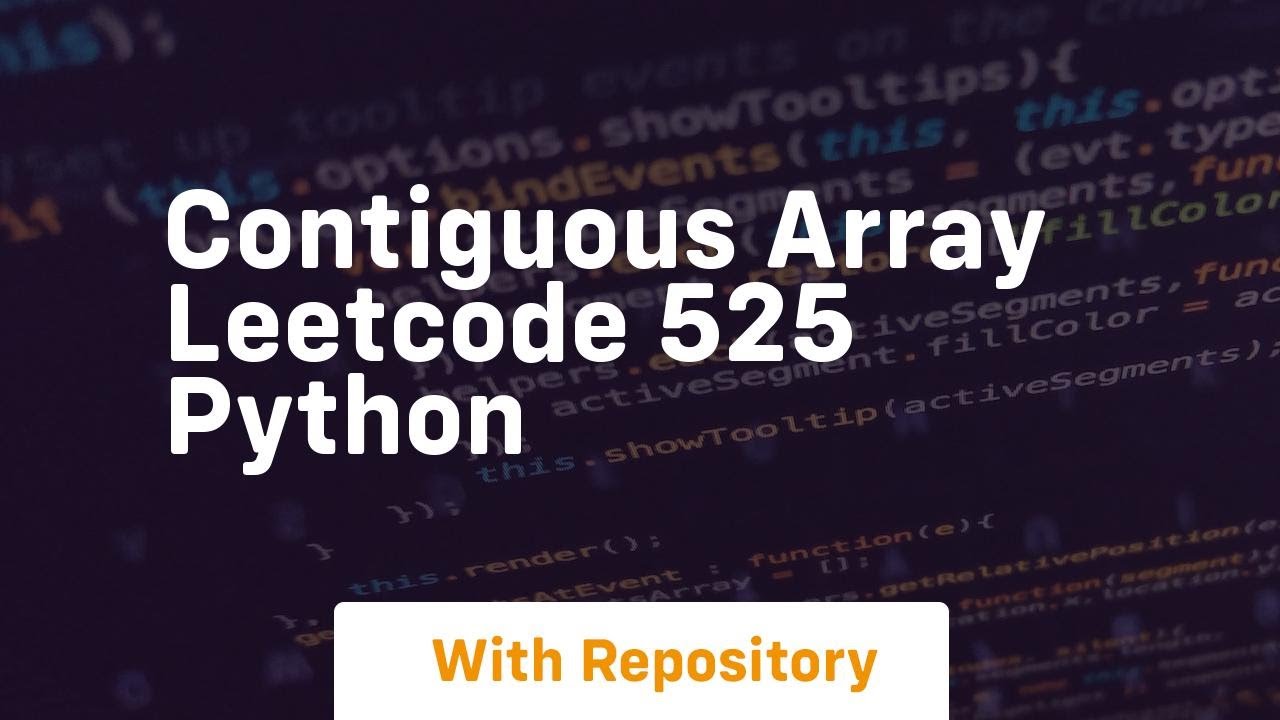
Показать описание
certainly! the "contiguous array" problem on leetcode (problem 525) is a classic problem that involves finding the largest contiguous subarray where the number of 0s is equal to the number of 1s. let's break down the problem, the approach, and provide a code example in python.
problem statement
given a binary array `nums`, you need to find the maximum length of a contiguous subarray that contains an equal number of 0s and 1s.
example
approach
1. **transform the array**: convert all 0s in the array to -1s. this way, the problem reduces to finding the largest subarray with a sum of zero.
- for example, `[0, 1, 0]` becomes `[-1, 1, -1]`.
2. **use a hashmap**: maintain a hashmap (or dictionary) to store the cumulative sum at each index and its corresponding first occurrence index.
- if the same cumulative sum occurs again at a later index, it means that the subarray between those two indices has a sum of zero.
3. **iterate through the array**: keep track of the cumulative sum. for each element:
- if the cumulative sum is zero, update the maximum length.
- if the cumulative sum has been seen before, calculate the length of the subarray and update the maximum length if this length is greater.
code example
here’s how you can implement this in python:
explanation of the code
- we initialize a dictionary `sum_index_map` with the key `0` mapped to `-1` to handle cases where a subarray starting from index `0` sums to zero.
- we then iterate through the `nums` array while maintaining a `cumulative_sum`.
- for each element, we update the `cumulative_sum` accordingly.
- if the `cumulative_sum` has been encountered before, we calculate the length of the subarray and compare it with the maximum length found so far.
- if it hasn't been seen, we store the index of this `cumulative_sum` in the dictionary.
complexity analysis
- **time complexity**: o(n), where n is the length of the input array. we traverse the array once.
- **space complexity**: o(n) in th ...
#ContiguousArray #LeetCode #windows
contiguous array
leetcode
problem 525
python
subarray
binary array
maximum length
prefix sum
hashmap
zero sum
count occurrences
algorithm
sliding window
optimization
time complexity
problem statement
given a binary array `nums`, you need to find the maximum length of a contiguous subarray that contains an equal number of 0s and 1s.
example
approach
1. **transform the array**: convert all 0s in the array to -1s. this way, the problem reduces to finding the largest subarray with a sum of zero.
- for example, `[0, 1, 0]` becomes `[-1, 1, -1]`.
2. **use a hashmap**: maintain a hashmap (or dictionary) to store the cumulative sum at each index and its corresponding first occurrence index.
- if the same cumulative sum occurs again at a later index, it means that the subarray between those two indices has a sum of zero.
3. **iterate through the array**: keep track of the cumulative sum. for each element:
- if the cumulative sum is zero, update the maximum length.
- if the cumulative sum has been seen before, calculate the length of the subarray and update the maximum length if this length is greater.
code example
here’s how you can implement this in python:
explanation of the code
- we initialize a dictionary `sum_index_map` with the key `0` mapped to `-1` to handle cases where a subarray starting from index `0` sums to zero.
- we then iterate through the `nums` array while maintaining a `cumulative_sum`.
- for each element, we update the `cumulative_sum` accordingly.
- if the `cumulative_sum` has been encountered before, we calculate the length of the subarray and compare it with the maximum length found so far.
- if it hasn't been seen, we store the index of this `cumulative_sum` in the dictionary.
complexity analysis
- **time complexity**: o(n), where n is the length of the input array. we traverse the array once.
- **space complexity**: o(n) in th ...
#ContiguousArray #LeetCode #windows
contiguous array
leetcode
problem 525
python
subarray
binary array
maximum length
prefix sum
hashmap
zero sum
count occurrences
algorithm
sliding window
optimization
time complexity