filmov
tv
Java with BlueJ in Hindi Class X part 1
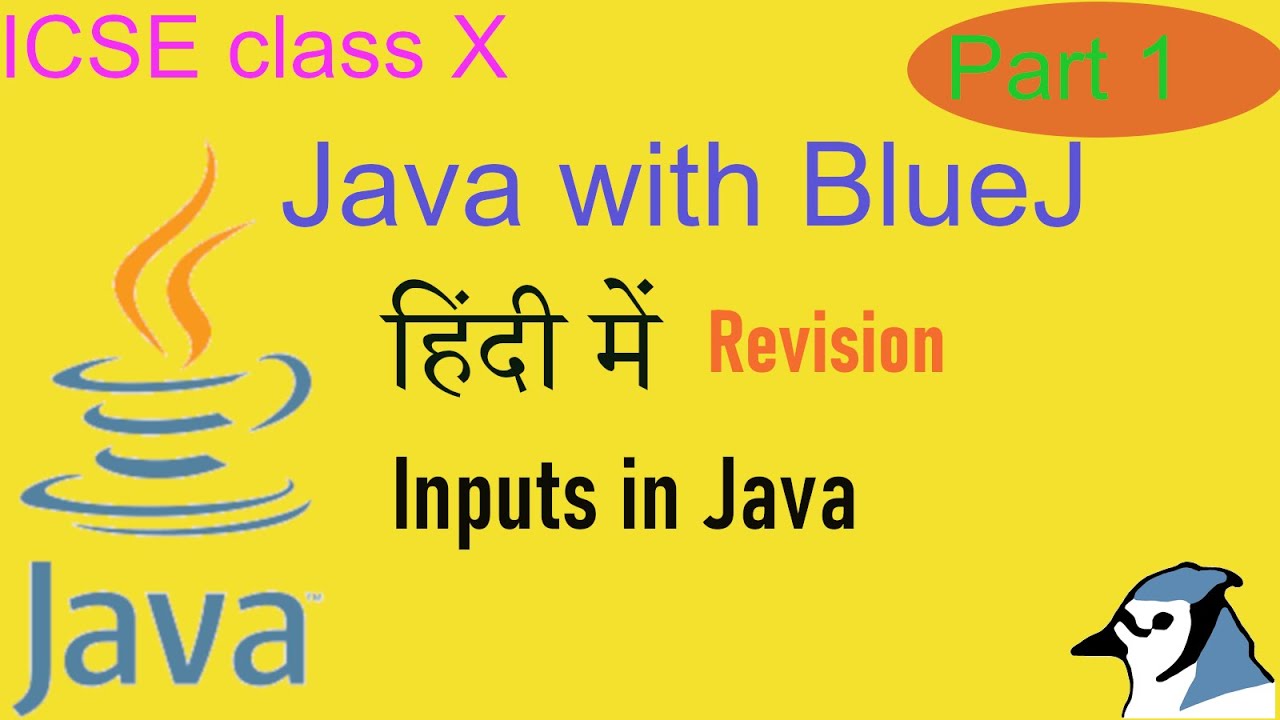
Показать описание
Learn Java with BlueJ IDE for Class 10th - Part 1
Syllabus according to Class X of ICSE
Revision
Syllabus according to Class X of ICSE
Revision
Java with BlueJ in Hindi - Part 1
Learn Java Programming through Bluej in Hindi #programming #bluej
How to download and install BlueJ in Windows | Java
My first Java program using Blue-J part-1 (hindi)
Java with BlueJ in Hindi Class X part 1
How to Write A Simple Java Program Using BlueJ in Hindi | Java Program
Java with BlueJ in Hindi - Ch 6
java programming with bluej interface (HINDI)
Introduction to Java (Hindi) | What is Java? Explain with Syntax and Example
Java with BlueJ in Hindi - Ch 9
Run Your First JAVA Program in BlueJ || in Hindi
How to run Java program on BlueJ (in hindi)|| Java full Tutorial||
Java with BlueJ in Hindi - Ch 5 full
Java with BlueJ in Hindi - Part 22
Java with BlueJ in Hindi Class X Chapter 3 part 1
Java with BlueJ in Hindi - Part 4
Java with BlueJ in Hindi - Part 2
Java with BlueJ in Hindi - Part 30
Java with BlueJ in Hindi - Part 21
Java with BlueJ in Hindi - Part 18
How to Install BlueJ on Windows - In Hindi
Java with BlueJ in Hindi - Part 35
Java with BlueJ in Hindi - Ch 7
Java Programming : Installing BlueJ on Windows Part 1 (Hindi)
Комментарии