filmov
tv
How to Properly Decode Base64 String to Image in Java
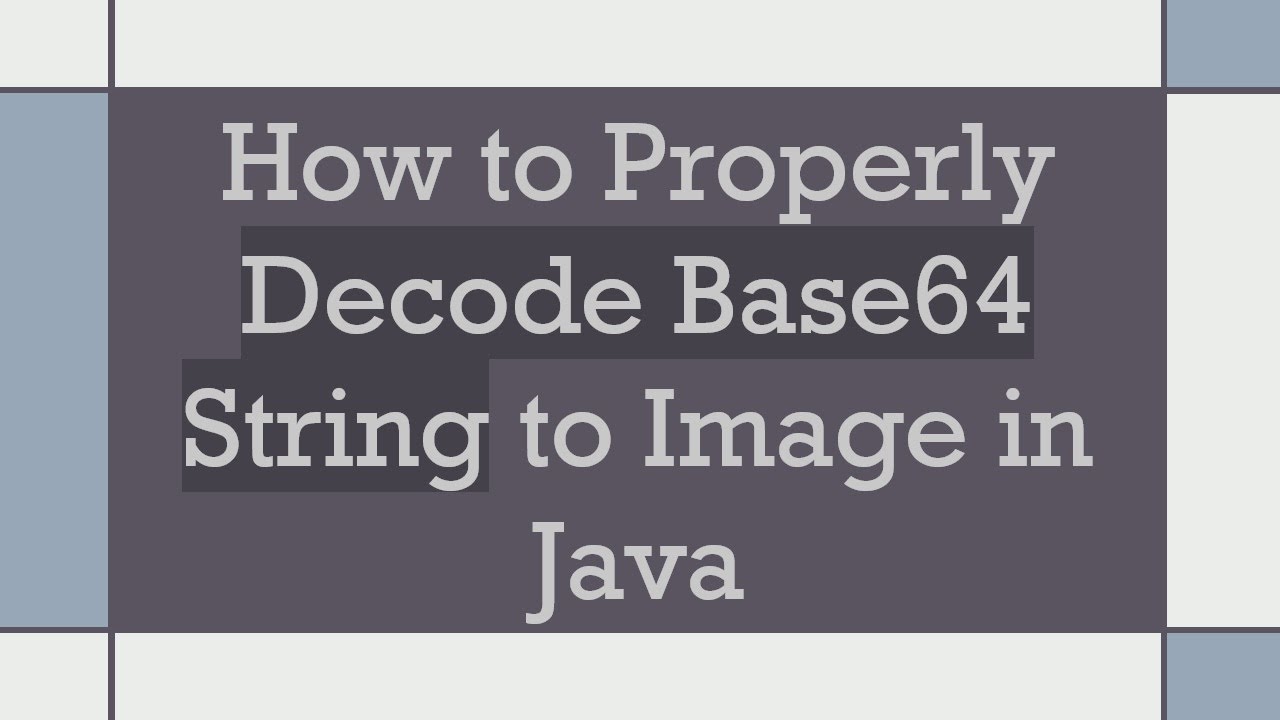
Показать описание
Learn how to decode a Base64 string to an image in Java, essential for handling graphics in Java applications, including Android development.
---
How to Properly Decode Base64 String to Image in Java?
In today's digital era, working with images programmatically is a common necessity. One such use case is decoding a Base64 encoded string into an image file. Base64 encoding is widely used for data transfer when binary data needs to be embedded in text formats such as JSON, XML, or HTML. This guide covers step-by-step how to decode a Base64 string to an image using Java, which is a critical skill for developers working on graphics-rich applications, including those on the Android platform.
What is Base64 Encoding?
Base64 encoding is a method of converting binary data into ASCII text. This technique is used to represent data in a manner that can be easily transmitted over text-based formats, such as HTTP or emails. However, when you receive Base64 encoded data, it often needs to be decoded back to its original binary form for use.
Steps to Decode Base64 String to Image in Java
The following steps will guide you through the process of decoding a Base64 string, converting it back into an image file using Java:
Import Necessary Libraries:
Prepare the Base64 String:
Typically, the Base64 string will be in a well-defined format, often including a prefix such as data:image/png;base64, which you will need to strip before conversion.
Decoding the Base64 String:
Use the Base64.Decoder class to decode the string into a byte array.
Create an Image File from Byte Array:
Write the byte array into a file to generate the image.
Here's a simple code example illustrating these steps:
[[See Video to Reveal this Text or Code Snippet]]
Important Points to Note
Handling Different Image Formats: Ensure that you consider the image format when decoding and saving. The file extension (.png, .jpg, etc.) should match the actual content of the Base64 string.
Error Handling: Always implement proper error handling. The decoding process can throw exceptions if the Base64 string is improperly formatted or the file operations fail.
Security Considerations: Base64 strings may come from untrusted sources, so always validate and sanitize the input to prevent security vulnerabilities.
This process is essential for applications that need to handle and display images received in Base64 format, which is common in web applications and APIs, including Android development.
By following this guide, you will be equipped to efficiently decode Base64 strings to images in your Java applications, facilitating seamless graphics rendering and processing.
---
How to Properly Decode Base64 String to Image in Java?
In today's digital era, working with images programmatically is a common necessity. One such use case is decoding a Base64 encoded string into an image file. Base64 encoding is widely used for data transfer when binary data needs to be embedded in text formats such as JSON, XML, or HTML. This guide covers step-by-step how to decode a Base64 string to an image using Java, which is a critical skill for developers working on graphics-rich applications, including those on the Android platform.
What is Base64 Encoding?
Base64 encoding is a method of converting binary data into ASCII text. This technique is used to represent data in a manner that can be easily transmitted over text-based formats, such as HTTP or emails. However, when you receive Base64 encoded data, it often needs to be decoded back to its original binary form for use.
Steps to Decode Base64 String to Image in Java
The following steps will guide you through the process of decoding a Base64 string, converting it back into an image file using Java:
Import Necessary Libraries:
Prepare the Base64 String:
Typically, the Base64 string will be in a well-defined format, often including a prefix such as data:image/png;base64, which you will need to strip before conversion.
Decoding the Base64 String:
Use the Base64.Decoder class to decode the string into a byte array.
Create an Image File from Byte Array:
Write the byte array into a file to generate the image.
Here's a simple code example illustrating these steps:
[[See Video to Reveal this Text or Code Snippet]]
Important Points to Note
Handling Different Image Formats: Ensure that you consider the image format when decoding and saving. The file extension (.png, .jpg, etc.) should match the actual content of the Base64 string.
Error Handling: Always implement proper error handling. The decoding process can throw exceptions if the Base64 string is improperly formatted or the file operations fail.
Security Considerations: Base64 strings may come from untrusted sources, so always validate and sanitize the input to prevent security vulnerabilities.
This process is essential for applications that need to handle and display images received in Base64 format, which is common in web applications and APIs, including Android development.
By following this guide, you will be equipped to efficiently decode Base64 strings to images in your Java applications, facilitating seamless graphics rendering and processing.