filmov
tv
String Buffer methods in Java
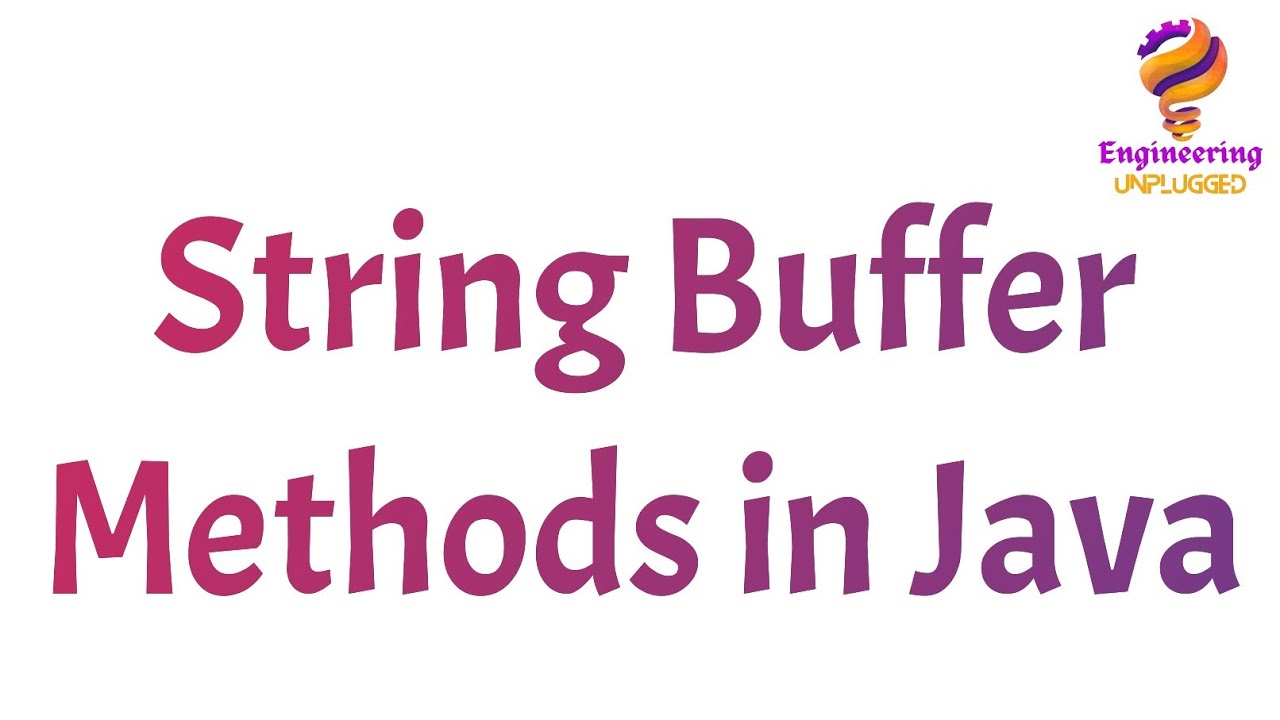
Показать описание
The `StringBuffer` class in Java is used to create mutable (modifiable) strings. Here are some commonly used methods with examples:
1. append(String str)
Adds the specified string to the end of the buffer.
Example:
StringBuffer sb = new StringBuffer("Hello");
2. insert(int offset, String str)
Inserts the string at the specified position.
Example:
StringBuffer sb = new StringBuffer("Hello");
3. replace(int start, int end, String str)
Description: Replaces characters from `start` to `end` with the specified string.
Example:
StringBuffer sb = new StringBuffer("Hello World");
4. delete(int start, int end)
Removes characters from `start` to `end`.
Example:
StringBuffer sb = new StringBuffer("Hello World");
5. reverse()
Reverses the characters in the buffer.
Example:
StringBuffer sb = new StringBuffer("Hello");
6. capacity()
Returns the current capacity of the buffer.
Example:
StringBuffer sb = new StringBuffer();
These methods demonstrate how `StringBuffer` can be used for efficient string manipulation.
1. append(String str)
Adds the specified string to the end of the buffer.
Example:
StringBuffer sb = new StringBuffer("Hello");
2. insert(int offset, String str)
Inserts the string at the specified position.
Example:
StringBuffer sb = new StringBuffer("Hello");
3. replace(int start, int end, String str)
Description: Replaces characters from `start` to `end` with the specified string.
Example:
StringBuffer sb = new StringBuffer("Hello World");
4. delete(int start, int end)
Removes characters from `start` to `end`.
Example:
StringBuffer sb = new StringBuffer("Hello World");
5. reverse()
Reverses the characters in the buffer.
Example:
StringBuffer sb = new StringBuffer("Hello");
6. capacity()
Returns the current capacity of the buffer.
Example:
StringBuffer sb = new StringBuffer();
These methods demonstrate how `StringBuffer` can be used for efficient string manipulation.