filmov
tv
How to Use LINQ to Select and Bind Data to a Model Class in VB.NET
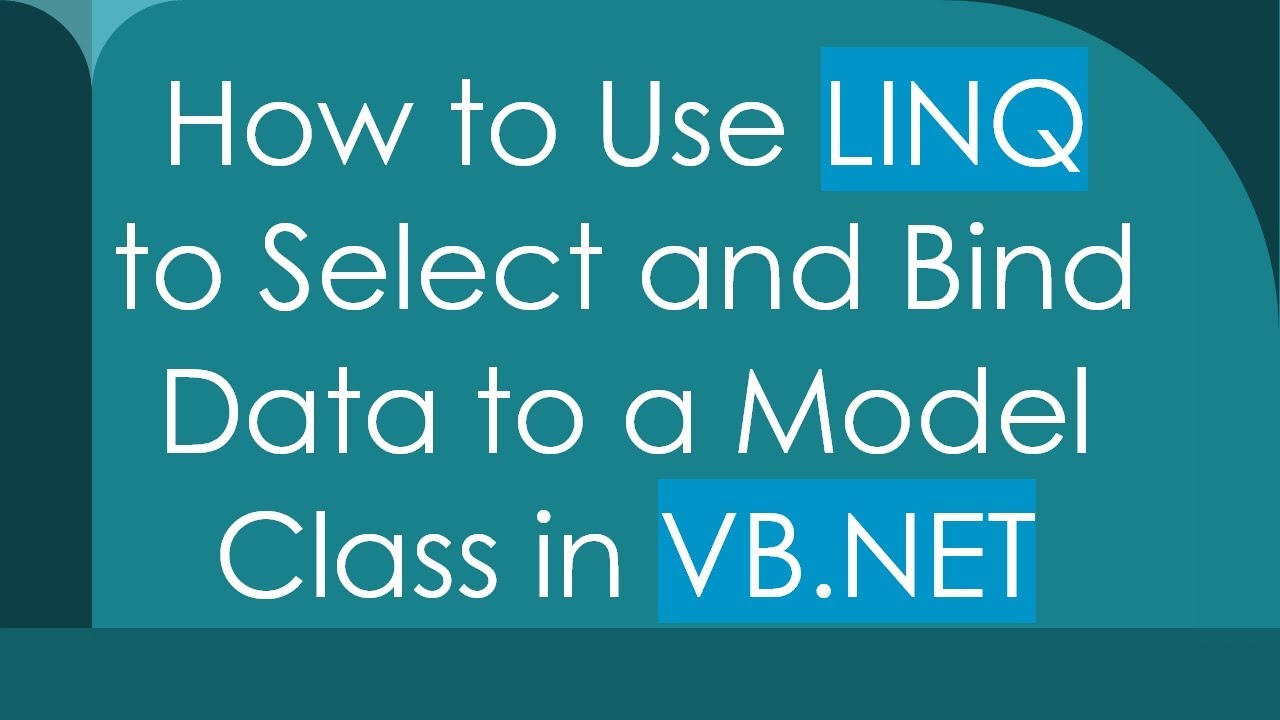
Показать описание
Learn how to effectively use LINQ in VB.NET to select and bind XML data to your model class, step-by-step.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: How to Linq select Model class Bind data in VB.net
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
How to Use LINQ to Select and Bind Data to a Model Class in VB.NET
If you're working on a VB.NET project and need to handle XML data, you might find yourself asking: How do I effectively use LINQ to select and bind this information to a model class? This common scenario can be a bit tricky if you're not familiar with the syntax or the process involved. In this guide, we'll break down the necessary steps to achieve this, ensuring that you can utilize LINQ effectively to manage XML data.
Understanding the Problem
When dealing with XML data in VB.NET, the goal is often to extract specific information and bind it to a model class. A model class is essentially a representation of the data structure you want to work with in your code. In this case, you might have an XML structure that looks something like this:
[[See Video to Reveal this Text or Code Snippet]]
Here, each <Item> element has attributes Name and Value. Our task is to select these attributes and bind them to an instance of a class, XMLClassName, which will have properties named FieldName and ValueName.
The Solution
Step 1: Define Your Model Class
First, you’ll need to create a model class that represents the data structure you want to bind to. In this case, the class might look like this:
[[See Video to Reveal this Text or Code Snippet]]
Step 2: Query the XML with LINQ
Once you have your model class defined, you can start querying the XML data using LINQ. In VB.NET, this is done using the Select method along with the Function syntax to create new instances of your model class.
Here’s how you can do this:
[[See Video to Reveal this Text or Code Snippet]]
Let’s break down this line of code:
xd.Descendants().Elements(): This part gets all the descendant elements under a specified XML node. In this case, it retrieves all <Item> elements within the XML.
Select(Function(n): This is where the selection begins. The Function(n) creates a new instance of XMLClassName for each item found.
New XMLClassName With {...}: This part initializes a new instance of the XMLClassName and assigns values to its properties using the attributes from the XML.
ToArray(): Finally, this converts the result of the selection into an array of XMLClassName objects.
Step 3: Use the Data
Now that you have an array of XMLClassName objects populated with data from the XML file, you can use it throughout your application. You might loop through the array to display the values, bind it to a UI component, or perform any other operations as needed.
Conclusion
In summary, using LINQ to select and bind XML data to a model class in VB.NET is a straightforward process once you break it down into manageable steps. By defining your model, performing a LINQ query, and utilizing the resulting data, you can effectively manage XML data in your applications. With these foundational skills, you can tackle any XML data manipulation tasks with confidence!
Now that you know the steps, give it a try in your own project! You'll discover how powerful and efficient working with data can be when using LINQ in VB.NET.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: How to Linq select Model class Bind data in VB.net
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
How to Use LINQ to Select and Bind Data to a Model Class in VB.NET
If you're working on a VB.NET project and need to handle XML data, you might find yourself asking: How do I effectively use LINQ to select and bind this information to a model class? This common scenario can be a bit tricky if you're not familiar with the syntax or the process involved. In this guide, we'll break down the necessary steps to achieve this, ensuring that you can utilize LINQ effectively to manage XML data.
Understanding the Problem
When dealing with XML data in VB.NET, the goal is often to extract specific information and bind it to a model class. A model class is essentially a representation of the data structure you want to work with in your code. In this case, you might have an XML structure that looks something like this:
[[See Video to Reveal this Text or Code Snippet]]
Here, each <Item> element has attributes Name and Value. Our task is to select these attributes and bind them to an instance of a class, XMLClassName, which will have properties named FieldName and ValueName.
The Solution
Step 1: Define Your Model Class
First, you’ll need to create a model class that represents the data structure you want to bind to. In this case, the class might look like this:
[[See Video to Reveal this Text or Code Snippet]]
Step 2: Query the XML with LINQ
Once you have your model class defined, you can start querying the XML data using LINQ. In VB.NET, this is done using the Select method along with the Function syntax to create new instances of your model class.
Here’s how you can do this:
[[See Video to Reveal this Text or Code Snippet]]
Let’s break down this line of code:
xd.Descendants().Elements(): This part gets all the descendant elements under a specified XML node. In this case, it retrieves all <Item> elements within the XML.
Select(Function(n): This is where the selection begins. The Function(n) creates a new instance of XMLClassName for each item found.
New XMLClassName With {...}: This part initializes a new instance of the XMLClassName and assigns values to its properties using the attributes from the XML.
ToArray(): Finally, this converts the result of the selection into an array of XMLClassName objects.
Step 3: Use the Data
Now that you have an array of XMLClassName objects populated with data from the XML file, you can use it throughout your application. You might loop through the array to display the values, bind it to a UI component, or perform any other operations as needed.
Conclusion
In summary, using LINQ to select and bind XML data to a model class in VB.NET is a straightforward process once you break it down into manageable steps. By defining your model, performing a LINQ query, and utilizing the resulting data, you can effectively manage XML data in your applications. With these foundational skills, you can tackle any XML data manipulation tasks with confidence!
Now that you know the steps, give it a try in your own project! You'll discover how powerful and efficient working with data can be when using LINQ in VB.NET.