filmov
tv
Python Turtle Graphics Tutorial for Absolute Beginners - Drawing a Spirographic
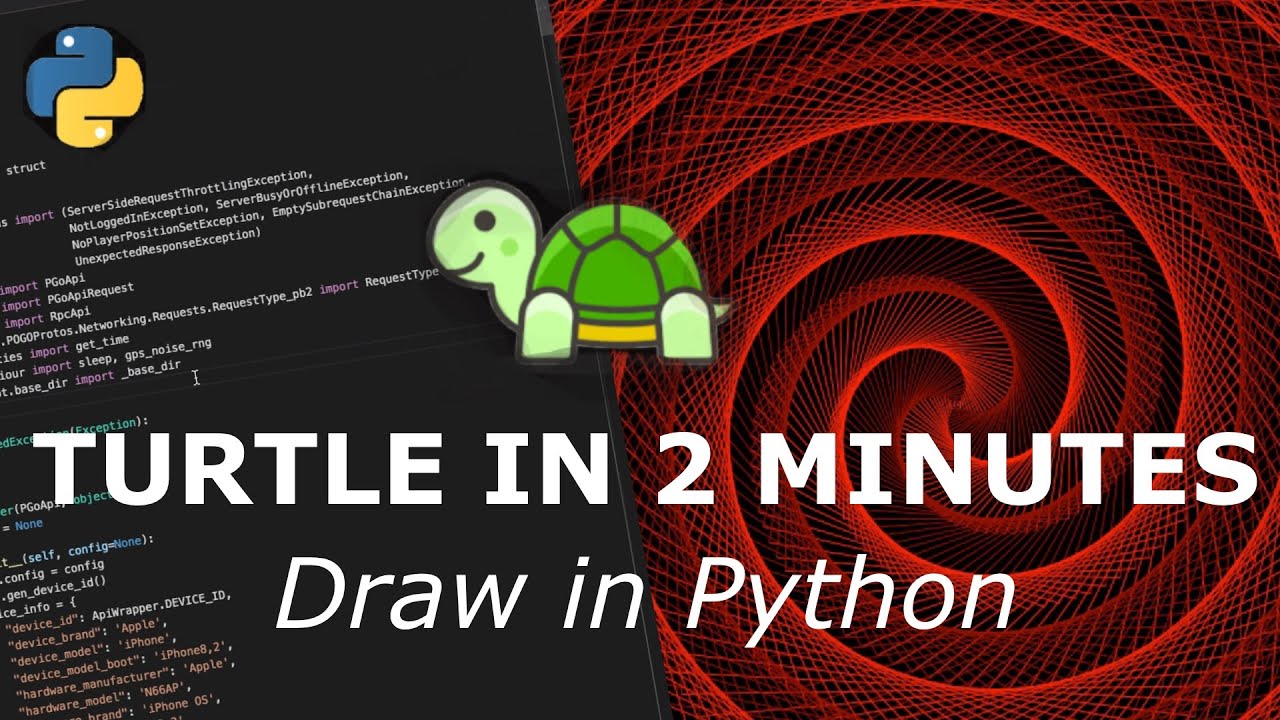
Показать описание
Learn programming in Turtle, a Python Library, in this 2 minute tutorial for beginners. Playing around with the Turtle library is a great way to practise the basics susch as variables, lists, looping, functions and more.
—————————————————————
Drawing with Turtle - Spirographic
—————————————————————
In this tutorial we will code this piece of art in turtle, which is a python library.
To use the turtle library start with ‘import turtle’
For the background color we can use the turtle function
Set the background color to black
Next we need to create the object turtle and store it in the variable ’t'.
The colours red and dark red are used. We can define them here in a list
Now as you saw in the animation. the code draws a line and then takes a turn
And then draws a line again.
Each line is drawn longer than the line before.
We can use a for loop here and loop about 400 times with the range function
With the forward function we draw a straight line.
The length of the line is defined by the value stored in the variable number
Since we are looping in the range from 0 to 400, we can re-use the variable for the length of the line
Next we need to define the angle.
If we enter 90 here, this loop would create a square.
We don’t want to it to be a perfect corner, but slightly off so it creates the spiral effect
Finally spiral alternates between red and dark red
In one iteration of the loop we want to use red, the next iteration dark red and keep alternating like that in each iteration.
We defined a list with the colors
Red has an index number of 0 and dark red has an index number of 1
So one iteration we need to pass the index number 0 and the next 1
We can do that by use the operator modulo, which is the percentage sign
This operator returns the remainder of a division problem
To show you how this works, lets visualise what the operator does
If we want to divide 5 by 2 with the modulo, what python does is, it makes in this case groups of 2.
If it is no longer able to make a group of 2, it returns the remainder. In this case one
Here’s an example of 4 divided by 2 with the out come 0
And here’s an example of 1 divided by 2
the modulo groups the input figure by 2 and if it cannot make a group of 2 anymore, then it returns the remainder
That’s how we can switch back and forth between red and dark red
And that’s it.
🦁 Who are you?
My name is Raza. I am 30. I am an IT - manager right now, but I’ve been an accountant for the majority of my career.
I’m in love with #Python :)
You can find me here:
—————————————————————————————
Goals for 2021
Training Python hours: 57/1000
Python/Django Projects: 2/30
Subscribers: 1999/10,000
—————————————————————————————-
#100SecondsOfCode
#pythonforbeginners #programming
—————————————————————
Drawing with Turtle - Spirographic
—————————————————————
In this tutorial we will code this piece of art in turtle, which is a python library.
To use the turtle library start with ‘import turtle’
For the background color we can use the turtle function
Set the background color to black
Next we need to create the object turtle and store it in the variable ’t'.
The colours red and dark red are used. We can define them here in a list
Now as you saw in the animation. the code draws a line and then takes a turn
And then draws a line again.
Each line is drawn longer than the line before.
We can use a for loop here and loop about 400 times with the range function
With the forward function we draw a straight line.
The length of the line is defined by the value stored in the variable number
Since we are looping in the range from 0 to 400, we can re-use the variable for the length of the line
Next we need to define the angle.
If we enter 90 here, this loop would create a square.
We don’t want to it to be a perfect corner, but slightly off so it creates the spiral effect
Finally spiral alternates between red and dark red
In one iteration of the loop we want to use red, the next iteration dark red and keep alternating like that in each iteration.
We defined a list with the colors
Red has an index number of 0 and dark red has an index number of 1
So one iteration we need to pass the index number 0 and the next 1
We can do that by use the operator modulo, which is the percentage sign
This operator returns the remainder of a division problem
To show you how this works, lets visualise what the operator does
If we want to divide 5 by 2 with the modulo, what python does is, it makes in this case groups of 2.
If it is no longer able to make a group of 2, it returns the remainder. In this case one
Here’s an example of 4 divided by 2 with the out come 0
And here’s an example of 1 divided by 2
the modulo groups the input figure by 2 and if it cannot make a group of 2 anymore, then it returns the remainder
That’s how we can switch back and forth between red and dark red
And that’s it.
🦁 Who are you?
My name is Raza. I am 30. I am an IT - manager right now, but I’ve been an accountant for the majority of my career.
I’m in love with #Python :)
You can find me here:
—————————————————————————————
Goals for 2021
Training Python hours: 57/1000
Python/Django Projects: 2/30
Subscribers: 1999/10,000
—————————————————————————————-
#100SecondsOfCode
#pythonforbeginners #programming
Комментарии