filmov
tv
Reverse String in Java Practice Program #41
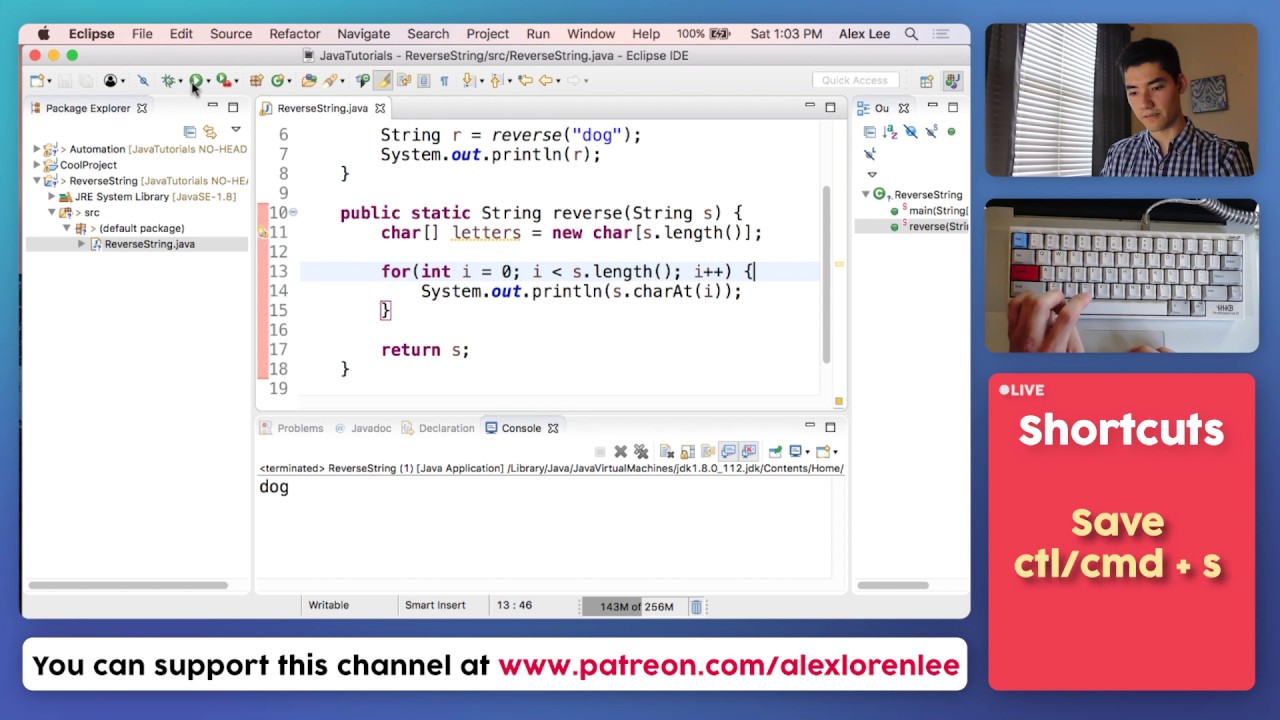
Показать описание
This tutorial will walk you through the reverse string in java program! ✅This is a fun little java program that might help you learn java :)
Each "letter" or symbol in a string is called a character. To reverse a string, all you have to do, is store each character, and then stick em backwards!
So:
"dog"
turns into:
Characters 'd' 'o' 'g'
which we can reverse:
'g' 'o' 'd'
and make the reversed string:
"god"
This reverse string in java program can be tricky at first... But SURELY you'll get it :) If you followed along, congrats! You learned-by-doing!
I hope you enjoyed this java program to reverse a string in java! I like to have a nice mix of tutorials and actual java projects for you all :)
Was this reverse string in java program helpful for you? -
Disclosure: The Springboard link provided is linked to my affiliate account & supports the channel.
~
Alex Lee
Reverse String in Java Practice Program #41
Reverse Strings in JAVA | (simple & easy)
Java Program #7 - Reverse a String in Java
Reversing a String | JAVA INTERVIEW QUESTIONS
Reverse String in Java Practice Program WITHOUT FOR LOOPS - Easy | Very Easy Coding Tutorial
Reverse A String In Java | Program To Reverse A String | Reverse String In Java | Java Interview
Frequently Asked Java Program 02: Reverse A Number | 3 Ways of Reverse a Number
How can you reverse a String? - Cracking the Java Coding Interview
Java Program to Reverse each Word of a String
How to reverse a string using Java 8 | Java Tutorial Series | HackCS
How to Reverse a String in Java | Coding Skills
LeetCode Reverse String Solution Explained - Java
Reverse String in Java | #Shorts
String program #2- Reverse A String In Java using Java8 Stream API | using Lambda expr by Naren
Shortcut to reverse a string - Java Program.
How To Reverse An Array In Java Explained With Debugging | Java Practice Programs | Code Bode
Java Program to Reverse a String with Explanation
Strings | Lecture 12 | Java Placement Series
#36 StringBuffer and StringBuilder in Java
Java Strings Set-1 | School Practice Problem | GeeksforGeeks School
I LOVE YOU program in C Language || #shorts || #CloudCODE
How to reverse a string in Java ? | Java string
Java Program to Reverse a Number | Learn Coding
Loops in Java (Exercise 9)
Комментарии