filmov
tv
Security Checks for Python Code
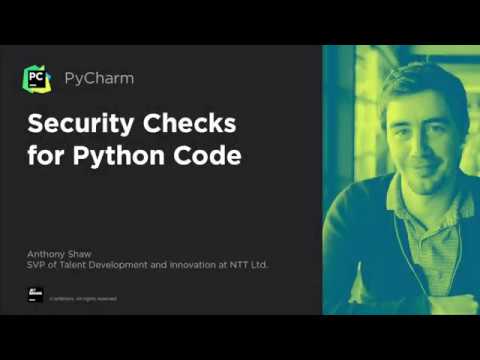
Показать описание
Software has security issues, #Python is software, so how do Python developers avoid common traps? In this webinar, Anthony Shaw discusses the topic of #security vulnerabilities, how code quality tools can help and demonstrates the #PyCharm plugin he wrote to let the IDE help.
Timeline:
00:30 - Demo the application being used
01:30 - Installing the plugin
03:49 - Show some reported vulnerabilities
04:28 - Running the checks manually
05:15 - First round of questions
11:20 - Investigate first vulnerability
15:30 - Second round of questions
16:20 - Browsing the shipped list of inspections/vulnerabilities
20:58 - Code inspection tool
26:58 - Third round of questions
30:38 - Django-specific app vulnerability
36:35 - Show documentation page with a full list of vulnerabilities
38:28 - Fourth round of questions
44:07 - Running checks in continuous integration (CI) via Docker image, headless PyCharm
47:07 - Final round of questions
51:18 - Suppressing warnings on a specific line
52:21 - "View on Marketplace" for the GitHub Action
Resources:
About the Presenter:
Anthony Shaw is a Python researcher from Australia. He publishes articles about Python, software, and automation to over 1 million readers annually. Anthony is an open-source software advocate, Fellow of the Python Software Foundation, and a member of the Apache Software Foundation.
Timeline:
00:30 - Demo the application being used
01:30 - Installing the plugin
03:49 - Show some reported vulnerabilities
04:28 - Running the checks manually
05:15 - First round of questions
11:20 - Investigate first vulnerability
15:30 - Second round of questions
16:20 - Browsing the shipped list of inspections/vulnerabilities
20:58 - Code inspection tool
26:58 - Third round of questions
30:38 - Django-specific app vulnerability
36:35 - Show documentation page with a full list of vulnerabilities
38:28 - Fourth round of questions
44:07 - Running checks in continuous integration (CI) via Docker image, headless PyCharm
47:07 - Final round of questions
51:18 - Suppressing warnings on a specific line
52:21 - "View on Marketplace" for the GitHub Action
Resources:
About the Presenter:
Anthony Shaw is a Python researcher from Australia. He publishes articles about Python, software, and automation to over 1 million readers annually. Anthony is an open-source software advocate, Fellow of the Python Software Foundation, and a member of the Apache Software Foundation.
Комментарии