filmov
tv
How to Detect Clicks on HTML Elements Outside Your React App
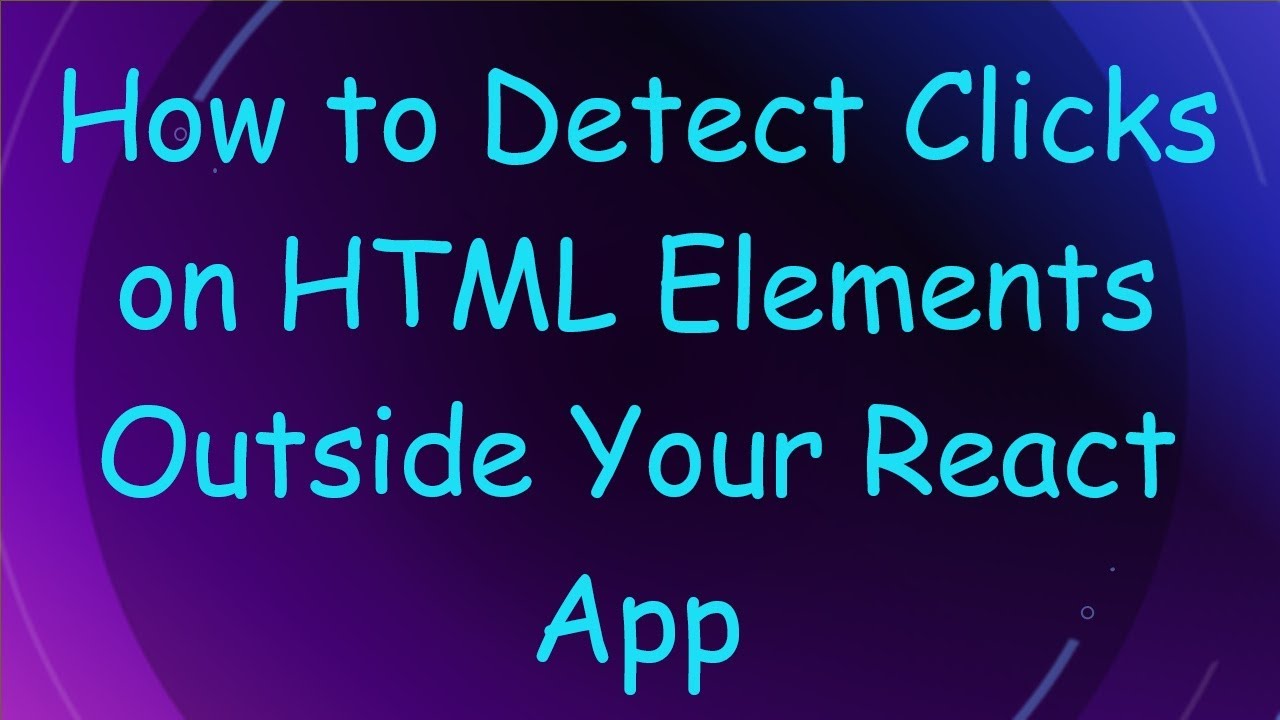
Показать описание
Learn how to effectively handle click events from static HTML elements in your React app by utilizing JavaScript event listeners. This step-by-step guide will help you interact seamlessly with your React components.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Detect click from an html element outside a React app and a react component
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Detect Clicks on HTML Elements Outside Your React App: A Simple Guide
In web development, it's common to encounter situations where you need to interact with HTML elements that sit outside your React application. Specifically, you might find yourself wanting to detect click events on plain HTML elements from within a React component. This can be particularly useful when your HTML page contains various static elements that users need to interact with.
In this guide, we'll explore how to listen to click events on static HTML elements using React features and JavaScript. Whether you're looking to improve user feedback or simply handle interactivity better, this post will provide clear solutions to your problem.
Problem Overview
Given an HTML structure like this:
[[See Video to Reveal this Text or Code Snippet]]
You want to listen for click events on the static element with the ID click. The challenge lies in the fact that this element isn't part of your React component, yet you need React to respond when it's clicked.
Solution Overview
To achieve this functionality, you can utilize the native addEventListener method in conjunction with React’s useEffect hook. This approach will enable you to capture click events from the static HTML element and respond appropriately within your React component.
Step-by-Step Implementation
Here’s how you can set it up:
Step 1: Set Up Your React Component
First, ensure you have a functional React component where you will implement the event listener.
[[See Video to Reveal this Text or Code Snippet]]
Step 2: Add the Click Event Listener
Use the useEffect hook to set up an event listener for the click events. Here's how you can do this:
[[See Video to Reveal this Text or Code Snippet]]
Step 3: Customize Your Listener
If you only want to respond to clicks on a specific element (like the div with ID click), you can refine the listener. Instead of capturing all clicks, check if the target of the click event is the desired element.
Here’s how you can do that:
[[See Video to Reveal this Text or Code Snippet]]
Step 4: Testing Your Component
Now you can test your component by rendering it on your HTML page. When you click on the static HTML element, you should see the appropriate console message or any other reaction you coded in your listener.
Conclusion
By employing the addEventListener method alongside React's useEffect, you can seamlessly detect click events from HTML elements that are outside your React components. This technique not only enhances your application’s interactivity but also allows for better integration between React and static web elements.
In summary, whether you're aiming to expand the functionality of a simple page or tackle complex user interactions, implementing event listeners in this way can significantly elevate the user experience.
Feel free to experiment with this approach and adjust the logic to fit your application's particular needs!
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Detect click from an html element outside a React app and a react component
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Detect Clicks on HTML Elements Outside Your React App: A Simple Guide
In web development, it's common to encounter situations where you need to interact with HTML elements that sit outside your React application. Specifically, you might find yourself wanting to detect click events on plain HTML elements from within a React component. This can be particularly useful when your HTML page contains various static elements that users need to interact with.
In this guide, we'll explore how to listen to click events on static HTML elements using React features and JavaScript. Whether you're looking to improve user feedback or simply handle interactivity better, this post will provide clear solutions to your problem.
Problem Overview
Given an HTML structure like this:
[[See Video to Reveal this Text or Code Snippet]]
You want to listen for click events on the static element with the ID click. The challenge lies in the fact that this element isn't part of your React component, yet you need React to respond when it's clicked.
Solution Overview
To achieve this functionality, you can utilize the native addEventListener method in conjunction with React’s useEffect hook. This approach will enable you to capture click events from the static HTML element and respond appropriately within your React component.
Step-by-Step Implementation
Here’s how you can set it up:
Step 1: Set Up Your React Component
First, ensure you have a functional React component where you will implement the event listener.
[[See Video to Reveal this Text or Code Snippet]]
Step 2: Add the Click Event Listener
Use the useEffect hook to set up an event listener for the click events. Here's how you can do this:
[[See Video to Reveal this Text or Code Snippet]]
Step 3: Customize Your Listener
If you only want to respond to clicks on a specific element (like the div with ID click), you can refine the listener. Instead of capturing all clicks, check if the target of the click event is the desired element.
Here’s how you can do that:
[[See Video to Reveal this Text or Code Snippet]]
Step 4: Testing Your Component
Now you can test your component by rendering it on your HTML page. When you click on the static HTML element, you should see the appropriate console message or any other reaction you coded in your listener.
Conclusion
By employing the addEventListener method alongside React's useEffect, you can seamlessly detect click events from HTML elements that are outside your React components. This technique not only enhances your application’s interactivity but also allows for better integration between React and static web elements.
In summary, whether you're aiming to expand the functionality of a simple page or tackle complex user interactions, implementing event listeners in this way can significantly elevate the user experience.
Feel free to experiment with this approach and adjust the logic to fit your application's particular needs!