filmov
tv
Destructuring Assignment in JavaScript - #41 @Everyday-Be-Coding
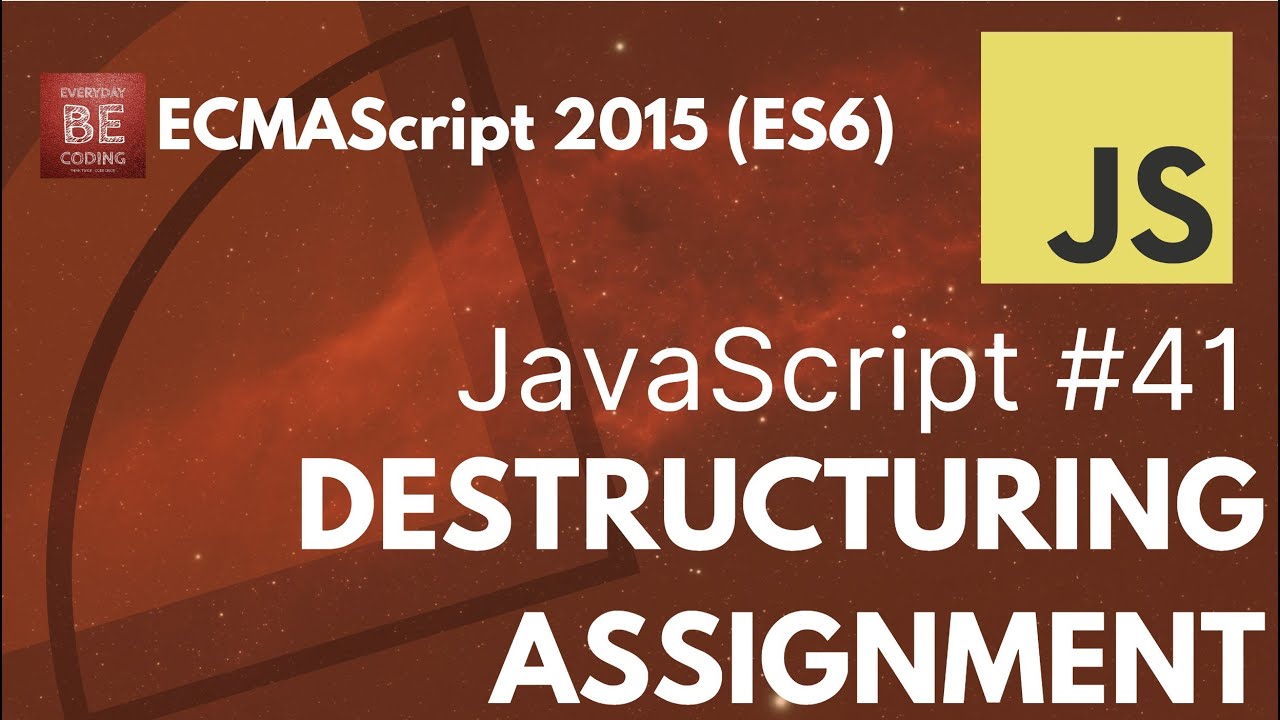
Показать описание
Destructuring assignment is a powerful feature introduced in ECMAScript 2015 (ES6) that allows you to extract values from arrays or properties from objects and assign them to variables in a concise and readable way. Here's how it works:
Array Destructuring:
You can destructure arrays by using square brackets []. The syntax looks like this:
JavaScript code:
const array = [1, 2, 3];
// Destructuring assignment
const [a, b, c] = array;
You can also skip elements by using commas:
JavaScript code:
const [first, , third] = array;
Object Destructuring:
You can destructure objects by using curly braces {}. The syntax looks like this:
JavaScript code
const obj = { x: 1, y: 2, z: 3 };
// Destructuring assignment
const { x, y, z } = obj;
You can also assign default values in case the property is not present in the object:
JavaScript code:
const { a = 10, b = 20, c = 30 } = obj;
Nested Destructuring:
You can destructure nested arrays and objects as well:
JavaScript code:
const nestedArray = [1, [2, 3], 4];
const [, [second, third]] = nestedArray;
JavaScript code:
const nestedObj = { outer: { inner: 42 } };
const { outer: { inner } } = nestedObj;
Rest Syntax:
You can use the rest syntax (...) to capture remaining elements of an array or remaining properties of an object:
javascript
Copy code
const numbers = [1, 2, 3, 4, 5];
const [first, ...rest] = numbers;
javascript
Copy code
const { x, ...restProps } = { x: 1, y: 2, z: 3 };
Destructuring assignment is a convenient way to work with arrays and objects in JavaScript, making code more concise and readable.
JavaScript Destructuring
ES6 Destructuring
ECMAScript 2015 Destructuring
JavaScript ES6 Features
Destructuring Assignment Tutorial
JavaScript Object Destructuring
Array Destructuring Example
Modern JavaScript Destructuring
Destructuring Assignment Syntax
Frontend Development
#JavaScript
#ES6
#Destructuring
#Programming
#WebDevelopment
#Coding
#JavaScriptTutorial
#Tech
#Frontend
#CodeTips
These hashtags can help
Chapter :
00:00 ES6 Destructuring
00:28 Array Destructuring
00:55 Object Destructuring
01:31 Nested Destructuring
01:56 Rest Syntax
02:24 Summery
Thank you for watching this video
EVERYDAY BE CODING