filmov
tv
Understanding Function Hoisting in JavaScript: Why Do Function Expressions Take Precedence?
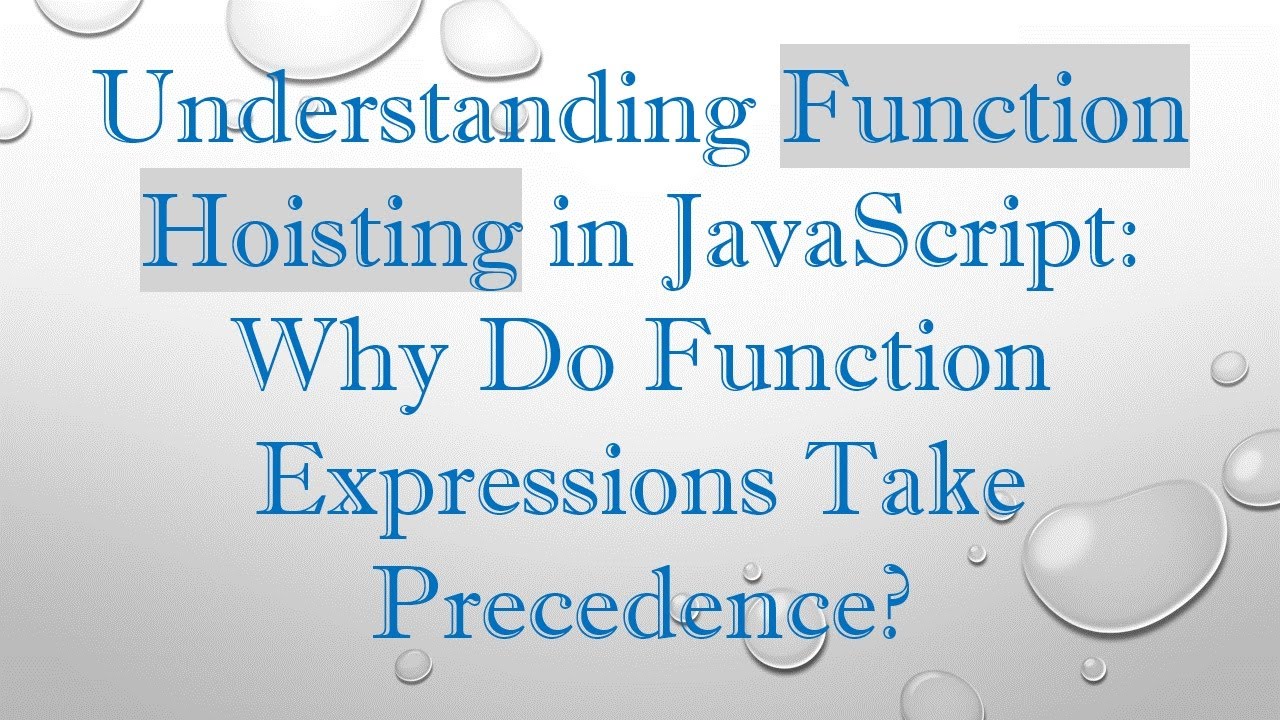
Показать описание
Discover why function expressions take precedence over function declarations in JavaScript and gain a deeper understanding of function hoisting.
---
Disclaimer/Disclosure: Some of the content was synthetically produced using various Generative AI (artificial intelligence) tools; so, there may be inaccuracies or misleading information present in the video. Please consider this before relying on the content to make any decisions or take any actions etc. If you still have any concerns, please feel free to write them in a comment. Thank you.
---
Understanding Function Hoisting in JavaScript: Why Do Function Expressions Take Precedence?
JavaScript is renowned for its peculiar behaviors, one of which is function hoisting. Hoisting is a mechanism where JavaScript moves function declarations to the top of the current scope before code execution. This allows for the usage of functions and variables even before they have been declared. However, in the scenario where both a function expression and a function declaration are present, it might lead to confusion. Let's delve deeper into why the function expression takes precedence over the function declaration.
Function Declarations vs. Function Expressions
Function Declarations
A function declaration is defined with the function keyword and has the following structure:
[[See Video to Reveal this Text or Code Snippet]]
Function declarations are hoisted entirely, including the body of the function. This means that you can invoke these functions before they appear in the source code.
Function Expressions
A function expression creates a function and assigns it to a variable:
[[See Video to Reveal this Text or Code Snippet]]
Function expressions are not hoisted in the same way. The variable declaration myFunction is hoisted, but it initially holds undefined until the function expression is evaluated.
The Precedence Rule
Case 1: Function Expression
[[See Video to Reveal this Text or Code Snippet]]
Case 2: Function Declaration
[[See Video to Reveal this Text or Code Snippet]]
When both the function expression and the function declaration are defined, the sequence in which they take precedence is key.
In this case, the function expression takes precedence over the function declaration due to the following reasons:
Hoisting Order: During the hoisting phase, JavaScript lifts declarations (both variables and functions) to the top of their scope. The function declaration is hoisted first, followed by variable declarations. However, the actual assignment from the function expression happens later after the initial hoisting phase. The most recently assigned value prevails, meaning the function expression overrides the hoisted function declaration.
Assignment and Execution Phase: After hoisting, JavaScript assigns the expressions. Since the variable myFunction is reassigned to the function expression, this assignment holds and is executed thereafter.
Code Example
Consider the following example:
[[See Video to Reveal this Text or Code Snippet]]
In this code snippet:
The function declaration for myFunction is hoisted and initially sets typeof myFunction to function.
The assignment through the function expression occurs during the execution phase, overriding the function declaration.
Conclusion
In JavaScript, function expressions take precedence over function declarations owing to the way hoisting and subsequent assignment work. Understanding function hoisting and the order of execution helps avoid unintended behaviors and bugs in your JavaScript code. Next time you define both a function declaration and a function expression, remember that the latter will take the final lead.
Understanding these nuances makes you better equipped to write cleaner, error-free JavaScript code.
---
Disclaimer/Disclosure: Some of the content was synthetically produced using various Generative AI (artificial intelligence) tools; so, there may be inaccuracies or misleading information present in the video. Please consider this before relying on the content to make any decisions or take any actions etc. If you still have any concerns, please feel free to write them in a comment. Thank you.
---
Understanding Function Hoisting in JavaScript: Why Do Function Expressions Take Precedence?
JavaScript is renowned for its peculiar behaviors, one of which is function hoisting. Hoisting is a mechanism where JavaScript moves function declarations to the top of the current scope before code execution. This allows for the usage of functions and variables even before they have been declared. However, in the scenario where both a function expression and a function declaration are present, it might lead to confusion. Let's delve deeper into why the function expression takes precedence over the function declaration.
Function Declarations vs. Function Expressions
Function Declarations
A function declaration is defined with the function keyword and has the following structure:
[[See Video to Reveal this Text or Code Snippet]]
Function declarations are hoisted entirely, including the body of the function. This means that you can invoke these functions before they appear in the source code.
Function Expressions
A function expression creates a function and assigns it to a variable:
[[See Video to Reveal this Text or Code Snippet]]
Function expressions are not hoisted in the same way. The variable declaration myFunction is hoisted, but it initially holds undefined until the function expression is evaluated.
The Precedence Rule
Case 1: Function Expression
[[See Video to Reveal this Text or Code Snippet]]
Case 2: Function Declaration
[[See Video to Reveal this Text or Code Snippet]]
When both the function expression and the function declaration are defined, the sequence in which they take precedence is key.
In this case, the function expression takes precedence over the function declaration due to the following reasons:
Hoisting Order: During the hoisting phase, JavaScript lifts declarations (both variables and functions) to the top of their scope. The function declaration is hoisted first, followed by variable declarations. However, the actual assignment from the function expression happens later after the initial hoisting phase. The most recently assigned value prevails, meaning the function expression overrides the hoisted function declaration.
Assignment and Execution Phase: After hoisting, JavaScript assigns the expressions. Since the variable myFunction is reassigned to the function expression, this assignment holds and is executed thereafter.
Code Example
Consider the following example:
[[See Video to Reveal this Text or Code Snippet]]
In this code snippet:
The function declaration for myFunction is hoisted and initially sets typeof myFunction to function.
The assignment through the function expression occurs during the execution phase, overriding the function declaration.
Conclusion
In JavaScript, function expressions take precedence over function declarations owing to the way hoisting and subsequent assignment work. Understanding function hoisting and the order of execution helps avoid unintended behaviors and bugs in your JavaScript code. Next time you define both a function declaration and a function expression, remember that the latter will take the final lead.
Understanding these nuances makes you better equipped to write cleaner, error-free JavaScript code.