filmov
tv
How to Catch Multiple Exceptions in Java
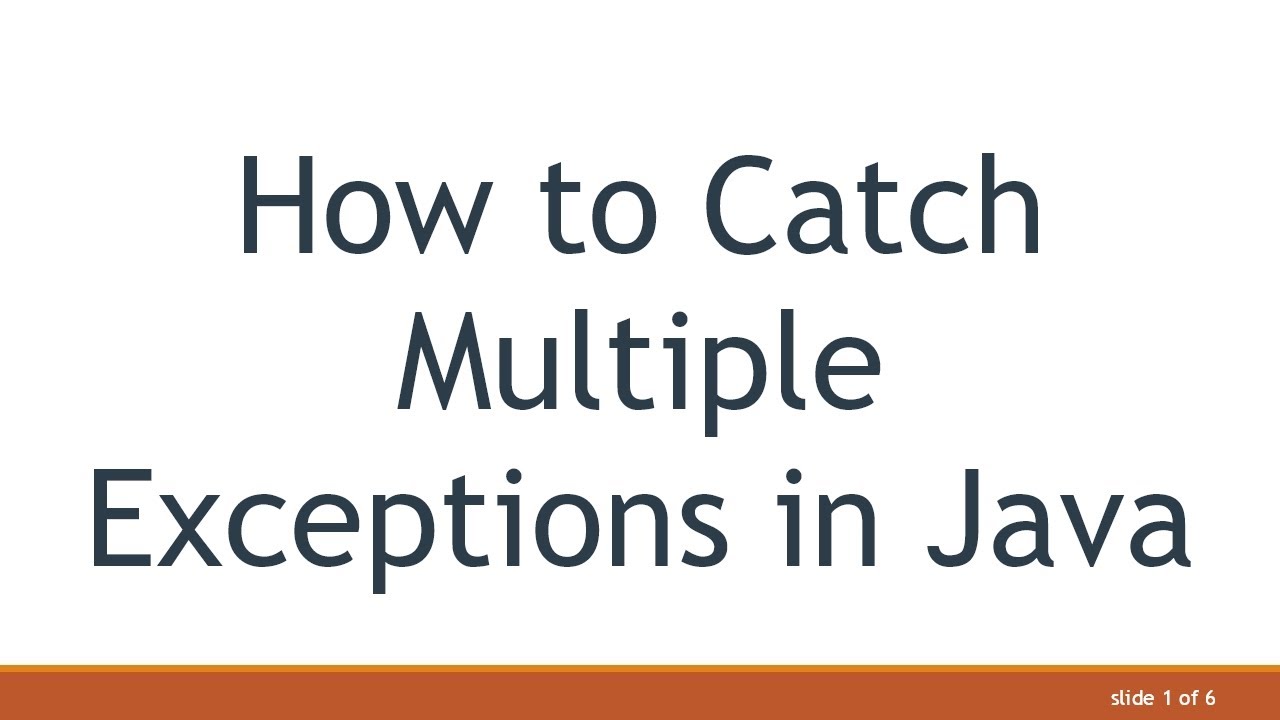
Показать описание
Learn how to effectively catch and handle multiple exceptions in Java with examples and best practices.
---
Disclaimer/Disclosure: Some of the content was synthetically produced using various Generative AI (artificial intelligence) tools; so, there may be inaccuracies or misleading information present in the video. Please consider this before relying on the content to make any decisions or take any actions etc. If you still have any concerns, please feel free to write them in a comment. Thank you.
---
Exception handling is a crucial aspect of writing robust and reliable Java code. While dealing with errors and exceptions, developers often encounter scenarios where multiple exceptions may occur within a single block of code. In such cases, it's essential to gracefully handle each exception type appropriately. In this guide, we will explore how to catch multiple exceptions in Java and discuss best practices.
Basic Exception Handling
Before diving into handling multiple exceptions, let's quickly review the basic structure of a try-catch block in Java:
[[See Video to Reveal this Text or Code Snippet]]
In the above example:
The try block contains the code that may throw an exception.
Multiple catch blocks handle specific exception types.
The finally block contains code that is executed regardless of whether an exception is thrown.
Handling Multiple Exceptions
Example 1: Catching Multiple Exceptions
[[See Video to Reveal this Text or Code Snippet]]
In this example, the catch block catches either an IOException or an SQLException. This feature was introduced in Java 7, allowing multiple exception types to be caught in a single catch block.
Example 2: Common Handling for Different Exceptions
[[See Video to Reveal this Text or Code Snippet]]
Here, both IOException and SQLException are caught in the same block because they share common handling logic. This helps reduce code duplication.
Best Practices
Specific Exception Handling: Catch specific exceptions before more general ones. This ensures that the most specific handlers are invoked.
Common Handling: If different exceptions share common handling logic, group them together in a single catch block.
Avoid Catching Generic Exceptions: While it's tempting to catch Exception for a catch-all scenario, it's generally better to catch specific exceptions to handle them appropriately.
Order Matters: The order of catch blocks is crucial. Place more specific exceptions before more general ones.
Keep it Concise: Aim for concise and readable exception handling code. Use separate catch blocks for distinct handling logic.
Conclusion
Effective exception handling is vital for writing robust and maintainable Java code. By understanding how to catch multiple exceptions and following best practices, developers can create more resilient applications. Remember to catch specific exceptions first, group common handling logic, and maintain a clean and readable code structure.
---
Disclaimer/Disclosure: Some of the content was synthetically produced using various Generative AI (artificial intelligence) tools; so, there may be inaccuracies or misleading information present in the video. Please consider this before relying on the content to make any decisions or take any actions etc. If you still have any concerns, please feel free to write them in a comment. Thank you.
---
Exception handling is a crucial aspect of writing robust and reliable Java code. While dealing with errors and exceptions, developers often encounter scenarios where multiple exceptions may occur within a single block of code. In such cases, it's essential to gracefully handle each exception type appropriately. In this guide, we will explore how to catch multiple exceptions in Java and discuss best practices.
Basic Exception Handling
Before diving into handling multiple exceptions, let's quickly review the basic structure of a try-catch block in Java:
[[See Video to Reveal this Text or Code Snippet]]
In the above example:
The try block contains the code that may throw an exception.
Multiple catch blocks handle specific exception types.
The finally block contains code that is executed regardless of whether an exception is thrown.
Handling Multiple Exceptions
Example 1: Catching Multiple Exceptions
[[See Video to Reveal this Text or Code Snippet]]
In this example, the catch block catches either an IOException or an SQLException. This feature was introduced in Java 7, allowing multiple exception types to be caught in a single catch block.
Example 2: Common Handling for Different Exceptions
[[See Video to Reveal this Text or Code Snippet]]
Here, both IOException and SQLException are caught in the same block because they share common handling logic. This helps reduce code duplication.
Best Practices
Specific Exception Handling: Catch specific exceptions before more general ones. This ensures that the most specific handlers are invoked.
Common Handling: If different exceptions share common handling logic, group them together in a single catch block.
Avoid Catching Generic Exceptions: While it's tempting to catch Exception for a catch-all scenario, it's generally better to catch specific exceptions to handle them appropriately.
Order Matters: The order of catch blocks is crucial. Place more specific exceptions before more general ones.
Keep it Concise: Aim for concise and readable exception handling code. Use separate catch blocks for distinct handling logic.
Conclusion
Effective exception handling is vital for writing robust and maintainable Java code. By understanding how to catch multiple exceptions and following best practices, developers can create more resilient applications. Remember to catch specific exceptions first, group common handling logic, and maintain a clean and readable code structure.