filmov
tv
TypeError float object is not iterable error in python 3
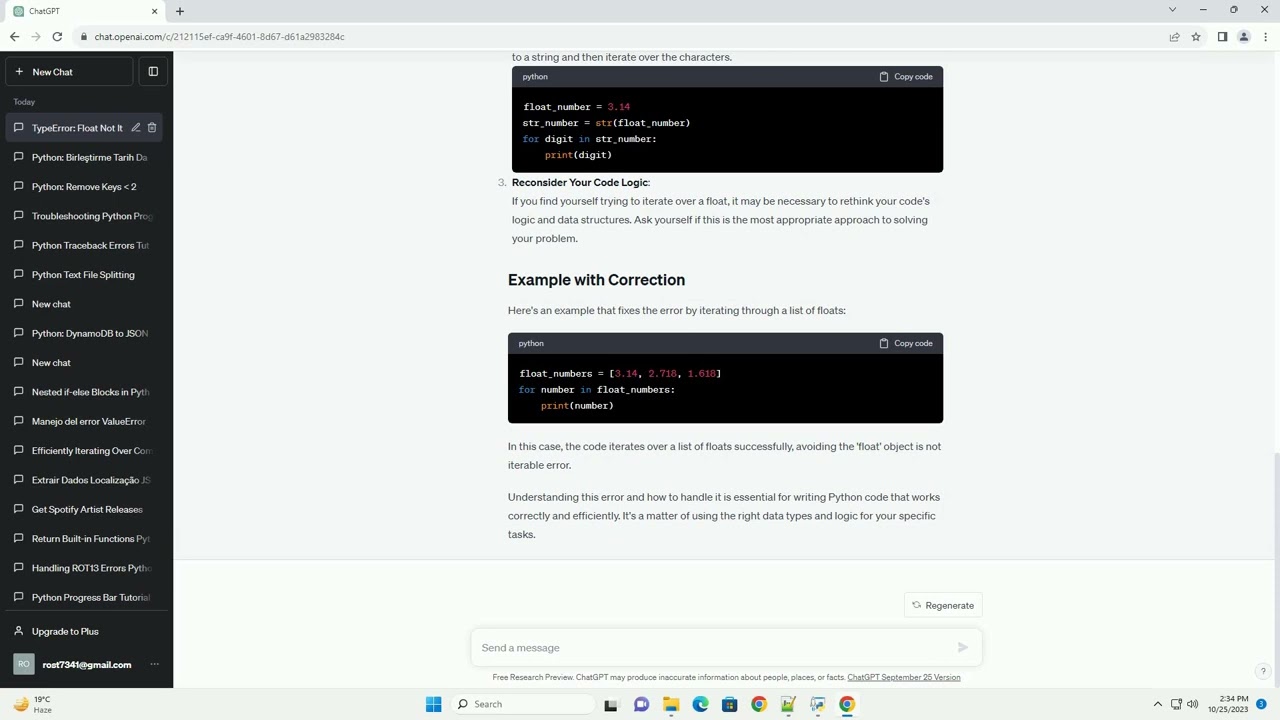
Показать описание
Python is a versatile programming language that allows you to work with various data types, including integers, strings, lists, and floats. However, it's essential to understand the limitations of each data type and how they can be used. One common error that developers encounter is the TypeError: 'float' object is not iterable. In this tutorial, we'll explain what this error means and provide examples of how it can occur.
The error message TypeError: 'float' object is not iterable indicates that you are trying to iterate over a float (a decimal number) as if it were an iterable data type. In Python, iteration is typically performed on objects like lists, tuples, strings, and dictionaries. These objects have multiple elements that can be accessed one at a time using a loop.
When you attempt to loop through a float, Python raises a TypeError because a float is a scalar data type, meaning it represents a single numeric value rather than a sequence of values. Python doesn't support iterating over a single numeric value like you would with an iterable data type.
Incorrect Use of for Loop:
This code will raise the 'float' object is not iterable error because you cannot iterate through a float like you would with a list or a string.
Accidental Mixing of Data Types:
In this example, the mixed_data list contains a float (3.14). The inner loop attempts to iterate through this float, causing the error.
To fix this error, you should review your code and identify the problematic section where you are mistakenly trying to iterate over a float. Depending on your intention, you can choose from the following solutions:
Check Data Types:
Ensure that you are trying to iterate over an iterable data type such as a list, string, or dictionary and not a float. If necessary, modify your data structures.
Use Indexing:
If you need to access individual digits in a floating-point number, you can convert the float to a string and then iterate over the characters.
Reconsider Your Code Logic:
If you find yourself trying to iterate over a float, it may be necessary to rethink your code's logic and data structures. Ask yourself if this is the most appropriate approach to solving your problem.
Here's an example that fixes the error by iterating through a list of floats:
In this case, the code iterates over a list of floats successfully, avoiding the 'float' object is not iterable error.
Understanding this error and how to handle it is essential for writing Python code that works correctly
The error message TypeError: 'float' object is not iterable indicates that you are trying to iterate over a float (a decimal number) as if it were an iterable data type. In Python, iteration is typically performed on objects like lists, tuples, strings, and dictionaries. These objects have multiple elements that can be accessed one at a time using a loop.
When you attempt to loop through a float, Python raises a TypeError because a float is a scalar data type, meaning it represents a single numeric value rather than a sequence of values. Python doesn't support iterating over a single numeric value like you would with an iterable data type.
Incorrect Use of for Loop:
This code will raise the 'float' object is not iterable error because you cannot iterate through a float like you would with a list or a string.
Accidental Mixing of Data Types:
In this example, the mixed_data list contains a float (3.14). The inner loop attempts to iterate through this float, causing the error.
To fix this error, you should review your code and identify the problematic section where you are mistakenly trying to iterate over a float. Depending on your intention, you can choose from the following solutions:
Check Data Types:
Ensure that you are trying to iterate over an iterable data type such as a list, string, or dictionary and not a float. If necessary, modify your data structures.
Use Indexing:
If you need to access individual digits in a floating-point number, you can convert the float to a string and then iterate over the characters.
Reconsider Your Code Logic:
If you find yourself trying to iterate over a float, it may be necessary to rethink your code's logic and data structures. Ask yourself if this is the most appropriate approach to solving your problem.
Here's an example that fixes the error by iterating through a list of floats:
In this case, the code iterates over a list of floats successfully, avoiding the 'float' object is not iterable error.
Understanding this error and how to handle it is essential for writing Python code that works correctly