filmov
tv
Creating a classfactory to Generate dataclass Variants in Python
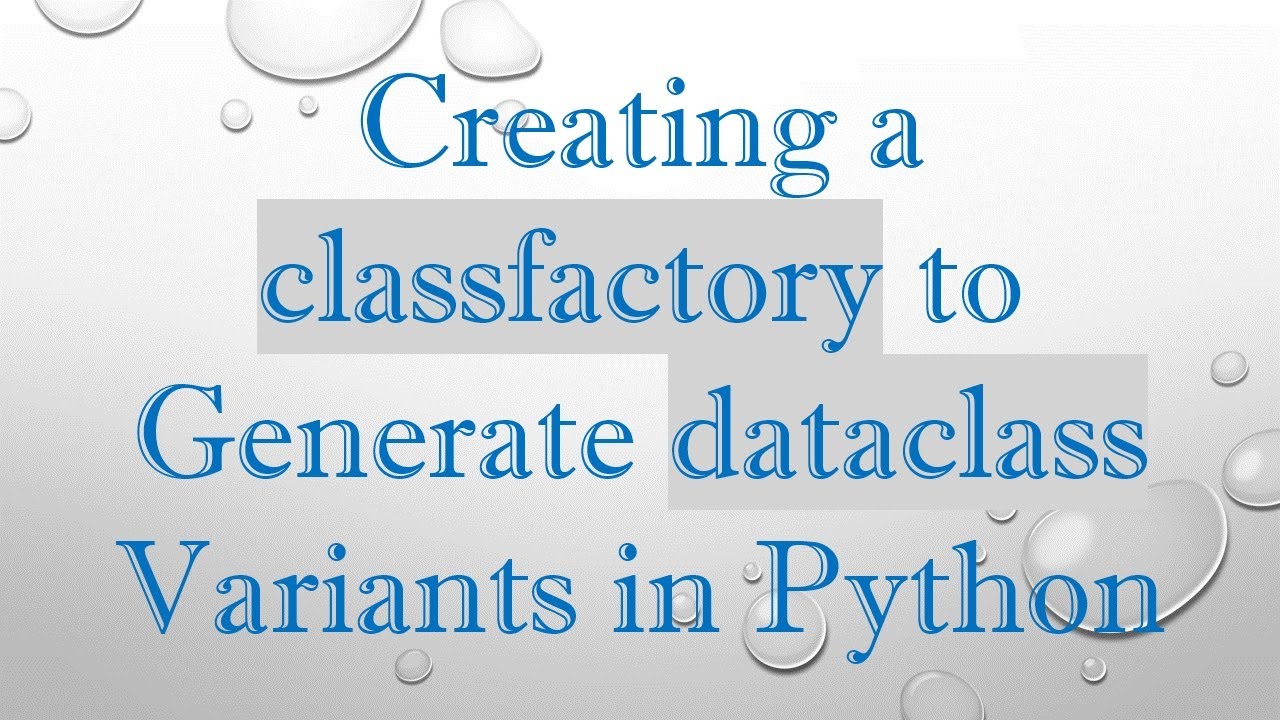
Показать описание
Learn how to create a `classfactory` in Python that transforms `dataclass` attributes into optional lists, simplifying data handling and filtering.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Making classfactory that derive from dataclass objects informed by types in python
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Creating a classfactory to Generate dataclass Variants in Python
Introduction
In the world of Python programming, particularly when dealing with data structures and types, dataclasses provide a clean and efficient way to manage your data attributes. However, when faced with a scenario where you need to extend the functionality of these dataclass definitions, complications can arise. One such scenario involves transforming attribute types from a simple data type to an optional list format.
In this guide, we will explore how to automate the creation of dataclass variants using a classfactory. This will allow us to quickly generate new classes that accommodate lists of optional types, providing a clear solution for data handling and filtering.
The Problem
Suppose you have a dataclass like below:
[[See Video to Reveal this Text or Code Snippet]]
You want to create a new class, SrcClassLister, that transforms each attribute type from X to Optional[List[X]]:
[[See Video to Reveal this Text or Code Snippet]]
The goal is to build a function called make_lister that can automatically generate such a class without painstakingly redefining each attribute manually.
The Solution
Step 1: Setup Your Environment
To get started, make sure you have the necessary imports set up for creating dataclasses and handling JSON serialization.
[[See Video to Reveal this Text or Code Snippet]]
Step 2: Define Your Original Dataclass
Our original dataclass will remain the same:
[[See Video to Reveal this Text or Code Snippet]]
Step 3: Create the make_lister Function
The heart of our solution lies in the make_lister function. This function will produce a new class by processing the provided dataclass:
[[See Video to Reveal this Text or Code Snippet]]
Step 4: Define a Helper Function for Pluralization
The helper function pluralize can improve naming conventions for your new fields:
[[See Video to Reveal this Text or Code Snippet]]
Step 5: Generate the New Class
Now, we use make_lister to create our SrcClassLister:
[[See Video to Reveal this Text or Code Snippet]]
Step 6: Testing the New Class
To confirm our implementation worked correctly, let’s instantiate the new class and print its details:
[[See Video to Reveal this Text or Code Snippet]]
Expected Output
When running the above code, we should see an output like:
[[See Video to Reveal this Text or Code Snippet]]
This confirms that our classfactory successfully transformed the original dataclass attributes into optional lists, allowing for effective filtering and handling of data.
Conclusion
With the make_lister function, we've created a flexible and powerful mechanism for generating data classes that cater to a variety of filtering needs. This approach minimizes repetitive manual coding and enhances the maintainability of your code.
By incorporating a classfactory in your Python projects, you can streamline your data management processes and focus on building robust applications. Happy coding!
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Making classfactory that derive from dataclass objects informed by types in python
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Creating a classfactory to Generate dataclass Variants in Python
Introduction
In the world of Python programming, particularly when dealing with data structures and types, dataclasses provide a clean and efficient way to manage your data attributes. However, when faced with a scenario where you need to extend the functionality of these dataclass definitions, complications can arise. One such scenario involves transforming attribute types from a simple data type to an optional list format.
In this guide, we will explore how to automate the creation of dataclass variants using a classfactory. This will allow us to quickly generate new classes that accommodate lists of optional types, providing a clear solution for data handling and filtering.
The Problem
Suppose you have a dataclass like below:
[[See Video to Reveal this Text or Code Snippet]]
You want to create a new class, SrcClassLister, that transforms each attribute type from X to Optional[List[X]]:
[[See Video to Reveal this Text or Code Snippet]]
The goal is to build a function called make_lister that can automatically generate such a class without painstakingly redefining each attribute manually.
The Solution
Step 1: Setup Your Environment
To get started, make sure you have the necessary imports set up for creating dataclasses and handling JSON serialization.
[[See Video to Reveal this Text or Code Snippet]]
Step 2: Define Your Original Dataclass
Our original dataclass will remain the same:
[[See Video to Reveal this Text or Code Snippet]]
Step 3: Create the make_lister Function
The heart of our solution lies in the make_lister function. This function will produce a new class by processing the provided dataclass:
[[See Video to Reveal this Text or Code Snippet]]
Step 4: Define a Helper Function for Pluralization
The helper function pluralize can improve naming conventions for your new fields:
[[See Video to Reveal this Text or Code Snippet]]
Step 5: Generate the New Class
Now, we use make_lister to create our SrcClassLister:
[[See Video to Reveal this Text or Code Snippet]]
Step 6: Testing the New Class
To confirm our implementation worked correctly, let’s instantiate the new class and print its details:
[[See Video to Reveal this Text or Code Snippet]]
Expected Output
When running the above code, we should see an output like:
[[See Video to Reveal this Text or Code Snippet]]
This confirms that our classfactory successfully transformed the original dataclass attributes into optional lists, allowing for effective filtering and handling of data.
Conclusion
With the make_lister function, we've created a flexible and powerful mechanism for generating data classes that cater to a variety of filtering needs. This approach minimizes repetitive manual coding and enhances the maintainability of your code.
By incorporating a classfactory in your Python projects, you can streamline your data management processes and focus on building robust applications. Happy coding!