filmov
tv
Find K Closest Elements - Leetcode 658 - Python
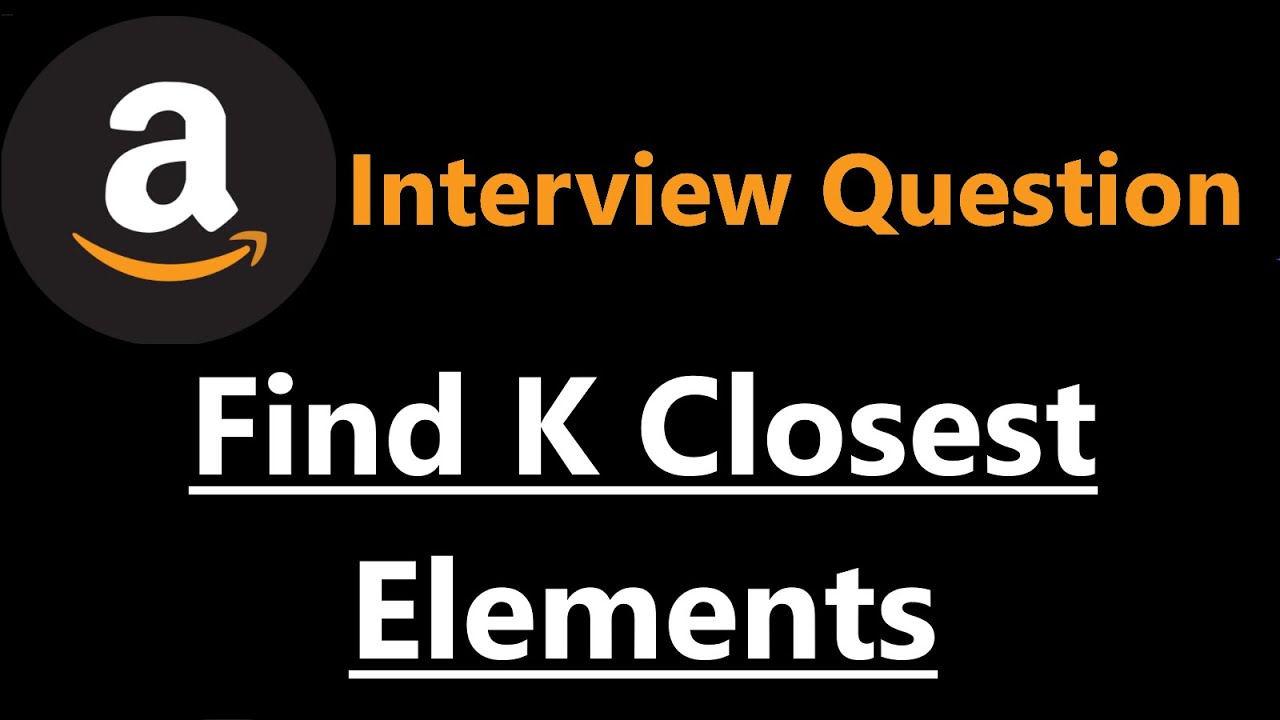
Показать описание
0:00 - Read the problem
1:50 - Drawing Explanation
15:01 - Coding Explanation
leetcode 658
#coding #interview #python
Disclosure: Some of the links above may be affiliate links, from which I may earn a small commission.
Find K Closest Elements - Leetcode 658 - Python
Coding Interview: Find K Closest Elements
658. Find K Closest Elements - Day 29/30 Leetcode September Challenge
658. Find k closest elements | Leetcode | Medium | Java | Binary Search | Two Pointers
658. Find K Closest Elements | LEETCODE MEDIUM
[Java] Leetcode 658. Find K Closest Elements [Binary Search #8]
Find K Closest Elements | LeetCode 658 | Java | Binary Search | @LearnOverflow
LeetCode 658. Find K Closest Elements
Find K Closest Elements | Leetcode 658 Solution | Searching and Sorting
5 K Closest Numbers
Find K Closest Elements 🔥🔥 | 3 approaches | Brute + Better + Optimal | Leetcode 658 | C++ | Python...
Find K Closest Elements - Optimised Approach | Leetcode 658 Solution | Searching and Sorting
Leetcode 658 Find K Closest Elements
LeetCode 658: Find K Closest Elements: Python Medium
POTD- 21/05/2024 | K Closest Elements | Problem of the Day | GeeksforGeeks Practice
Find K Closest Elements 🔥| Leetcode #658 Code Explained Hindi @HelloWorldbyprince Heap DSA playlist ...
Find K Closest Elements | Leetcode 658 | Live Coding session
K closest elements - 3 methods | gfg potd | GeeksForGeeks | Problem Of The Day | 21 May
658. Find K Closest Elements
LeetCode 658. Find K Closest Elements | JSer - JavaScript & Algorithm
Leetcode 658: Find K Closest Elements | Binary Search | Intuition and Approach
POTD- 21/05/2024 | K closest elements | Problem of the Day | GeeksforGeeks
Coding Interview Problem - Find K Closest Elements
Find K Closest Elements | LeetCode 658 | Coders Camp
Комментарии