filmov
tv
Creating the Perfect JPA Entity
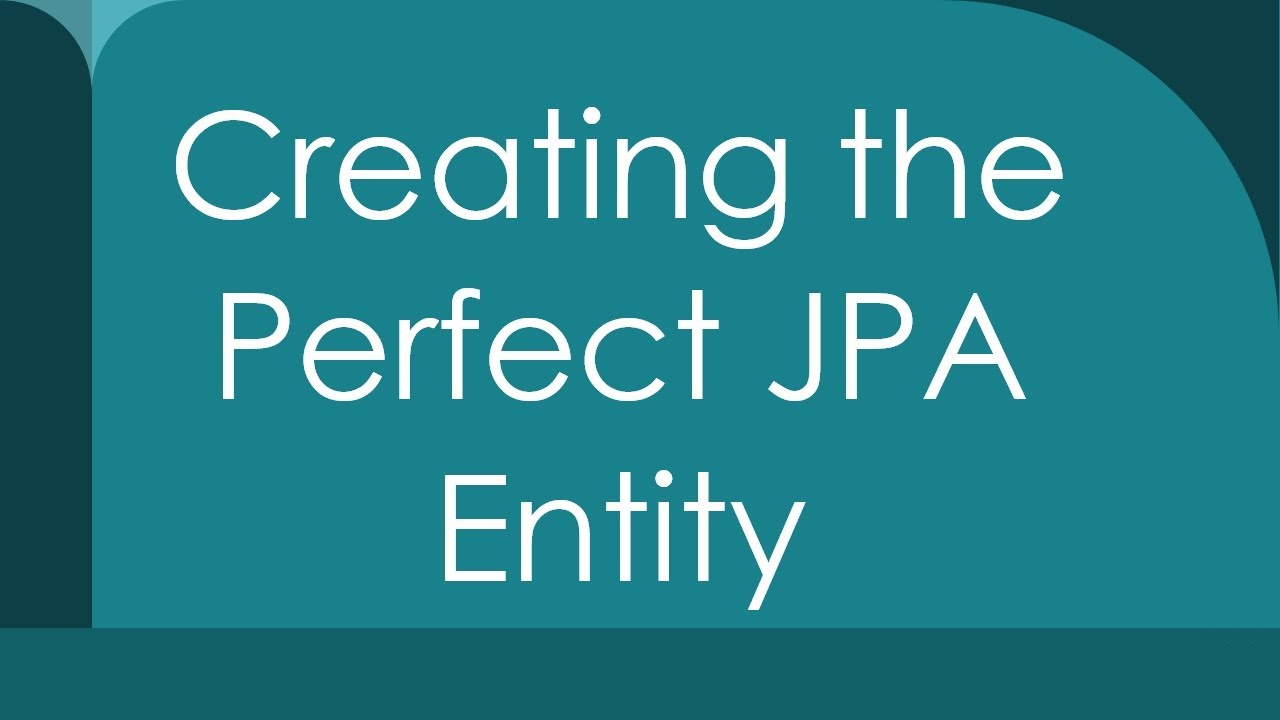
Показать описание
Summary: Learn how to create the perfect JPA entity with best practices and essential guidelines. Understand annotations, primary keys, and relationships to optimize your JPA entities.
---
Creating the Perfect JPA Entity: A Comprehensive Guide
Java Persistence API (JPA) simplifies the management of relational data in applications using Java. Creating the perfect JPA entity is essential for maintaining a clean, efficient, and scalable codebase. In this guide, we will explore best practices and guidelines to help you design optimal JPA entities.
Define the Entity Class
An entity in JPA is a lightweight, persistent domain object. To define an entity class, you need to annotate it with @Entity. Here is a basic example:
[[See Video to Reveal this Text or Code Snippet]]
The @Entity annotation specifies that the class is an entity and will be mapped to a table in the database.
Primary Key Annotations
Every entity must have a primary key, which uniquely identifies each record. The @Id annotation is used to denote the primary key:
[[See Video to Reveal this Text or Code Snippet]]
Here, @GeneratedValue is used to automatically generate the ID value. The strategy attribute specifies how the IDs should be generated (e.g., AUTO, IDENTITY, SEQUENCE, TABLE).
Column Mapping
You can customize the mapping between entity attributes and database columns with the @Column annotation:
[[See Video to Reveal this Text or Code Snippet]]
This annotation allows you to override the default column name and specify constraints like nullable, length, etc.
Relationships between Entities
Entities often have relationships with other entities. The major types of relationships are:
One-to-One:
[[See Video to Reveal this Text or Code Snippet]]
One-to-Many and Many-to-One:
[[See Video to Reveal this Text or Code Snippet]]
Many-to-Many:
[[See Video to Reveal this Text or Code Snippet]]
Use these annotations to define relationships, and manage the associated fetching and cascading behaviors efficiently.
Lifecycle Callbacks
JPA allows you to annotate methods with lifecycle callback annotations:
[[See Video to Reveal this Text or Code Snippet]]
These callbacks help manage entity states during the lifecycle events such as persistence, update, and deletion.
Implementing equals and hashCode
Override the equals and hashCode methods to ensure entity comparison is based on the identity and not the object reference:
[[See Video to Reveal this Text or Code Snippet]]
DTOs and Entity Projections
To prevent exposure of sensitive data and to optimize performance, use DTOs (Data Transfer Objects) and projections.
[[See Video to Reveal this Text or Code Snippet]]
Map entity data to the DTO when transferring between layers, thus ensuring a clear separation of concerns.
Conclusion
Creating the perfect JPA entity involves attention to detail and adherence to best practices. By following these guidelines, you can ensure that your entities are robust, maintainable, and performant. Whether you are defining relationships, handling lifecycle events, or mapping columns, each step is crucial for the efficient functioning of your application’s persistence layer.
Creating optimal entities not only enhances the integrity of your data but also improves the overall efficiency of your application, ensuring it is well-suited to handle future growth and complexity.
---
Creating the Perfect JPA Entity: A Comprehensive Guide
Java Persistence API (JPA) simplifies the management of relational data in applications using Java. Creating the perfect JPA entity is essential for maintaining a clean, efficient, and scalable codebase. In this guide, we will explore best practices and guidelines to help you design optimal JPA entities.
Define the Entity Class
An entity in JPA is a lightweight, persistent domain object. To define an entity class, you need to annotate it with @Entity. Here is a basic example:
[[See Video to Reveal this Text or Code Snippet]]
The @Entity annotation specifies that the class is an entity and will be mapped to a table in the database.
Primary Key Annotations
Every entity must have a primary key, which uniquely identifies each record. The @Id annotation is used to denote the primary key:
[[See Video to Reveal this Text or Code Snippet]]
Here, @GeneratedValue is used to automatically generate the ID value. The strategy attribute specifies how the IDs should be generated (e.g., AUTO, IDENTITY, SEQUENCE, TABLE).
Column Mapping
You can customize the mapping between entity attributes and database columns with the @Column annotation:
[[See Video to Reveal this Text or Code Snippet]]
This annotation allows you to override the default column name and specify constraints like nullable, length, etc.
Relationships between Entities
Entities often have relationships with other entities. The major types of relationships are:
One-to-One:
[[See Video to Reveal this Text or Code Snippet]]
One-to-Many and Many-to-One:
[[See Video to Reveal this Text or Code Snippet]]
Many-to-Many:
[[See Video to Reveal this Text or Code Snippet]]
Use these annotations to define relationships, and manage the associated fetching and cascading behaviors efficiently.
Lifecycle Callbacks
JPA allows you to annotate methods with lifecycle callback annotations:
[[See Video to Reveal this Text or Code Snippet]]
These callbacks help manage entity states during the lifecycle events such as persistence, update, and deletion.
Implementing equals and hashCode
Override the equals and hashCode methods to ensure entity comparison is based on the identity and not the object reference:
[[See Video to Reveal this Text or Code Snippet]]
DTOs and Entity Projections
To prevent exposure of sensitive data and to optimize performance, use DTOs (Data Transfer Objects) and projections.
[[See Video to Reveal this Text or Code Snippet]]
Map entity data to the DTO when transferring between layers, thus ensuring a clear separation of concerns.
Conclusion
Creating the perfect JPA entity involves attention to detail and adherence to best practices. By following these guidelines, you can ensure that your entities are robust, maintainable, and performant. Whether you are defining relationships, handling lifecycle events, or mapping columns, each step is crucial for the efficient functioning of your application’s persistence layer.
Creating optimal entities not only enhances the integrity of your data but also improves the overall efficiency of your application, ensuring it is well-suited to handle future growth and complexity.