filmov
tv
Dart Collection Tricks: Finding the Maximum and Minimum Values in Lists
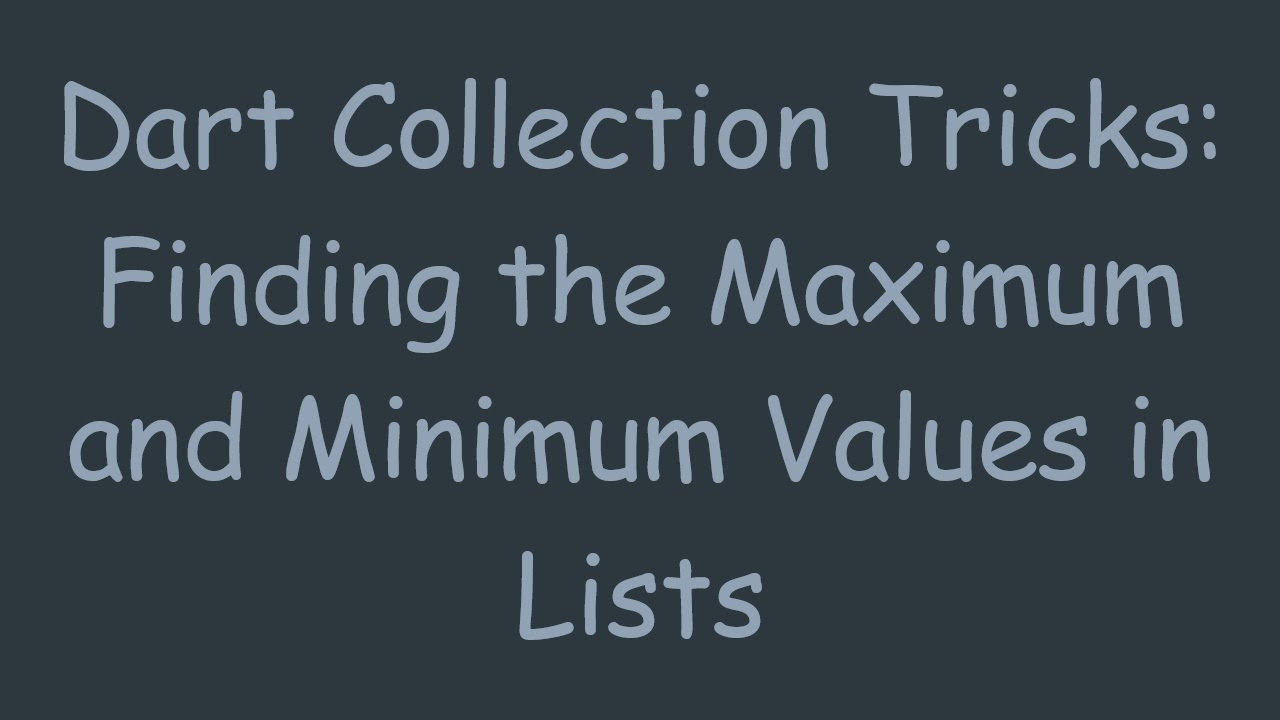
Показать описание
Learn how to efficiently find the maximum and minimum values in Dart lists using built-in methods and functional programming techniques. Discover straightforward approaches to streamline your code and handle various scenarios with ease.
---
Disclaimer/Disclosure: Some of the content was synthetically produced using various Generative AI (artificial intelligence) tools; so, there may be inaccuracies or misleading information present in the video. Please consider this before relying on the content to make any decisions or take any actions etc. If you still have any concerns, please feel free to write them in a comment. Thank you.
---
When working with collections in Dart, such as lists, you'll often encounter the need to find the maximum and minimum values within them. Dart provides several convenient ways to achieve this efficiently. Let's explore some tricks to accomplish these tasks effectively.
Finding the Maximum Value
[[See Video to Reveal this Text or Code Snippet]]
In this example, reduce(max) iterates through the list and compares each element to determine the maximum value efficiently.
Finding the Minimum Value
Similarly, you can find the minimum value using reduce with min from dart:math.
[[See Video to Reveal this Text or Code Snippet]]
Here, reduce(min) traverses the list to identify the smallest element.
Custom Comparisons
[[See Video to Reveal this Text or Code Snippet]]
In this case, the custom function passed to reduce compares Person objects based on their age property to find the oldest person.
Handling Edge Cases
When working with empty lists or lists of null values, be mindful of potential null or empty checks to avoid runtime errors.
[[See Video to Reveal this Text or Code Snippet]]
Always consider edge cases to ensure robustness in your code.
By leveraging these Dart collection tricks, you can efficiently find the maximum and minimum values in lists, whether dealing with primitive types or custom objects, and handle various scenarios with confidence.
---
Disclaimer/Disclosure: Some of the content was synthetically produced using various Generative AI (artificial intelligence) tools; so, there may be inaccuracies or misleading information present in the video. Please consider this before relying on the content to make any decisions or take any actions etc. If you still have any concerns, please feel free to write them in a comment. Thank you.
---
When working with collections in Dart, such as lists, you'll often encounter the need to find the maximum and minimum values within them. Dart provides several convenient ways to achieve this efficiently. Let's explore some tricks to accomplish these tasks effectively.
Finding the Maximum Value
[[See Video to Reveal this Text or Code Snippet]]
In this example, reduce(max) iterates through the list and compares each element to determine the maximum value efficiently.
Finding the Minimum Value
Similarly, you can find the minimum value using reduce with min from dart:math.
[[See Video to Reveal this Text or Code Snippet]]
Here, reduce(min) traverses the list to identify the smallest element.
Custom Comparisons
[[See Video to Reveal this Text or Code Snippet]]
In this case, the custom function passed to reduce compares Person objects based on their age property to find the oldest person.
Handling Edge Cases
When working with empty lists or lists of null values, be mindful of potential null or empty checks to avoid runtime errors.
[[See Video to Reveal this Text or Code Snippet]]
Always consider edge cases to ensure robustness in your code.
By leveraging these Dart collection tricks, you can efficiently find the maximum and minimum values in lists, whether dealing with primitive types or custom objects, and handle various scenarios with confidence.