filmov
tv
Dart Collections: Arrays or LIST as Fixed-length List. Dart for Flutter #11.1
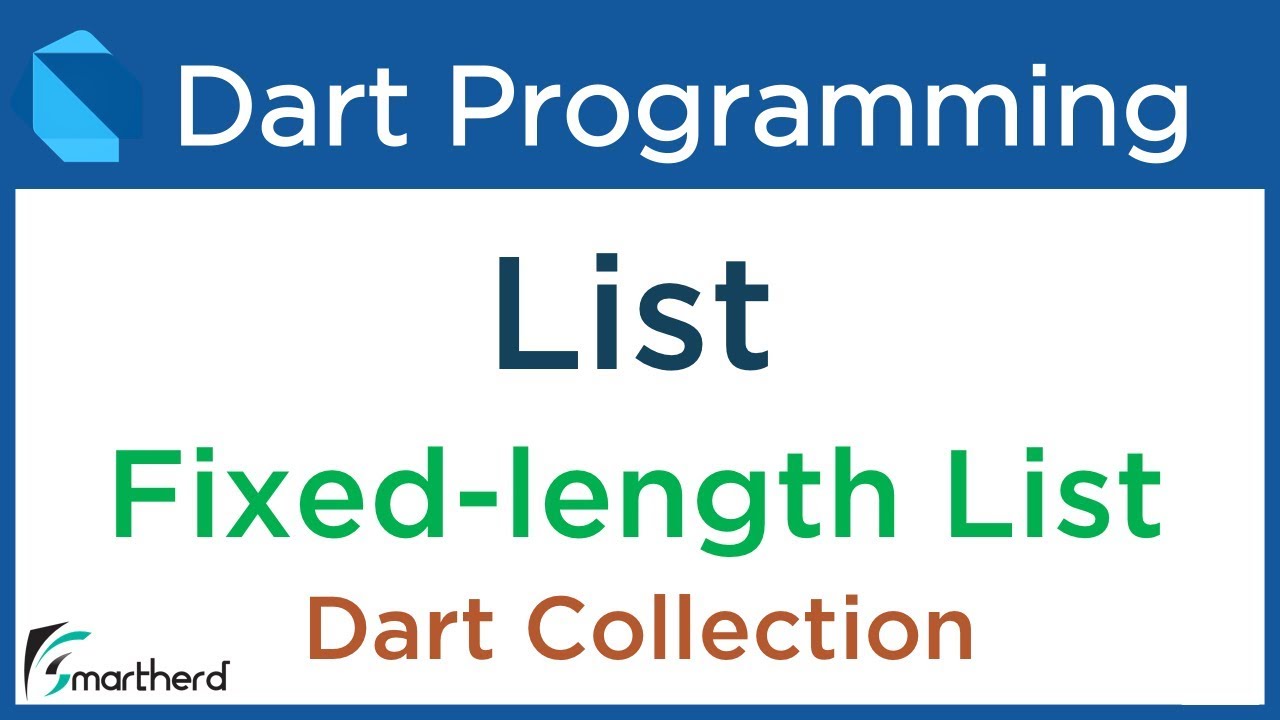
Показать описание
Dart beginners guide. Explore Arrays or Fixed length List in Ordered Dart Collections. Dart supports various Collection types such as Set, Map, HashSet, HashMap, Queue, LinkedList. Let us explore each one of them in this section.
.
Please donate and support my work
(If you think my free tutorials are better than paid ones :)
Free Programming courses:
Free Flutter course:
Free Android courses:
More free programming courses:
Check out my website:
Let's get in touch! [Sriyank Siddhartha]
---- Thank you for your love and support ----
Dart Collections: Arrays or LIST as Fixed-length List. Dart for Flutter #11.1
Dart Collections - Lists
Dart Collections: Arrays as List: Growable List. Dart for Flutter #11.2
DART COLLECTION - LIST AS IN ARRAYS - BEGINNERS TUTORIALS
10 Must Know Dart Array Methods
Arrays Or Lists in Dart #shorts
Collections In Dart - Dart Tips, Ep 5
Mastering Lists in Dart: Adding Values, Final vs Const in list, Spread Operator & More | Hindi
#5 DART: Arrays and array list
12- Dart Lists
#22 Collection pada dart - LIST
Section 4- Dart Collections (Lists, Maps and Sets) and Generics
DART COLLECTION - GROWABLE LIST & QUEUE - TUTORIALS
2. List and Map Data Types in Dart | Dart Fundamentals Course | Become Dart Language Master
Dart/Flutter Array List(How to Create Array List in Dart/Flutter)
#76 dart collection Map VS HashMap
Dart Collection : List, Sets, Maps
#40 - Dart Collections : List - Array as Growable List | Dart Programming for flutter in telugu
40 Dart Collection List & Map
Dart Features For Better Code: Spreads, Collection-If, Collection-For
41 Dart Collection List
Dart Collections - Sets
Coding for 1 Month Versus 1 Year #shorts #coding
List in Dart Concept Tutorial for Beginners (Hindi) | Flutter🔥
Комментарии