filmov
tv
C++ Weekly - Ep 264 - Covariant Return Types and Covariant Smart Pointers
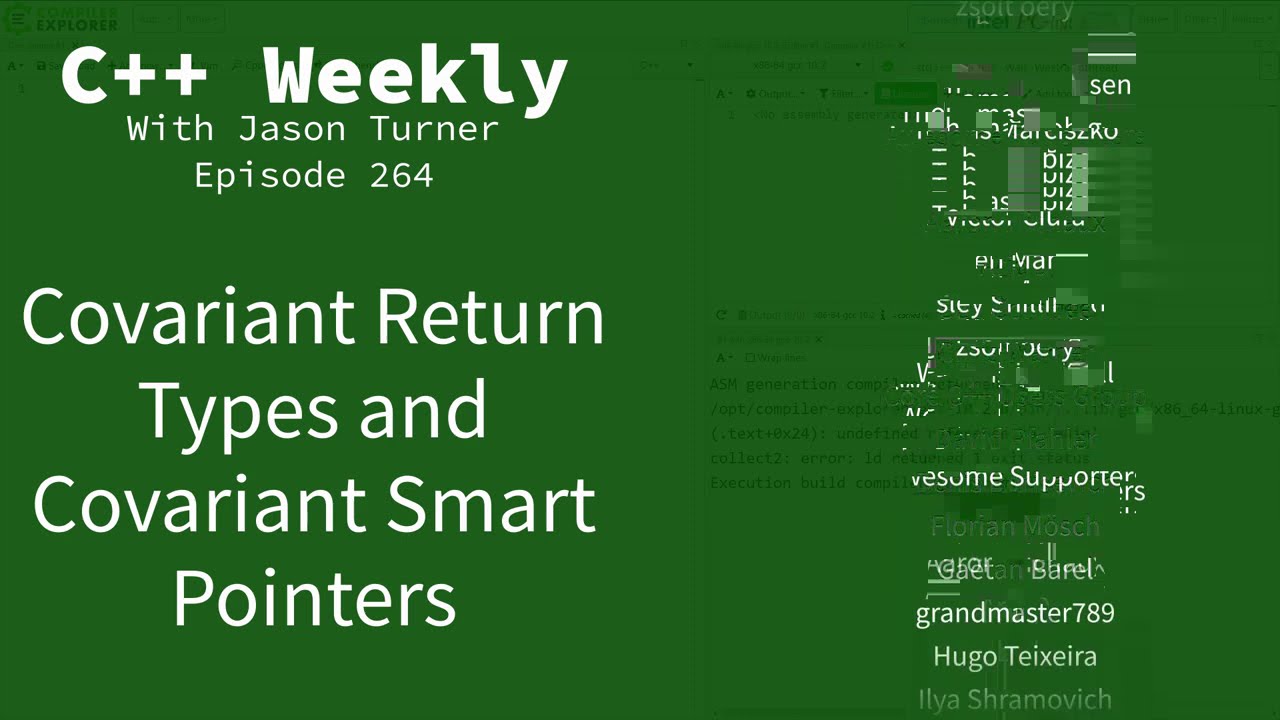
Показать описание
☟☟ Awesome T-Shirts! Sponsors! Books! ☟☟
T-SHIRTS AVAILABLE!
WANT MORE JASON?
SUPPORT THE CHANNEL
GET INVOLVED
JASON'S BOOKS
► C++23 Best Practices
► C++ Best Practices
JASON'S PUZZLE BOOKS
► Object Lifetime Puzzlers Book 1
► Object Lifetime Puzzlers Book 2
► Object Lifetime Puzzlers Book 3
► Copy and Reference Puzzlers Book 1
► Copy and Reference Puzzlers Book 2
► Copy and Reference Puzzlers Book 3
► OpCode Puzzlers Book 1
RECOMMENDED BOOKS
AWESOME PROJECTS
O'Reilly VIDEOS
T-SHIRTS AVAILABLE!
WANT MORE JASON?
SUPPORT THE CHANNEL
GET INVOLVED
JASON'S BOOKS
► C++23 Best Practices
► C++ Best Practices
JASON'S PUZZLE BOOKS
► Object Lifetime Puzzlers Book 1
► Object Lifetime Puzzlers Book 2
► Object Lifetime Puzzlers Book 3
► Copy and Reference Puzzlers Book 1
► Copy and Reference Puzzlers Book 2
► Copy and Reference Puzzlers Book 3
► OpCode Puzzlers Book 1
RECOMMENDED BOOKS
AWESOME PROJECTS
O'Reilly VIDEOS
C++ Weekly - Ep 264 - Covariant Return Types and Covariant Smart Pointers
PBLV - Saison 2, Épisode 264 | Damien reste à Marseille
When Phil Met Steven Tyler, Willie's Diet Competition, and Churches and Gender Pronouns | Ep 26...
Esaret 264. Bölüm | Redemption Episode 264
Uppum Mulakum 2│Flowers│EP# 264
Mid Week Car Boot Sale Hunting - Ep #264
Jijaji Chhat Per Hai - Ep 264 - Full Episode - 8th January, 2019
ZU Party | Ep. 264 @ C PUB / Drobeta Turnu Severin
Flowers Top Singer | Musical Reality Show | Ep#264 ( Part - C )
Sajan Re Phir Jhoot Mat Bolo - Ep 264 - Full Episode - 31st May, 2018
Ep:264 PROOF THAT PLANTS AND VEGANISM IS NOT A HUMAN DIET - by Robert Cywes
Barbie - Stars For Preschool | Ep.265
Long-Haired Local Chases Me Down - Bicycle Touring Pro / EP. #264
Multi-Dimensional Array | Ep. 264 | C Programming Language
D.B. COOPER: SALTOU DE UM 727 COM 1 MILHÃO DE DÓLARES | EP. 264
C++ Weekly - Ep 261 - C++20's New consteval Keyword
C++ Weekly - Ep 265 - C++20's std::bit_cast
MAN AGAINST MOISTURE! (Being a live-aboard in the cold) | EP 264
Chakkappazham 2│Comedy Series│EP# 264
News in 90 EP 264: Top 5 Christmas Stocking Fillers
TRANSACTION GONE WRONG (Yawaskits - Episode 264) Kalistus
Rika Chan is so cute 🥰 | Jujutsu kaisen manga edit #jujutsukaisen #shorts
Kards 4K 60FPS UHD Without Commentary Episode 264
kurulus Osman Season 5 Behind the scene part 32 😂#interestingshorts#shorts #viral #trending #foryou...
Комментарии