filmov
tv
How to Swap Columns of a Matrix in Python Without NumPy
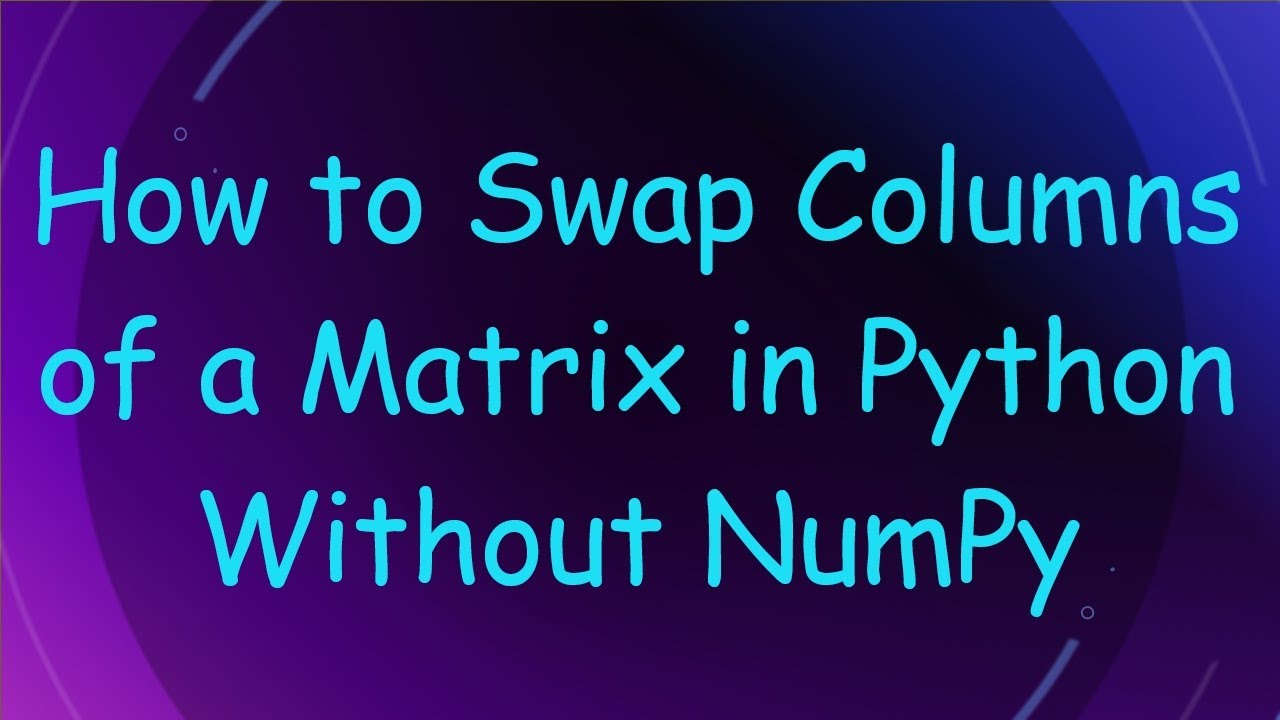
Показать описание
Learn how to swap columns in a matrix using Python from scratch, without relying on NumPy. This guide provides simple solutions and explanations to help you understand the concept better.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Swapping columns of a matrix without numpy
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Swapping Columns of a Matrix in Python without NumPy
In programming, manipulating data structures efficiently is crucial, particularly when working with matrices. One common task is swapping columns in a matrix. If you're using Python but want to avoid external libraries like NumPy, you might be facing some hurdles. Let’s unlock the solution and guide you through the process of swapping columns in a matrix without using NumPy.
Understanding the Problem
When we talk about a matrix, we typically refer to a two-dimensional array where you can access elements using row and column indices. In Python, this can be represented as a list of lists. The requirement here is to swap two columns in a given matrix M by providing the indices i and j.
Example:
Given a matrix M:
[[See Video to Reveal this Text or Code Snippet]]
If we want to swap column 0 (1, 4, 7) with column 2 (3, 6, 9), the resulting matrix should look like:
[[See Video to Reveal this Text or Code Snippet]]
Implementing the Solution
Let’s walk through a clear and effective solution.
Step 1: Create a Function
We’ll define a function called my_swap that takes three parameters: the matrix M, and the indices of the two columns i and j that we want to swap.
Step 2: Copy the Original Matrix
Using the copy module helps us avoid modifying the original matrix. This is essential because we want to retain the original data for validation or further processing.
Step 3: Perform the Swap Operation
Utilizing a loop, we will iterate over each row of the matrix and swap the specified columns.
Here’s the Complete Function:
[[See Video to Reveal this Text or Code Snippet]]
Explanation of the Code:
import copy: This line imports the copy module which allows us to create a deep copy of the matrix.
for k in range(len(M)):: Loops through each row of the matrix.
temp[k][i], temp[k][j] = M[k][j], M[k][i]: This line swaps the elements in column i and column j of the copied matrix.
Testing the Function
To ensure our function is correct, let’s test it with an example:
[[See Video to Reveal this Text or Code Snippet]]
Expected Output
[[See Video to Reveal this Text or Code Snippet]]
This output confirms that the columns have been swapped successfully!
Conclusion
Swapping columns in a matrix doesn't need to be complex, even in Python without NumPy. By utilizing built-in functions and clear logic, you can easily implement such features in your programs.
By following this guide, you should have a solid understanding of how to swap columns effectively. This foundational skill can aid in a variety of data manipulation tasks that you may encounter in the world of programming. Happy coding!
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Swapping columns of a matrix without numpy
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Swapping Columns of a Matrix in Python without NumPy
In programming, manipulating data structures efficiently is crucial, particularly when working with matrices. One common task is swapping columns in a matrix. If you're using Python but want to avoid external libraries like NumPy, you might be facing some hurdles. Let’s unlock the solution and guide you through the process of swapping columns in a matrix without using NumPy.
Understanding the Problem
When we talk about a matrix, we typically refer to a two-dimensional array where you can access elements using row and column indices. In Python, this can be represented as a list of lists. The requirement here is to swap two columns in a given matrix M by providing the indices i and j.
Example:
Given a matrix M:
[[See Video to Reveal this Text or Code Snippet]]
If we want to swap column 0 (1, 4, 7) with column 2 (3, 6, 9), the resulting matrix should look like:
[[See Video to Reveal this Text or Code Snippet]]
Implementing the Solution
Let’s walk through a clear and effective solution.
Step 1: Create a Function
We’ll define a function called my_swap that takes three parameters: the matrix M, and the indices of the two columns i and j that we want to swap.
Step 2: Copy the Original Matrix
Using the copy module helps us avoid modifying the original matrix. This is essential because we want to retain the original data for validation or further processing.
Step 3: Perform the Swap Operation
Utilizing a loop, we will iterate over each row of the matrix and swap the specified columns.
Here’s the Complete Function:
[[See Video to Reveal this Text or Code Snippet]]
Explanation of the Code:
import copy: This line imports the copy module which allows us to create a deep copy of the matrix.
for k in range(len(M)):: Loops through each row of the matrix.
temp[k][i], temp[k][j] = M[k][j], M[k][i]: This line swaps the elements in column i and column j of the copied matrix.
Testing the Function
To ensure our function is correct, let’s test it with an example:
[[See Video to Reveal this Text or Code Snippet]]
Expected Output
[[See Video to Reveal this Text or Code Snippet]]
This output confirms that the columns have been swapped successfully!
Conclusion
Swapping columns in a matrix doesn't need to be complex, even in Python without NumPy. By utilizing built-in functions and clear logic, you can easily implement such features in your programs.
By following this guide, you should have a solid understanding of how to swap columns effectively. This foundational skill can aid in a variety of data manipulation tasks that you may encounter in the world of programming. Happy coding!