filmov
tv
Python Trick: Use str.removeprefix() and str.removesuffix() for Cleaner String Manipulation!
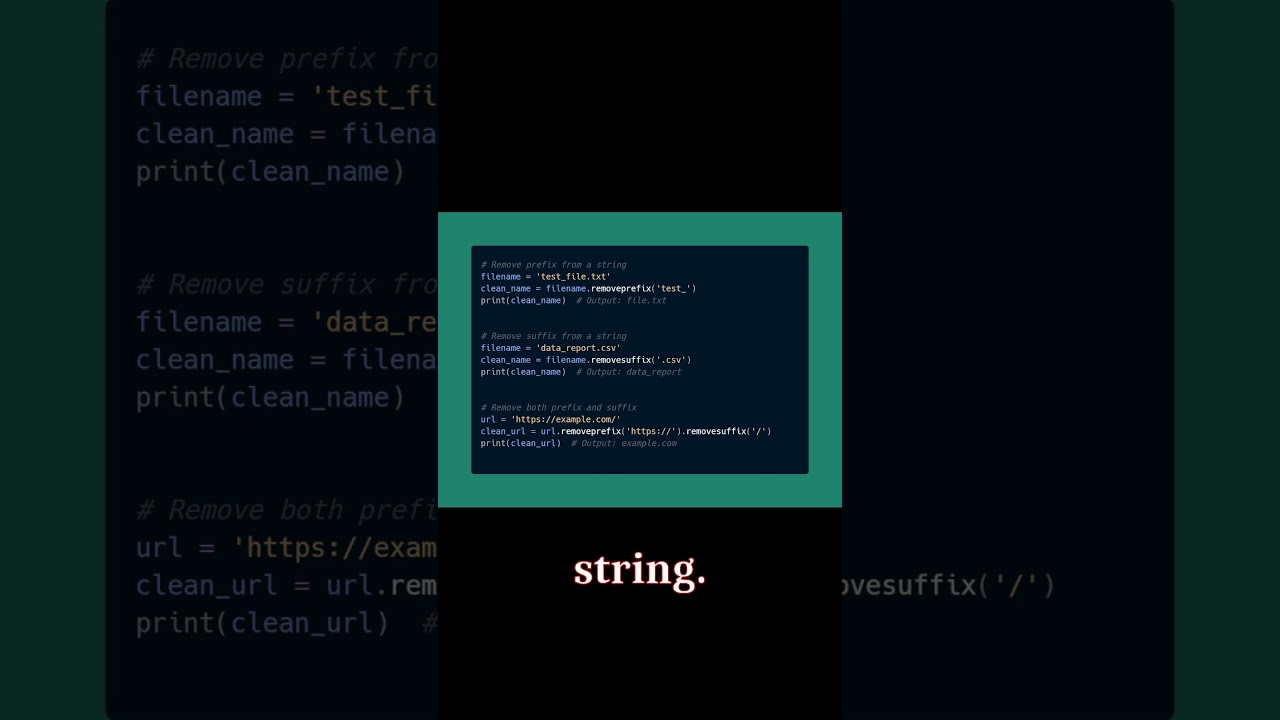
Показать описание
Tired of removing prefixes and suffixes from strings with clunky if statements or replace()?
Python 3.9+ introduced two handy methods:
removeprefix(prefix): Removes the prefix from a string.
removesuffix(suffix): Removes the suffix from a string.
These methods are cleaner, more readable, and faster than traditional approaches.
⚙️ How It Works
If the prefix/suffix doesn’t exist, the string remains unchanged.
🔍 Introduction
Tired of removing prefixes and suffixes from strings with clunky if statements or replace()?
Python 3.9+ introduced two handy methods:
removeprefix(prefix): Removes the prefix from a string.
removesuffix(suffix): Removes the suffix from a string.
These methods are cleaner, more readable, and faster than traditional approaches.
⚙️ How It Works
If the prefix/suffix doesn’t exist, the string remains unchanged.
💡 Example 1: Remove Prefix
python
Copy code
# Remove prefix from a string
Why it works: The removeprefix() method removes only the beginning of the string, not all occurrences of the prefix.
💡 Example 2: Remove Suffix
python
Copy code
# Remove suffix from a string
print(clean_name) # Output: data_report
Why it works: The removesuffix() method removes the end of the string only, unlike replace(), which works on all occurrences.
💡 Example 3: Combine removeprefix() and removesuffix()
python
Copy code
# Remove both prefix and suffix
Why it works: You can chain removeprefix() and removesuffix() for cleaner, multi-step transformations.
💡 Example 4: Compare Old vs New Approach
python
Copy code
# Old way (clunky)
filename = filename[len('test_'):]
filename = filename[:-len('.txt')]
print(filename) # Output: file
# New way (cleaner)
print(filename) # Output: file
Why it works: The old method is harder to read and more error-prone. The new method is clearer, faster, and simpler.
Why It’s Cool
Cleaner Code: No more awkward if conditions or manual slicing.
Readability: Easy to see the logic — no “what does this slicing do?” confusion.
No Overhead: If the prefix/suffix isn’t found, the string stays the same.
Chained Operations: Chain removeprefix() and removesuffix() for multi-step transformations.
---
EBOOKS:
---
BLOG AND COURSES:
---
SAAS PRODUCTS:
---
SOCIALS:
---
#Python #PythonTips #CodingTricks #CleanCode #LearnPython #DevTips #CodeQuality #SoftwareDevelopment #StringManipulation #Productivity #PythonTricks
Python 3.9+ introduced two handy methods:
removeprefix(prefix): Removes the prefix from a string.
removesuffix(suffix): Removes the suffix from a string.
These methods are cleaner, more readable, and faster than traditional approaches.
⚙️ How It Works
If the prefix/suffix doesn’t exist, the string remains unchanged.
🔍 Introduction
Tired of removing prefixes and suffixes from strings with clunky if statements or replace()?
Python 3.9+ introduced two handy methods:
removeprefix(prefix): Removes the prefix from a string.
removesuffix(suffix): Removes the suffix from a string.
These methods are cleaner, more readable, and faster than traditional approaches.
⚙️ How It Works
If the prefix/suffix doesn’t exist, the string remains unchanged.
💡 Example 1: Remove Prefix
python
Copy code
# Remove prefix from a string
Why it works: The removeprefix() method removes only the beginning of the string, not all occurrences of the prefix.
💡 Example 2: Remove Suffix
python
Copy code
# Remove suffix from a string
print(clean_name) # Output: data_report
Why it works: The removesuffix() method removes the end of the string only, unlike replace(), which works on all occurrences.
💡 Example 3: Combine removeprefix() and removesuffix()
python
Copy code
# Remove both prefix and suffix
Why it works: You can chain removeprefix() and removesuffix() for cleaner, multi-step transformations.
💡 Example 4: Compare Old vs New Approach
python
Copy code
# Old way (clunky)
filename = filename[len('test_'):]
filename = filename[:-len('.txt')]
print(filename) # Output: file
# New way (cleaner)
print(filename) # Output: file
Why it works: The old method is harder to read and more error-prone. The new method is clearer, faster, and simpler.
Why It’s Cool
Cleaner Code: No more awkward if conditions or manual slicing.
Readability: Easy to see the logic — no “what does this slicing do?” confusion.
No Overhead: If the prefix/suffix isn’t found, the string stays the same.
Chained Operations: Chain removeprefix() and removesuffix() for multi-step transformations.
---
EBOOKS:
---
BLOG AND COURSES:
---
SAAS PRODUCTS:
---
SOCIALS:
---
#Python #PythonTips #CodingTricks #CleanCode #LearnPython #DevTips #CodeQuality #SoftwareDevelopment #StringManipulation #Productivity #PythonTricks