filmov
tv
Demystifying Database Transactions with Entity Framework in C#
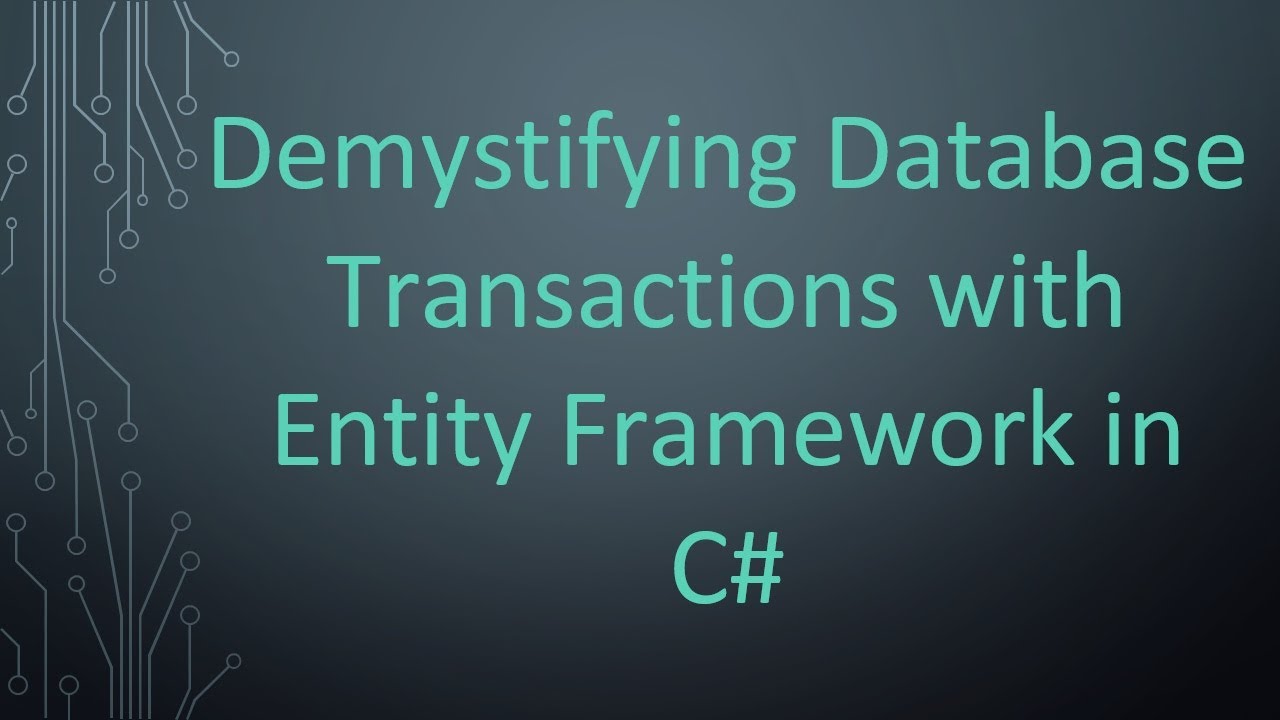
Показать описание
Disclaimer/Disclosure: Some of the content was synthetically produced using various Generative AI (artificial intelligence) tools; so, there may be inaccuracies or misleading information present in the video. Please consider this before relying on the content to make any decisions or take any actions etc. If you still have any concerns, please feel free to write them in a comment. Thank you.
---
Summary: Learn how to handle database transactions effectively using Entity Framework in C#. Understand the concept of transactions, their importance, and how to implement them using Entity Framework for efficient data manipulation.
---
In software development, especially when dealing with databases, ensuring data integrity is paramount. One essential tool for maintaining data consistency is transactions. Transactions allow you to group multiple database operations into a single, atomic unit of work, ensuring that either all operations succeed or none of them are applied.
When working with C and Entity Framework, understanding how to handle transactions is crucial for building robust and reliable applications. Entity Framework (EF) simplifies database interactions by providing an object-relational mapping (ORM) framework, but it's essential to grasp how transactions fit into this paradigm.
What are Transactions?
In the context of databases, a transaction is a series of operations performed on the database that must be executed as a single unit. These operations could include inserting, updating, or deleting records. The ACID properties - Atomicity, Consistency, Isolation, and Durability - define the characteristics of a transaction:
Atomicity: All operations within a transaction must succeed for the transaction to be considered successful. If any operation fails, the entire transaction is rolled back, leaving the database in its original state.
Consistency: A transaction ensures that the database moves from one consistent state to another consistent state. In other words, all integrity constraints and business rules must be enforced during the transaction.
Isolation: Transactions should be isolated from each other, meaning that the intermediate state of a transaction should not be visible to other transactions until it's committed.
Durability: Once a transaction is committed, its changes are persisted in the database and survive system failures.
Implementing Transactions with Entity Framework
Entity Framework simplifies working with transactions by providing built-in mechanisms for managing them. Here's how you can use EF to handle transactions in C:
DbContext Transactions: Entity Framework's DbContext class represents a session with the database and provides methods for managing transactions. You can use the Database.BeginTransaction() method to start a new transaction, execute database operations within the transaction scope, and then either commit or rollback the transaction based on the success of the operations.
TransactionScope: .NET's TransactionScope class allows you to define transactions at the code level. By wrapping your database operations within a TransactionScope block, you ensure that all operations within the scope participate in the same transaction. Entity Framework automatically detects the ambient transaction and enlists its operations accordingly.
Example:
[[See Video to Reveal this Text or Code Snippet]]
Conclusion
Understanding and effectively utilizing transactions is essential for building robust and reliable database-driven applications. With Entity Framework in C, handling transactions becomes more manageable, thanks to its built-in support for transaction management. By adhering to the ACID principles and leveraging Entity Framework's transaction capabilities, developers can ensure data integrity and consistency in their applications.
Remember to carefully design your transactions to minimize the risk of concurrency issues and ensure that your application behaves predictably even under high load scenarios.
By mastering transactions in Entity Framework, developers can build more resilient and scalable applications, providing users with a seamless and reliable experience.
---
Summary: Learn how to handle database transactions effectively using Entity Framework in C#. Understand the concept of transactions, their importance, and how to implement them using Entity Framework for efficient data manipulation.
---
In software development, especially when dealing with databases, ensuring data integrity is paramount. One essential tool for maintaining data consistency is transactions. Transactions allow you to group multiple database operations into a single, atomic unit of work, ensuring that either all operations succeed or none of them are applied.
When working with C and Entity Framework, understanding how to handle transactions is crucial for building robust and reliable applications. Entity Framework (EF) simplifies database interactions by providing an object-relational mapping (ORM) framework, but it's essential to grasp how transactions fit into this paradigm.
What are Transactions?
In the context of databases, a transaction is a series of operations performed on the database that must be executed as a single unit. These operations could include inserting, updating, or deleting records. The ACID properties - Atomicity, Consistency, Isolation, and Durability - define the characteristics of a transaction:
Atomicity: All operations within a transaction must succeed for the transaction to be considered successful. If any operation fails, the entire transaction is rolled back, leaving the database in its original state.
Consistency: A transaction ensures that the database moves from one consistent state to another consistent state. In other words, all integrity constraints and business rules must be enforced during the transaction.
Isolation: Transactions should be isolated from each other, meaning that the intermediate state of a transaction should not be visible to other transactions until it's committed.
Durability: Once a transaction is committed, its changes are persisted in the database and survive system failures.
Implementing Transactions with Entity Framework
Entity Framework simplifies working with transactions by providing built-in mechanisms for managing them. Here's how you can use EF to handle transactions in C:
DbContext Transactions: Entity Framework's DbContext class represents a session with the database and provides methods for managing transactions. You can use the Database.BeginTransaction() method to start a new transaction, execute database operations within the transaction scope, and then either commit or rollback the transaction based on the success of the operations.
TransactionScope: .NET's TransactionScope class allows you to define transactions at the code level. By wrapping your database operations within a TransactionScope block, you ensure that all operations within the scope participate in the same transaction. Entity Framework automatically detects the ambient transaction and enlists its operations accordingly.
Example:
[[See Video to Reveal this Text or Code Snippet]]
Conclusion
Understanding and effectively utilizing transactions is essential for building robust and reliable database-driven applications. With Entity Framework in C, handling transactions becomes more manageable, thanks to its built-in support for transaction management. By adhering to the ACID principles and leveraging Entity Framework's transaction capabilities, developers can ensure data integrity and consistency in their applications.
Remember to carefully design your transactions to minimize the risk of concurrency issues and ensure that your application behaves predictably even under high load scenarios.
By mastering transactions in Entity Framework, developers can build more resilient and scalable applications, providing users with a seamless and reliable experience.