filmov
tv
44 Egg Dropping Problem Memoization
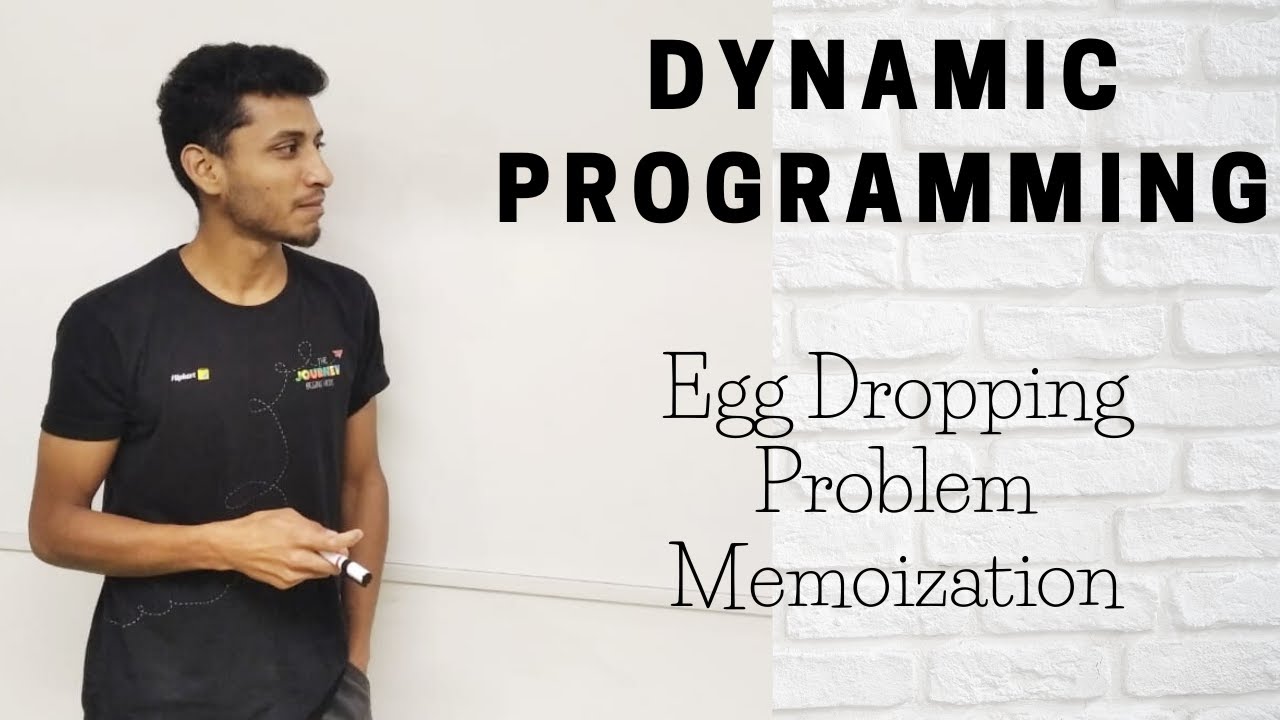
Показать описание
Egg Dropping using Memoization(Dynamic Programming)
Problem statement: You are given N floor and K eggs. You have to minimize the number of times you have to drop the eggs to find the critical floor where critical floor means the floor beyond which eggs start to break. Assumptions of the problem:
If egg breaks at ith floor then it also breaks at all greater floors.
If egg does not break at ith floor then it does not break at all lower floors.
Unbroken egg can be used again.
Note: You have to find minimum trials required to find the critical floor not the critical floor.
Example:
Input:
4
2
Output:
Number of trials when number of eggs is 2 and number of floors is 4: 3
------------------------------------------------------------------------------------------
Here are some of the gears that I use almost everyday:
PS: While having good gears help you perform efficiently, don’t get under the impression that they will make you successful without any hard work.
Problem statement: You are given N floor and K eggs. You have to minimize the number of times you have to drop the eggs to find the critical floor where critical floor means the floor beyond which eggs start to break. Assumptions of the problem:
If egg breaks at ith floor then it also breaks at all greater floors.
If egg does not break at ith floor then it does not break at all lower floors.
Unbroken egg can be used again.
Note: You have to find minimum trials required to find the critical floor not the critical floor.
Example:
Input:
4
2
Output:
Number of trials when number of eggs is 2 and number of floors is 4: 3
------------------------------------------------------------------------------------------
Here are some of the gears that I use almost everyday:
PS: While having good gears help you perform efficiently, don’t get under the impression that they will make you successful without any hard work.
Комментарии