filmov
tv
Resolving SyntaxError: await is only valid in async function in JavaScript
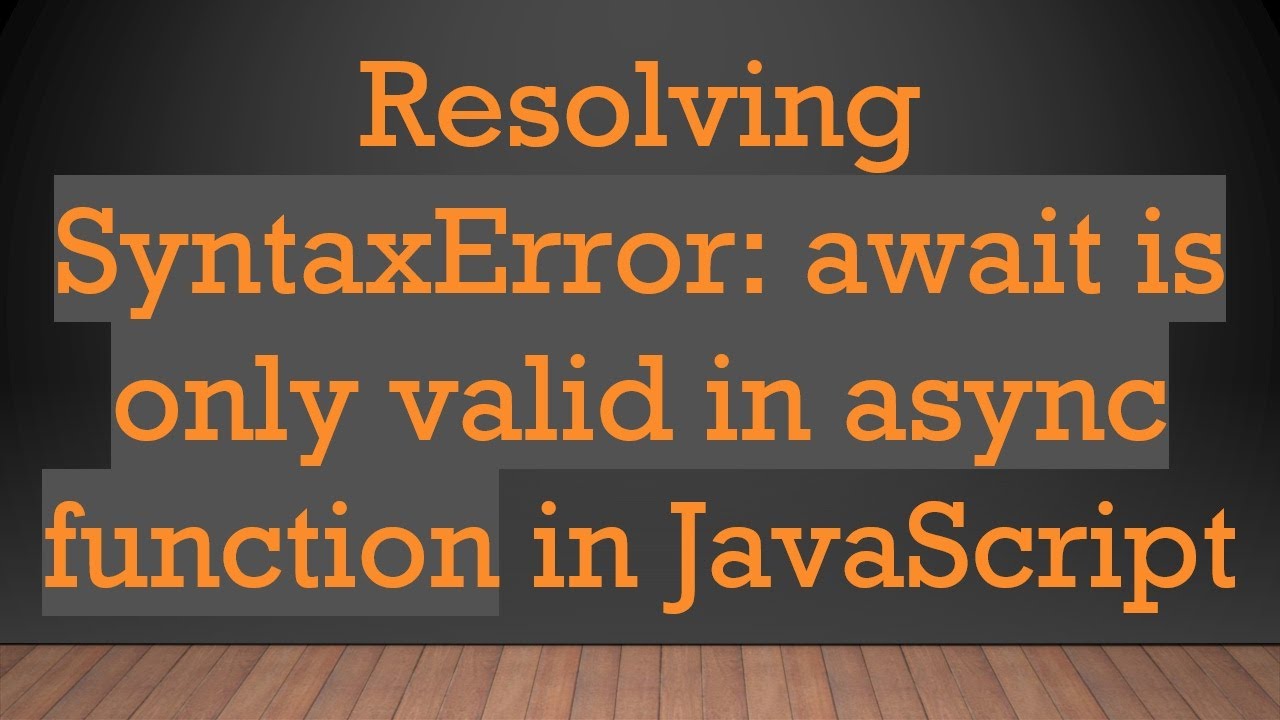
Показать описание
Learn how to properly use async/await in JavaScript and fix common errors like `SyntaxError: await is only valid in async function` with this easy-to-follow guide.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Issues with async/await
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Understanding the SyntaxError with Async/Await in JavaScript
When working with asynchronous operations in JavaScript, the async/await syntax can enhance code readability and functionality. However, many developers encounter confusion and errors when implementing this syntax, particularly the error message: SyntaxError: await is only valid in async function.
In this guide, we will discuss how to properly utilize the async/await syntax and resolve common issues, specifically focusing on the case of improper usage that leads to this syntax error.
The Problem: Understanding the Error Message
The error SyntaxError: await is only valid in async function generally occurs when you try to use the await keyword outside of an async function. This means that JavaScript isn't able to determine that the code requiring the asynchronous operation is set within a proper async context.
Understanding the Code Snippet
Here's a look at the original code that triggered the error:
[[See Video to Reveal this Text or Code Snippet]]
In this code, you can see that await is being used to get the response from the calculate function at the top level of the script. This leads to the syntax error.
The Solution: Making Adjustments
To resolve this issue, we will modify the original code. Instead of awaiting the result directly at the top level, we'll handle the promise resolution using .then(). Here's the fixed code:
[[See Video to Reveal this Text or Code Snippet]]
Breakdown of the Changes Made
Removal of the async Keyword:
We removed the async keyword from the calculate function to prevent confusion since we're handling the promise directly.
Using .then() for Handling Results:
Instead of await, we use .then() to handle the returned promise from the calculate function. This ensures that we are working within a resolved promise context.
Logging the Response:
Conclusion
By carefully organizing your async functions and ensuring that you only use the await keyword within async functions, you can eliminate the confusion that often arises with promise handling in JavaScript. Remember, whenever you see the error SyntaxError: await is only valid in async function, check your function scopes and structure.
Feel free to experiment with your code while keeping these adjustments in mind, and enjoy the advantages that async/await provides when done correctly!
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Issues with async/await
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Understanding the SyntaxError with Async/Await in JavaScript
When working with asynchronous operations in JavaScript, the async/await syntax can enhance code readability and functionality. However, many developers encounter confusion and errors when implementing this syntax, particularly the error message: SyntaxError: await is only valid in async function.
In this guide, we will discuss how to properly utilize the async/await syntax and resolve common issues, specifically focusing on the case of improper usage that leads to this syntax error.
The Problem: Understanding the Error Message
The error SyntaxError: await is only valid in async function generally occurs when you try to use the await keyword outside of an async function. This means that JavaScript isn't able to determine that the code requiring the asynchronous operation is set within a proper async context.
Understanding the Code Snippet
Here's a look at the original code that triggered the error:
[[See Video to Reveal this Text or Code Snippet]]
In this code, you can see that await is being used to get the response from the calculate function at the top level of the script. This leads to the syntax error.
The Solution: Making Adjustments
To resolve this issue, we will modify the original code. Instead of awaiting the result directly at the top level, we'll handle the promise resolution using .then(). Here's the fixed code:
[[See Video to Reveal this Text or Code Snippet]]
Breakdown of the Changes Made
Removal of the async Keyword:
We removed the async keyword from the calculate function to prevent confusion since we're handling the promise directly.
Using .then() for Handling Results:
Instead of await, we use .then() to handle the returned promise from the calculate function. This ensures that we are working within a resolved promise context.
Logging the Response:
Conclusion
By carefully organizing your async functions and ensuring that you only use the await keyword within async functions, you can eliminate the confusion that often arises with promise handling in JavaScript. Remember, whenever you see the error SyntaxError: await is only valid in async function, check your function scopes and structure.
Feel free to experiment with your code while keeping these adjustments in mind, and enjoy the advantages that async/await provides when done correctly!