filmov
tv
The coding conventions I use for C# and why I use them
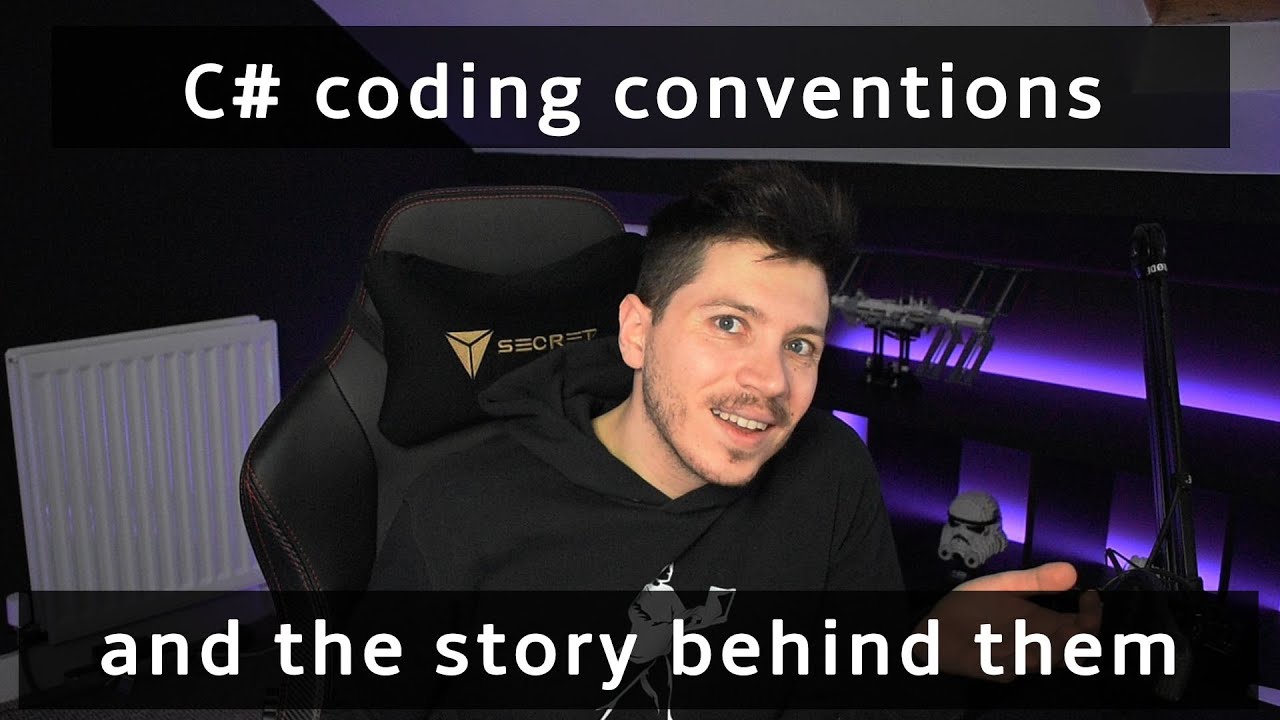
Показать описание
Hello everybody I'm Nick and in this video I wanna talk about the coding conventions I use when I'm writing C#. This is a heavily subjective and opinionated topic, so the conventions themselves don't matter. What matters, no matter the convention, is the story behind it.
Timestamps
Intro - 0:00
Top level folders and projects - 1:13
Unit test naming - 2:12
Folder naming - 3:05
Class naming and conventions - 5:20
Var vs explicit type - 7:04
Class ordering - 9:00
General stuff - 11:21
Don't forget to comment, like and subscribe :)
Social Media:
#dotnet #csharp
The coding conventions I use for C# and why I use them
MEDICAL CODING ICD-10-CM GUIDELINES LESSON - 1.A - Coder explanation and examples for 2021
Best Coding Practices and Code Conventions: Why Are They Important?
2024 ICD-10-CM Official Coding Guidelines
Coding Conventions
How Senior Programmers ACTUALLY Write Code
Naming Things in Code
'Why do you use C-Style Naming Conventions?'
Java Coding Standards and Best Practices:
how NASA writes space-proof code
The WORST Coding Standards EVER 💀 #developer #softwaredeveloper #code #technology #coding
ICD-10-CM Coding Conventions
HOW TO STUDY THE ICD-10-CM CODING GUIDELINES 2024
The coding conventions that makes our lives easier — Çağıl Uluşahin Sönmez
How principled coders outperform the competition
Coding Style - The ONLY video you NEED
Python Case Types and Naming Conventions
Python Coding Conventions You Really Should Follow
5 C# Naming Conventions I Wish Everyone Followed
ICD-10 Sequencing Rules Explained!!!
how Google writes gorgeous C++
Why You Shouldn't Nest Your Code
Coding Standards and Naming Conventions
JavaScript Coding Conventions
Комментарии