filmov
tv
Cython: Blend the Best of Python and C++ | SciPy 2015 Tutorial | Kurt Smith
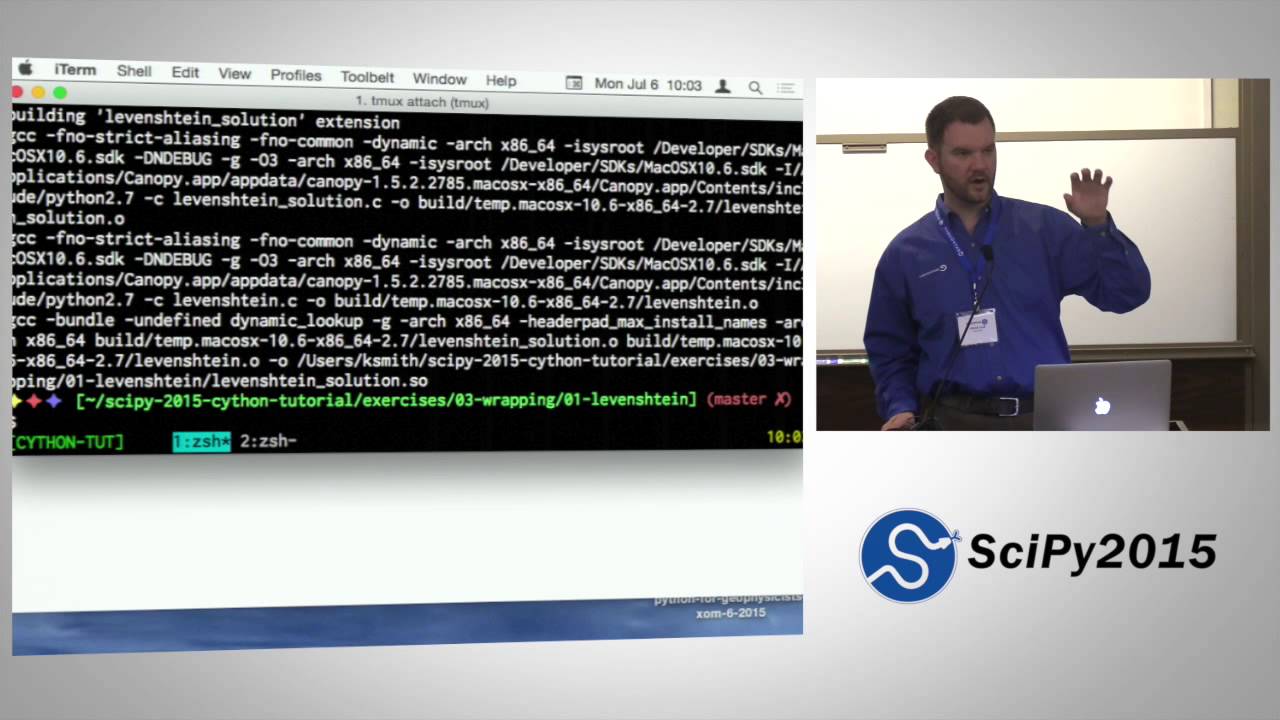
Показать описание
Cython: Blend the Best of Python and C++ | SciPy 2015 Tutorial | Kurt Smith
Stefan Behnel - Cython to speed up your Python code
Speed Up Your Code With Cython
How to use Cython to speed up Python
Cython 3.0: Compiling Python to C, the next generation
PyCon.DE 2018: Cython to speed up your Python code - Stefan Behnel
Matti Picus - CFFI, Ctypes, Cython The Good, The Bad and The Ugly - Pycon Israel 2017
Talk - Maryanne Wachter: Will it Blend? Writing A Custom Constraint Solver for Blender with Cython
#decompile #cython
Cython Tutorial - Bridging between Python and C/C++ for performance gains
Bridging between Static and Dynamic Typing in Cython- P7 | Cython for Python 🔥
Speeding up Scientific Python code using Cython | EuroSciPy 2014 | Stéfan van der Walt
Stefan Behnel - Get up to speed with Cython 3.0
How to C Function behaves inside the Cython code? -P13|Cython for Python| Python Tutorials
Grant Jenks - Cython for All with GitHub Actions
Simmi Mourya - Scientific computing using Cython: Best of both Worlds!
Stefan Behnel - Fast Async Code with Cython and AsyncIO
How to convert Python to Cython (and Speed Up 100X)
[IT] Cython Tutorial - Introduzione 1/2
Cython: pointers and strings
Speeding Up Python Data Analysis Using Cython
Creating Cython libraries for redistribution in a Python package
Cython for Python | Ultimate Guide - P1 | Python tutorials
MUSEN Kolloquium - Stefan Behnel - Cython for Python-Users
Комментарии