filmov
tv
Java Design Patterns Explained in 20 minutes
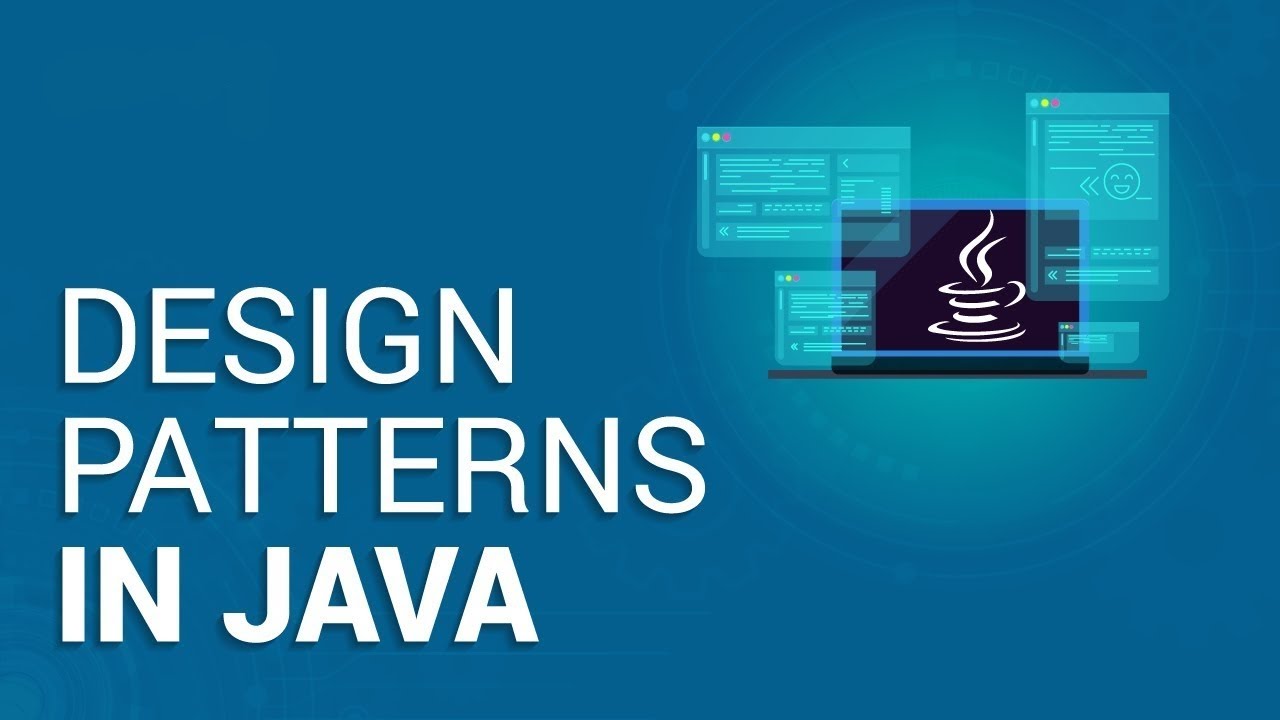
Показать описание
This video explores the software design patterns Command, Factories, Iterator, Memento, Observer, and MVC in Java, with practical examples and detailed explanations.
Command Pattern:
Encapsulates a request as an object, allowing clients to be parameterized with different requests, queue or log requests, and support undoable operations.
Participants:
Command (interface)
ConcreteCommand (links Receiver to the action)
Client (creates ConcreteCommand)
Invoker (executes the command)
Receiver (performs the action)
Example: Managing operations of a bank account (deposit, withdrawal) with history and undo functionality.
Pros:
Separates invoker from receiver.
Allows adding commands without modifying the client.
Supports undo/redo, deferred execution, and command composition.
Cons:
Adds complexity due to the extra layer between invoker and receiver.
Factory Patterns:
Abstract the creation of objects, providing flexibility and code reuse.
Difference between creation methods and factories:
All factories use creation methods, but not all creation methods are factories.
Types: Simple Factory, Factory Method, Abstract Factory.
Simple Factory: Centralizes the creation of object variants in a class with a method that selects the object type to return based on parameters.
Example: Creating different types of average calculation strategies.
Factory Method: Allows alternative factories for different product families.
Example: Creating different citation styles (IEEE, APA).
Pros: Promotes low coupling, single responsibility, and easy addition of new products.
Cons: May increase code complexity due to interfaces and subclasses.
Iterator Pattern:
Provides a standardized way to sequentially traverse elements of a container.
Participants:
Iterator (interface)
ConcreteIterator (maintains iteration position)
Collection (interface to create Iterator)
ConcreteCollection (creates the ConcreteIterator)
Example: Iterating through a stack without destroying its content.
Java Implementation: Iterable and Iterator interfaces.
Memento Pattern:
Allows saving and restoring an object’s internal state without violating encapsulation.
Participants:
Originator (object whose state is captured)
Memento (stores the state)
Caretaker (stores Mementos)
Example: Managing states of a bank account and undoing transactions.
Pros: Captures snapshots of the state without breaking encapsulation.
Cons: Can consume a lot of memory if many Mementos are created.
Observer Pattern:
Automatically notifies dependent objects (observers) when an object (subject) changes its state.
Participants:
Subject (interface)
ConcreteSubject (concrete subject)
Observer (interface)
ConcreteObserver (concrete observer)
Java Implementation: Observable and Observer interfaces.
Example: Separating application logic from user interface in a shopping cart.
MVC Pattern (Model-View-Controller):
Separates business logic (model), user interface (view), and control flow (controller).
Model: Manages data and operations, without knowledge of the interface.
View: Displays model data and delegates actions to the controller.
Controller: Synchronizes user actions with the model and view.
Applicability: Web and mobile development, present in frameworks like AngularJS, React, Spring.
Example: Implementing a shopping cart with MVC in JavaFX.
Timestamps:
0:00 - Introduction
0:53 - History behind Design Patterns
1:23 - Observer Pattern
3:15 - Iterator Pattern
4:18 - Iterator with Stack
5:05 - MVC Pattern
6:47 - Command Pattern
8:00 - Example with a real project
11:24 - Invoker
12:02 - Memento Pattern
13:11 - Strategy Pattern
15:11 - Factory Pattern
15:21 - Simple Factory
15:46 - Factory Method
16:57 - Abstract Factory
19:07 - Iterator with Composite Pattern
19:49 - Conclusion
Links to documentation used in this video:
Command Pattern:
Encapsulates a request as an object, allowing clients to be parameterized with different requests, queue or log requests, and support undoable operations.
Participants:
Command (interface)
ConcreteCommand (links Receiver to the action)
Client (creates ConcreteCommand)
Invoker (executes the command)
Receiver (performs the action)
Example: Managing operations of a bank account (deposit, withdrawal) with history and undo functionality.
Pros:
Separates invoker from receiver.
Allows adding commands without modifying the client.
Supports undo/redo, deferred execution, and command composition.
Cons:
Adds complexity due to the extra layer between invoker and receiver.
Factory Patterns:
Abstract the creation of objects, providing flexibility and code reuse.
Difference between creation methods and factories:
All factories use creation methods, but not all creation methods are factories.
Types: Simple Factory, Factory Method, Abstract Factory.
Simple Factory: Centralizes the creation of object variants in a class with a method that selects the object type to return based on parameters.
Example: Creating different types of average calculation strategies.
Factory Method: Allows alternative factories for different product families.
Example: Creating different citation styles (IEEE, APA).
Pros: Promotes low coupling, single responsibility, and easy addition of new products.
Cons: May increase code complexity due to interfaces and subclasses.
Iterator Pattern:
Provides a standardized way to sequentially traverse elements of a container.
Participants:
Iterator (interface)
ConcreteIterator (maintains iteration position)
Collection (interface to create Iterator)
ConcreteCollection (creates the ConcreteIterator)
Example: Iterating through a stack without destroying its content.
Java Implementation: Iterable and Iterator interfaces.
Memento Pattern:
Allows saving and restoring an object’s internal state without violating encapsulation.
Participants:
Originator (object whose state is captured)
Memento (stores the state)
Caretaker (stores Mementos)
Example: Managing states of a bank account and undoing transactions.
Pros: Captures snapshots of the state without breaking encapsulation.
Cons: Can consume a lot of memory if many Mementos are created.
Observer Pattern:
Automatically notifies dependent objects (observers) when an object (subject) changes its state.
Participants:
Subject (interface)
ConcreteSubject (concrete subject)
Observer (interface)
ConcreteObserver (concrete observer)
Java Implementation: Observable and Observer interfaces.
Example: Separating application logic from user interface in a shopping cart.
MVC Pattern (Model-View-Controller):
Separates business logic (model), user interface (view), and control flow (controller).
Model: Manages data and operations, without knowledge of the interface.
View: Displays model data and delegates actions to the controller.
Controller: Synchronizes user actions with the model and view.
Applicability: Web and mobile development, present in frameworks like AngularJS, React, Spring.
Example: Implementing a shopping cart with MVC in JavaFX.
Timestamps:
0:00 - Introduction
0:53 - History behind Design Patterns
1:23 - Observer Pattern
3:15 - Iterator Pattern
4:18 - Iterator with Stack
5:05 - MVC Pattern
6:47 - Command Pattern
8:00 - Example with a real project
11:24 - Invoker
12:02 - Memento Pattern
13:11 - Strategy Pattern
15:11 - Factory Pattern
15:21 - Simple Factory
15:46 - Factory Method
16:57 - Abstract Factory
19:07 - Iterator with Composite Pattern
19:49 - Conclusion
Links to documentation used in this video:
Комментарии