filmov
tv
Coding Interview Question and Answer: Longest Consecutive Characters
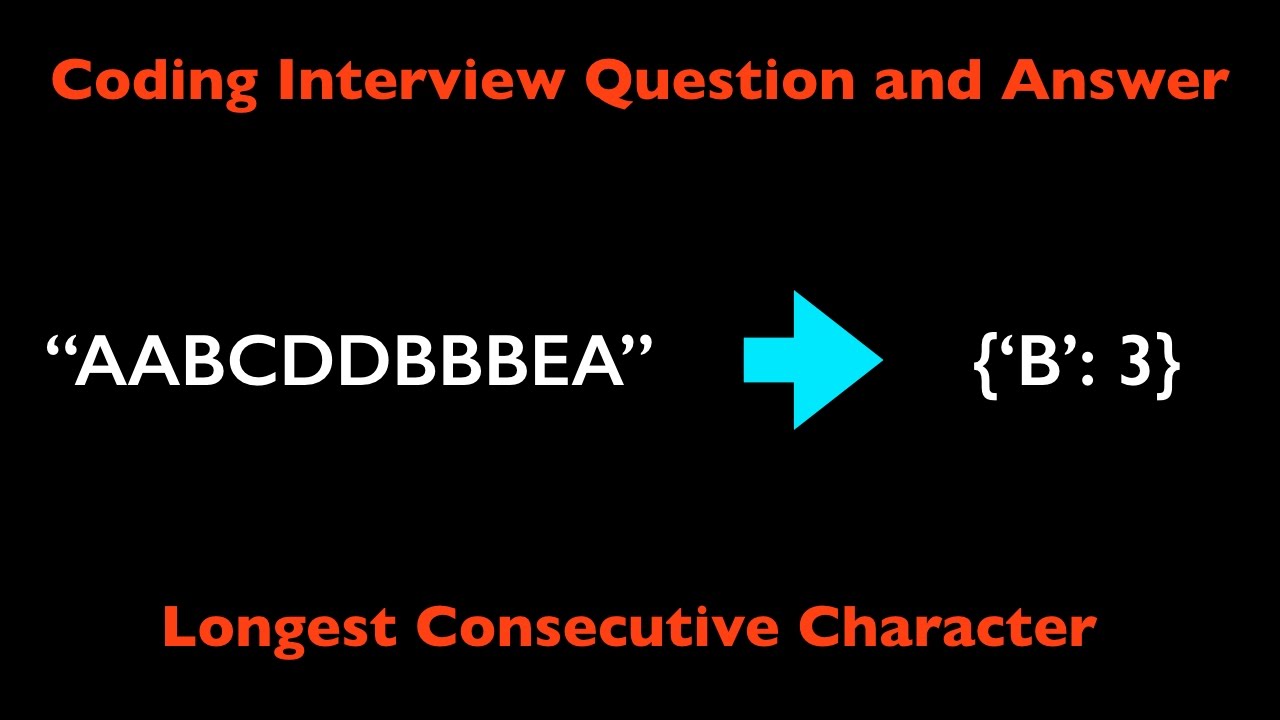
Показать описание
Given a string, find the longest subsequence consisting of a single character. Example: longest("ABAACDDDBBA") should return {'D': 3}.
(You'll get a 30-day trial with the link)
(You'll get a 30-day trial with the link)
Coding Interview Questions And Answers | Programming Interview Questions And Answers | Simplilearn
Top 6 Coding Interview Concepts (Data Structures & Algorithms)
How to NOT Fail a Technical Interview
Google Coding Interview Question and Answer #1: First Recurring Character
Most Asked Coding Interview Question (Don't Skip !!😮) #shorts
10 Common Coding Interview Problems - Solved!
Whiteboard Coding Interviews: 6 Steps to Solve Any Problem
Top 40 C Programming Interview Questions | C Programming Interview Questions And Answers|Simplilearn
python interview questions and answers __ python interview __ python interview question
Solving JavaScript's Most NOTORIOUS Interview Question
5 Problem Solving Tips for Cracking Coding Interview Questions
Nailing your Java Interview
How to use Cracking The Coding Interview Effectively
Cracking the Behavioral Interview for Software Developers
Medium Google Coding Interview With Ben Awad
The *secret* to coding Interviews 🤫
ANYONE can Crack Coding Interviews by Doing THIS
Python Coding Interview Practice - Difficulty: Hard
How to: Work at Google — Example Coding/Engineering Interview
Why should we hire you? Interview Question #6
How To Answer The HARDEST Job Interview Questions
Coding Interview | Software Engineer @ Bloomberg (Part 1)
Solving Coding Interview Questions in Python on LeetCode (easy & medium problems)
How to say I don't know in Interviews | Interview Qs #4
Комментарии