filmov
tv
Google Coding Interview Question and Answer #1: First Recurring Character
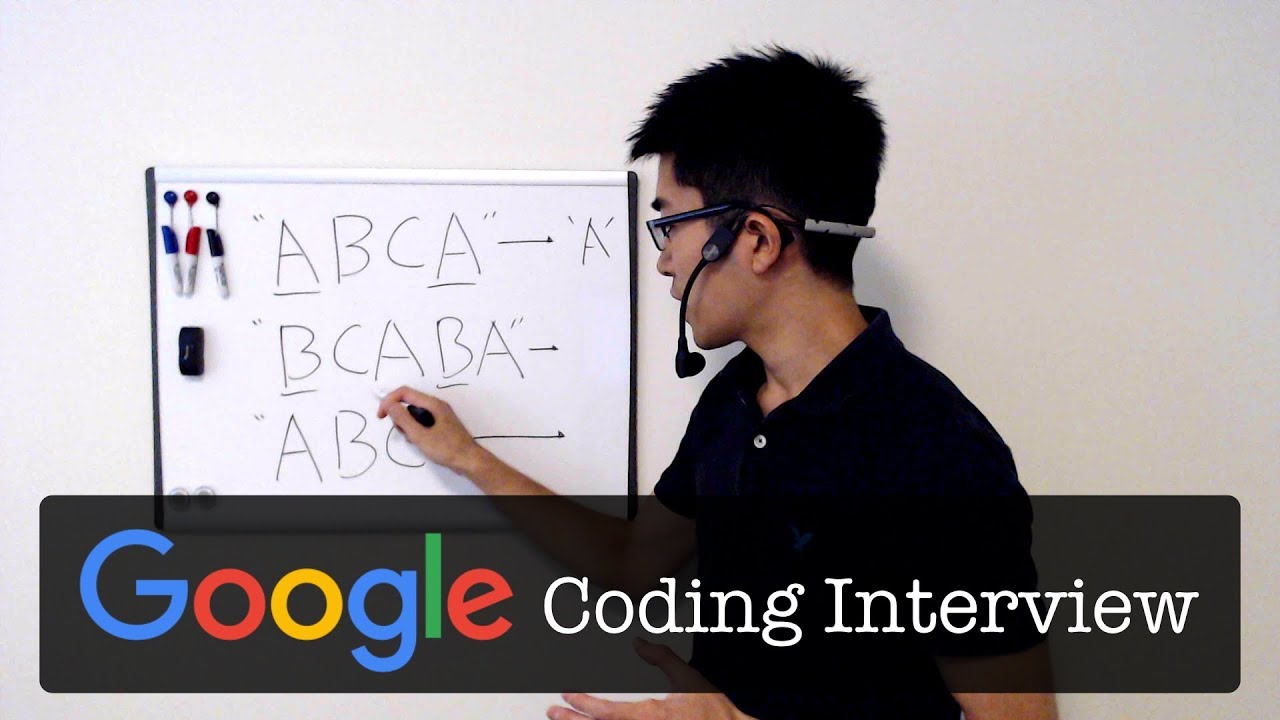
Показать описание
Find the first recurring character in the given string!
(You'll get a discount through the above link.)
(You'll get a discount through the above link.)
How to: Work at Google — Example Coding/Engineering Interview
Google Coding Interview Question and Answer #1: First Recurring Character
Google Coding Interview With A Normal Software Engineer
Google Coding Interview With A High School Student
How I cracked Google Interview after 500+ rejections
Easy Google Coding Interview With Ben Awad
How to: Work at Google — Example Coding/Engineering Interview
Medium Google Coding Interview With Ben Awad
Climbing Stairs: 70 - google, meta interview question
Google Coding Interview With A Facebook Software Engineer
How I Failed the Google Coding Interview (and lessons I learned)
How I Cracked the Google Coding Interview (from unemployment)
Google Interview Experience | SDE-III | L4
Google Coding Interview With A College Student
preparing for google's machine learning interview
Trapping Rain Water - Google Interview Question - Leetcode 42
Google Coding Interview With A Competitive Programmer
I gave 127 interviews. Top 5 Algorithms they asked me.
Google Software Engineering Interview Guide
Top 5 Coding Interview MISTAKES (from a Google Engineer)
I solved 541 Leetcode problems. But you need only 150.
My 3 Weeks GOOGLE Prep and NOTES
How to NOT Fail a Technical Interview
Most Asked Coding Interview Question (Don't Skip !!😮) #shorts
Комментарии