filmov
tv
Creating a Dynamic appendChild Function in JavaScript: Simplify Your Code!
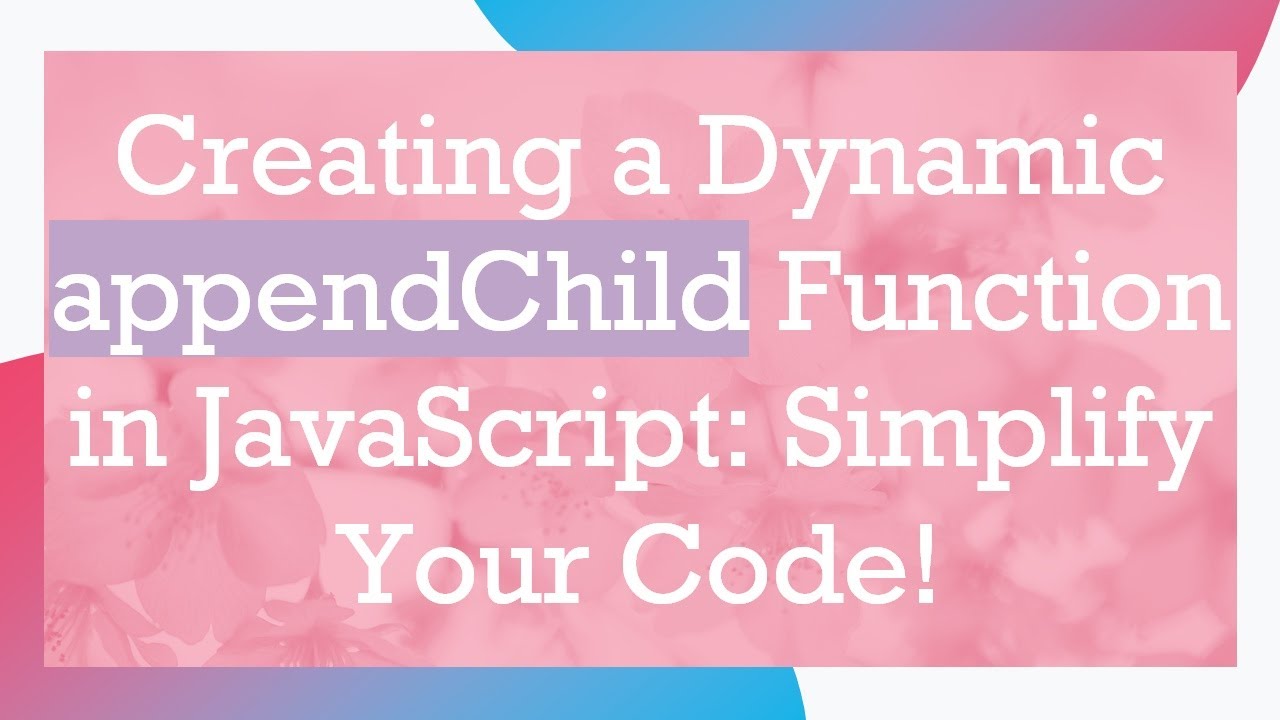
Показать описание
Learn how to create a dynamic `appendChild` function in JavaScript that simplifies your code, enables you to create multiple elements, and allows child elements to have their own children.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: appendChild function that could be called multiple times and make multiple elements with content in it
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Creating a Dynamic appendChild Function in JavaScript: Simplify Your Code!
JavaScript is a powerful tool for building dynamic web applications, but as your code grows, it can become unwieldy and hard to read. One common pain point is the repetitive nature of creating and appending DOM elements with the appendChild method. In this guide, we will discuss how to create a reusable function that streamlines the process of element creation and makes your code cleaner and more manageable.
The Problem
When building web applications, developers often need to create multiple DOM elements and append them to parents. This can lead to messy and repetitive code, as seen in the following example:
[[See Video to Reveal this Text or Code Snippet]]
The code above is functional, but it can be difficult to read and maintain, especially when you need to create several elements, or when those elements themselves need children. How can we simplify this process?
The Solution: A createElement Function
To enhance our code and make it more readable, we can create a createElement function. This function will allow us to define the type of element we want, add properties, and append children easily.
The createElement Function Structure
Here's how you can define the createElement function:
[[See Video to Reveal this Text or Code Snippet]]
How It Works
Element Creation: The function takes in a type (like "div" or "p"), properties (optional), and any number of children.
Children: We loop through the children array:
If a child is a string, we create a text node.
If it’s another element, we append it directly using appendChild.
Return the Element: Finally, we return the constructed element with its properties and children attached.
Usage Example
Here's how to use the createElement function to build a structured element:
[[See Video to Reveal this Text or Code Snippet]]
This piece of code renders to the following HTML structure:
[[See Video to Reveal this Text or Code Snippet]]
Benefits of Using createElement
Readability: Your code becomes cleaner and easier to read.
Reusability: You can create various elements quickly without repeating yourself.
Simplicity: The function manages both properties and children, making it versatile for different usage scenarios.
Conclusion
By implementing the createElement function, you will improve the structure and readability of your JavaScript code significantly. This approach not only simplifies the creation of elements but also allows for nesting, making it easier to manage complex DOM structures. Try incorporating this solution into your next project and experience the difference it makes!
Ready to streamline your JavaScript code? Start using the createElement function today and watch your productivity soar!
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: appendChild function that could be called multiple times and make multiple elements with content in it
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Creating a Dynamic appendChild Function in JavaScript: Simplify Your Code!
JavaScript is a powerful tool for building dynamic web applications, but as your code grows, it can become unwieldy and hard to read. One common pain point is the repetitive nature of creating and appending DOM elements with the appendChild method. In this guide, we will discuss how to create a reusable function that streamlines the process of element creation and makes your code cleaner and more manageable.
The Problem
When building web applications, developers often need to create multiple DOM elements and append them to parents. This can lead to messy and repetitive code, as seen in the following example:
[[See Video to Reveal this Text or Code Snippet]]
The code above is functional, but it can be difficult to read and maintain, especially when you need to create several elements, or when those elements themselves need children. How can we simplify this process?
The Solution: A createElement Function
To enhance our code and make it more readable, we can create a createElement function. This function will allow us to define the type of element we want, add properties, and append children easily.
The createElement Function Structure
Here's how you can define the createElement function:
[[See Video to Reveal this Text or Code Snippet]]
How It Works
Element Creation: The function takes in a type (like "div" or "p"), properties (optional), and any number of children.
Children: We loop through the children array:
If a child is a string, we create a text node.
If it’s another element, we append it directly using appendChild.
Return the Element: Finally, we return the constructed element with its properties and children attached.
Usage Example
Here's how to use the createElement function to build a structured element:
[[See Video to Reveal this Text or Code Snippet]]
This piece of code renders to the following HTML structure:
[[See Video to Reveal this Text or Code Snippet]]
Benefits of Using createElement
Readability: Your code becomes cleaner and easier to read.
Reusability: You can create various elements quickly without repeating yourself.
Simplicity: The function manages both properties and children, making it versatile for different usage scenarios.
Conclusion
By implementing the createElement function, you will improve the structure and readability of your JavaScript code significantly. This approach not only simplifies the creation of elements but also allows for nesting, making it easier to manage complex DOM structures. Try incorporating this solution into your next project and experience the difference it makes!
Ready to streamline your JavaScript code? Start using the createElement function today and watch your productivity soar!