filmov
tv
Resolving the Main Function Issue: Why Your Python Code Isn't Returning the DataFrame
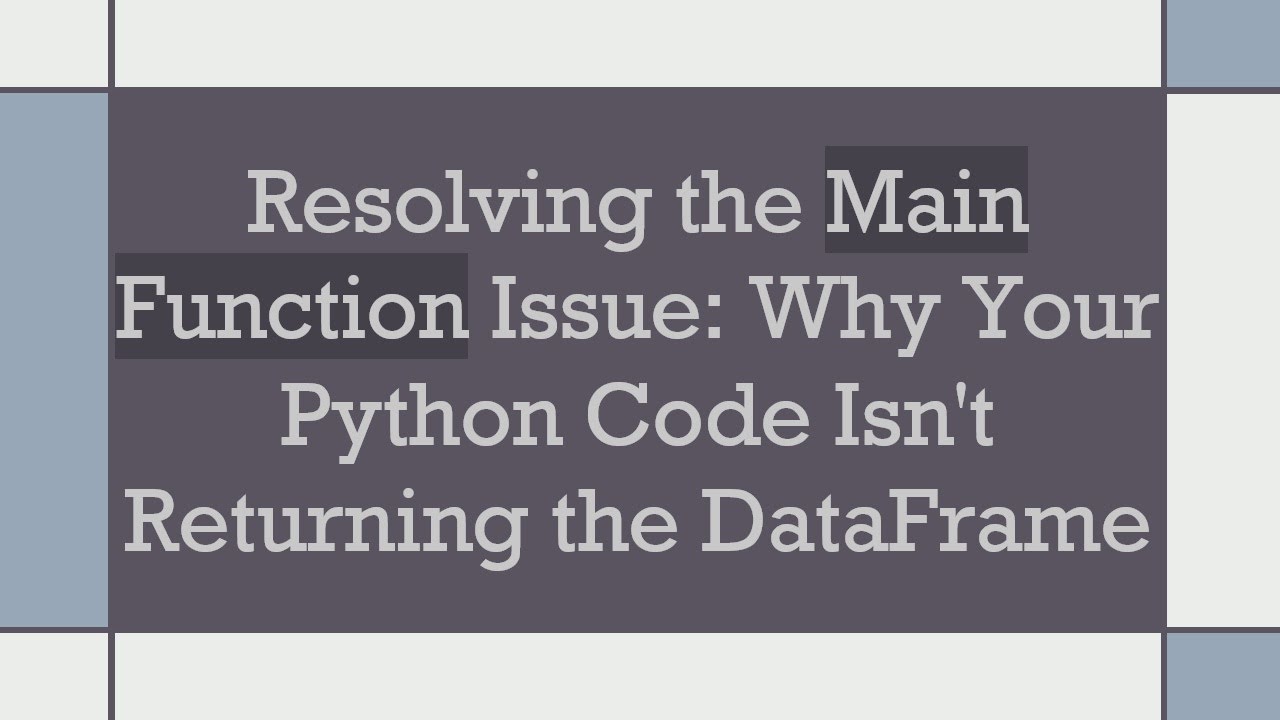
Показать описание
Discover how to fix the common problem of functions in Python that don't return DataFrames, and learn best practices for variable scope and returning variables from functions.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: python main function not returning the dataframe
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Understanding the Issue: Python's Main Function and DataFrames
In Python, particularly when working within a Jupyter notebook, you may encounter a frustrating issue where variables defined within a function aren't accessible outside that function. A common scenario arises when you have a main function that isn't returning the DataFrame you expect to work with later in your code. This guide will clarify why this happens and how you can address it effectively.
The Problem in Detail
You may find yourself in a situation similar to this:
[[See Video to Reveal this Text or Code Snippet]]
When you run the above code, you might encounter a NameError stating that exceldf is not defined. The reason? exceldf is defined locally within the main() function and is not accessible outside of it. So, let's break this down and see how we can solve the problem.
The Solution: Global Variables or Return Values
To fix the issue, you have two choices: declare exceldf as a global variable or return it from the main() function. Let’s explore both methods.
Method 1: Using Global Variables
Declaring exceldf as a global variable allows it to be accessed outside the function. Here’s how you implement this:
[[See Video to Reveal this Text or Code Snippet]]
How It Works:
Global Declaration: By declaring exceldf as global, you allow it to be used anywhere in the module after it’s been defined in main().
Method 2: Returning Values from Functions
Alternatively, you can return the exceldf DataFrame from the main() function. This is often seen as a cleaner approach as it maintains function encapsulation and avoids unexpected side-effects from global state:
[[See Video to Reveal this Text or Code Snippet]]
How It Works:
Noting the Return Value: By using return, you capture whatever exceldf holds after main() runs and it is then accessible in the broader context of your notebook or script.
Conclusion
When working in Python, particularly in a Jupyter notebook environment, understanding variable scope is vital to avoid issues like NameError. You can either make use of the global keyword to declare the variable outside of its function or simply return the value from the function. Always consider encapsulation and avoid using global variables unless necessary, as this can lead to harder-to-maintain code.
Navigating these concepts might seem tricky at first, but with practice, you’ll become adept at managing data within your functions. Happy coding!
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: python main function not returning the dataframe
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Understanding the Issue: Python's Main Function and DataFrames
In Python, particularly when working within a Jupyter notebook, you may encounter a frustrating issue where variables defined within a function aren't accessible outside that function. A common scenario arises when you have a main function that isn't returning the DataFrame you expect to work with later in your code. This guide will clarify why this happens and how you can address it effectively.
The Problem in Detail
You may find yourself in a situation similar to this:
[[See Video to Reveal this Text or Code Snippet]]
When you run the above code, you might encounter a NameError stating that exceldf is not defined. The reason? exceldf is defined locally within the main() function and is not accessible outside of it. So, let's break this down and see how we can solve the problem.
The Solution: Global Variables or Return Values
To fix the issue, you have two choices: declare exceldf as a global variable or return it from the main() function. Let’s explore both methods.
Method 1: Using Global Variables
Declaring exceldf as a global variable allows it to be accessed outside the function. Here’s how you implement this:
[[See Video to Reveal this Text or Code Snippet]]
How It Works:
Global Declaration: By declaring exceldf as global, you allow it to be used anywhere in the module after it’s been defined in main().
Method 2: Returning Values from Functions
Alternatively, you can return the exceldf DataFrame from the main() function. This is often seen as a cleaner approach as it maintains function encapsulation and avoids unexpected side-effects from global state:
[[See Video to Reveal this Text or Code Snippet]]
How It Works:
Noting the Return Value: By using return, you capture whatever exceldf holds after main() runs and it is then accessible in the broader context of your notebook or script.
Conclusion
When working in Python, particularly in a Jupyter notebook environment, understanding variable scope is vital to avoid issues like NameError. You can either make use of the global keyword to declare the variable outside of its function or simply return the value from the function. Always consider encapsulation and avoid using global variables unless necessary, as this can lead to harder-to-maintain code.
Navigating these concepts might seem tricky at first, but with practice, you’ll become adept at managing data within your functions. Happy coding!