filmov
tv
Python Exercise – Password Generator Using Built-in Modules
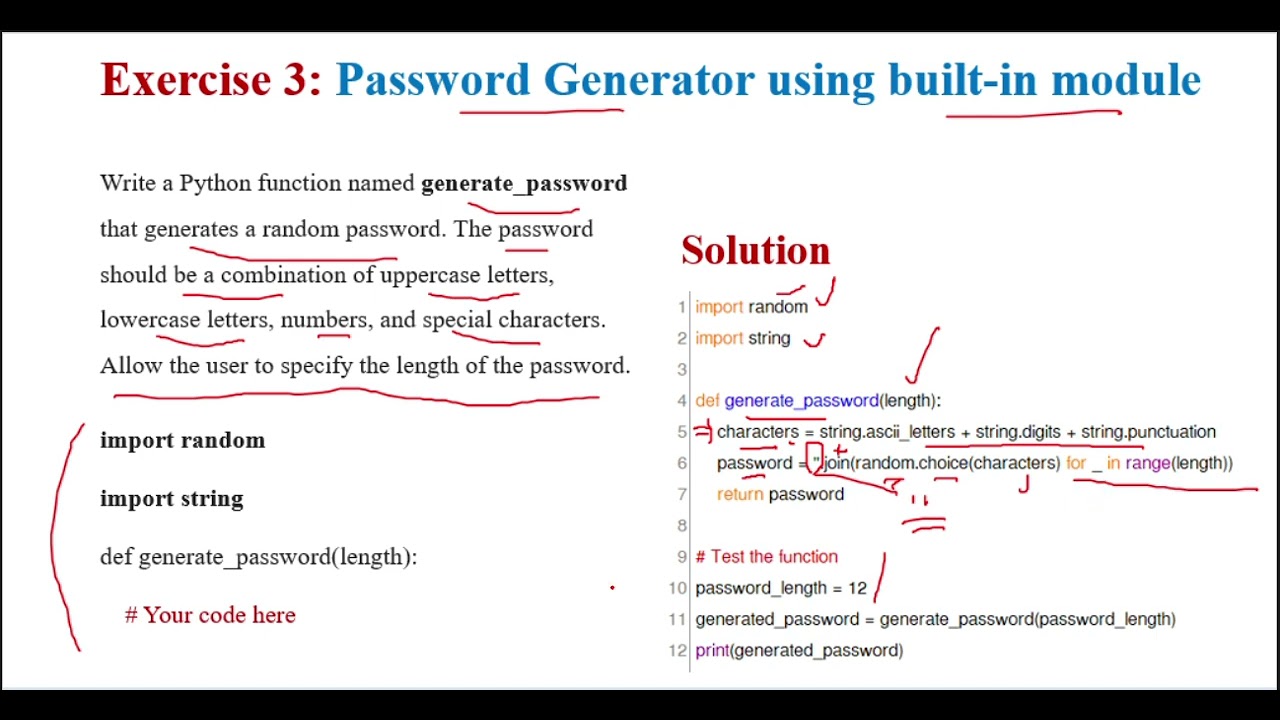
Показать описание
🎥 Python Password Generator: Secure Random Passwords Using Built-in Modules
📜 Video Description:
In this video, we build a secure password generator in Python using built-in modules like random and string. This function generates random passwords with uppercase letters, lowercase letters, numbers, and special characters.
📌 What You’ll Learn:
✅ How to generate random passwords in Python
✅ How to use the random and string modules
✅ How to allow users to specify password length
✅ How to ensure password security using randomness
🔹 Exercise: Generate a Secure Random Password
📌 Problem Statement:
- Write a Python function named generate_password() that creates a random password containing:
✔ Uppercase & lowercase letters
✔ Numbers (digits 0-9)
✔ Special characters
- The user should be able to specify the length of the password.
✅ Solution Code:
import random
import string
def generate_password(length):
return password
# Test the function
password_length = 12
generated_password = generate_password(password_length)
print(generated_password)
📌 Example Output:
- Each time you run the function, it generates a new, random password.
🚀 Why This Exercise Is Important?
✅ Strengthens Security – Ensures passwords are random and difficult to guess.
✅ Uses Built-in Modules – Demonstrates the power of random and string in Python.
✅ Enhances Coding Skills – Learn how to manipulate strings and use loops effectively.
🎯 What You’ll Learn in This Video:
🔹 How to generate random strings and passwords.
🔹 How to customize password length based on user input.
🔹 How to apply randomness securely in Python.
💡 Pro Tip: Always use secure random generators like Python’s secrets module for cryptographic-level security.
📢 Don’t Forget to Like 👍, Share 🔄, and Subscribe 🔔
If you found this password generator tutorial helpful, subscribe for more Python exercises, coding challenges, and real-world applications! 🚀
🔔 Stay updated with new Python tutorials every week!
📜 Video Description:
In this video, we build a secure password generator in Python using built-in modules like random and string. This function generates random passwords with uppercase letters, lowercase letters, numbers, and special characters.
📌 What You’ll Learn:
✅ How to generate random passwords in Python
✅ How to use the random and string modules
✅ How to allow users to specify password length
✅ How to ensure password security using randomness
🔹 Exercise: Generate a Secure Random Password
📌 Problem Statement:
- Write a Python function named generate_password() that creates a random password containing:
✔ Uppercase & lowercase letters
✔ Numbers (digits 0-9)
✔ Special characters
- The user should be able to specify the length of the password.
✅ Solution Code:
import random
import string
def generate_password(length):
return password
# Test the function
password_length = 12
generated_password = generate_password(password_length)
print(generated_password)
📌 Example Output:
- Each time you run the function, it generates a new, random password.
🚀 Why This Exercise Is Important?
✅ Strengthens Security – Ensures passwords are random and difficult to guess.
✅ Uses Built-in Modules – Demonstrates the power of random and string in Python.
✅ Enhances Coding Skills – Learn how to manipulate strings and use loops effectively.
🎯 What You’ll Learn in This Video:
🔹 How to generate random strings and passwords.
🔹 How to customize password length based on user input.
🔹 How to apply randomness securely in Python.
💡 Pro Tip: Always use secure random generators like Python’s secrets module for cryptographic-level security.
📢 Don’t Forget to Like 👍, Share 🔄, and Subscribe 🔔
If you found this password generator tutorial helpful, subscribe for more Python exercises, coding challenges, and real-world applications! 🚀
🔔 Stay updated with new Python tutorials every week!