filmov
tv
How to Create a Dynamic Guest List in JavaScript: A Beginner's Guide to Using If Statements
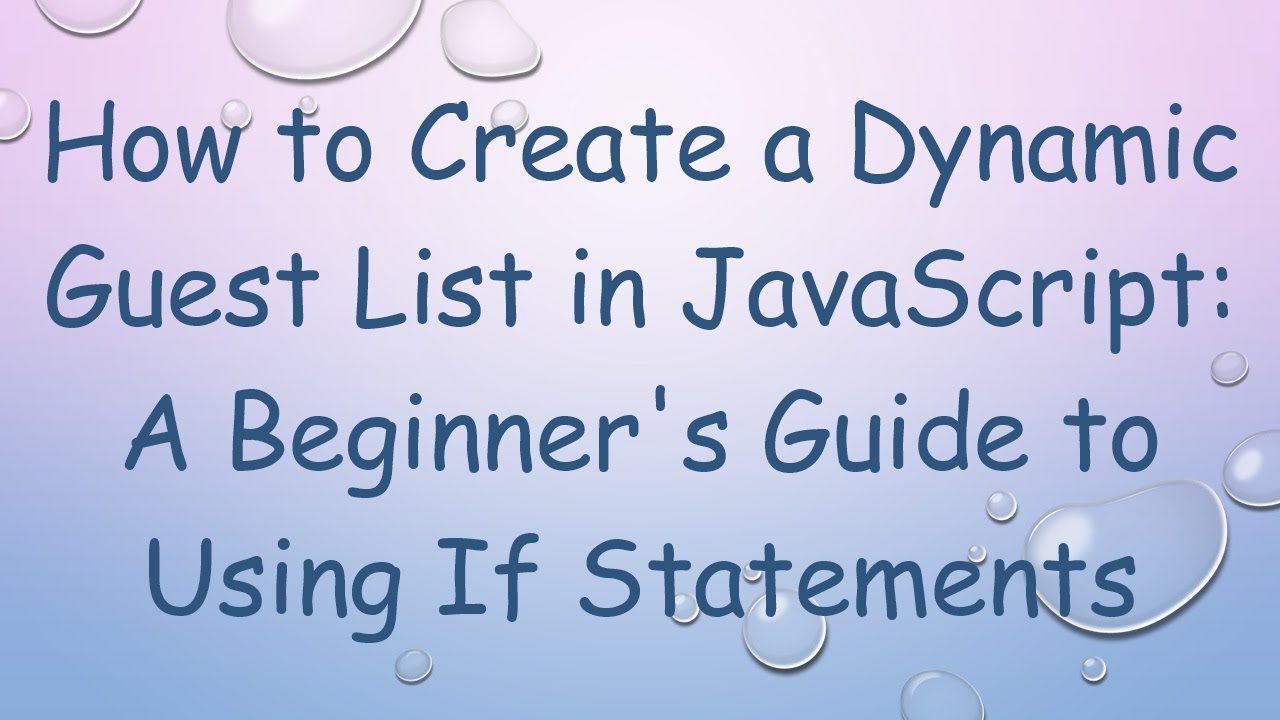
Показать описание
Learn how to build a smart guest list in JavaScript that gives special greetings for different guests using if statements and arrays.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: How to write a javascript to discern between specific guests for a "guest list" variable array
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
How to Create a Dynamic Guest List in JavaScript
As a budding programmer, you're probably excited about using conditional statements and arrays to create dynamic applications. Today, we're going to tackle a common scenario: creating a guest list in JavaScript. This guest list will allow for special greetings based on the identity of the guest. Let’s jump right into how this works!
The Problem: Understanding the Guest Greeting Logic
Imagine you have a guest list with several names, and you'd like to greet guests in a unique way. Specifically, there is one special guest who should be greeted differently from the others. However, if the name you’re checking doesn’t appear on either list, the response should be to tell them they can't enter.
From the initial code snippet you wrote, you faced a problem where the code would give multiple alerts for one guest, which can be confusing.
The Initial Code Snippet
Here’s a glimpse of the initial code:
[[See Video to Reveal this Text or Code Snippet]]
The issue here is primarily twofold:
Incorrect Variable Reference: You are checking for "guestName" as a string instead of the variable guestName.
Logical Flow: The order of your if statements allows both greetings to trigger if the guest is the owner.
The Solution: Structuring Your If Statements
To streamline this process, you can use if, else if, and else statements to avoid multiple alerts and to provide a clear path for the code to follow based on the guestName.
Step-by-Step Code Adjustment
Here’s how to properly structure your code:
[[See Video to Reveal this Text or Code Snippet]]
Explanation of the Corrected Code
Using includes Method Correctly: Instead of checking for a string, we're checking if ownerList contains the variable guestName.
Organizing Conditions:
First Condition: Checks if the guest is in ownerList. If true, greet them respectfully.
Second Condition: If they’re not in the owner list, it checks the guestList. If they are on this list, welcome them.
Else Statement: If the name doesn’t match either list, tell them they can't enter.
Conclusion: Mastering If Statements in JavaScript
By following this structured approach, you now have a dynamic guest list management system in JavaScript. You learned how to properly organize conditional logic to ensure that the right greeting is given to the right guest without redundancy.
Don't hesitate to experiment with this code and play around with different names and lists. The more you practice, the more confident you’ll feel with if statements and arrays in JavaScript!
Happy coding!
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: How to write a javascript to discern between specific guests for a "guest list" variable array
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
How to Create a Dynamic Guest List in JavaScript
As a budding programmer, you're probably excited about using conditional statements and arrays to create dynamic applications. Today, we're going to tackle a common scenario: creating a guest list in JavaScript. This guest list will allow for special greetings based on the identity of the guest. Let’s jump right into how this works!
The Problem: Understanding the Guest Greeting Logic
Imagine you have a guest list with several names, and you'd like to greet guests in a unique way. Specifically, there is one special guest who should be greeted differently from the others. However, if the name you’re checking doesn’t appear on either list, the response should be to tell them they can't enter.
From the initial code snippet you wrote, you faced a problem where the code would give multiple alerts for one guest, which can be confusing.
The Initial Code Snippet
Here’s a glimpse of the initial code:
[[See Video to Reveal this Text or Code Snippet]]
The issue here is primarily twofold:
Incorrect Variable Reference: You are checking for "guestName" as a string instead of the variable guestName.
Logical Flow: The order of your if statements allows both greetings to trigger if the guest is the owner.
The Solution: Structuring Your If Statements
To streamline this process, you can use if, else if, and else statements to avoid multiple alerts and to provide a clear path for the code to follow based on the guestName.
Step-by-Step Code Adjustment
Here’s how to properly structure your code:
[[See Video to Reveal this Text or Code Snippet]]
Explanation of the Corrected Code
Using includes Method Correctly: Instead of checking for a string, we're checking if ownerList contains the variable guestName.
Organizing Conditions:
First Condition: Checks if the guest is in ownerList. If true, greet them respectfully.
Second Condition: If they’re not in the owner list, it checks the guestList. If they are on this list, welcome them.
Else Statement: If the name doesn’t match either list, tell them they can't enter.
Conclusion: Mastering If Statements in JavaScript
By following this structured approach, you now have a dynamic guest list management system in JavaScript. You learned how to properly organize conditional logic to ensure that the right greeting is given to the right guest without redundancy.
Don't hesitate to experiment with this code and play around with different names and lists. The more you practice, the more confident you’ll feel with if statements and arrays in JavaScript!
Happy coding!