filmov
tv
Creating Reusable JavaScript Objects with Parameterized Constructors and Methods
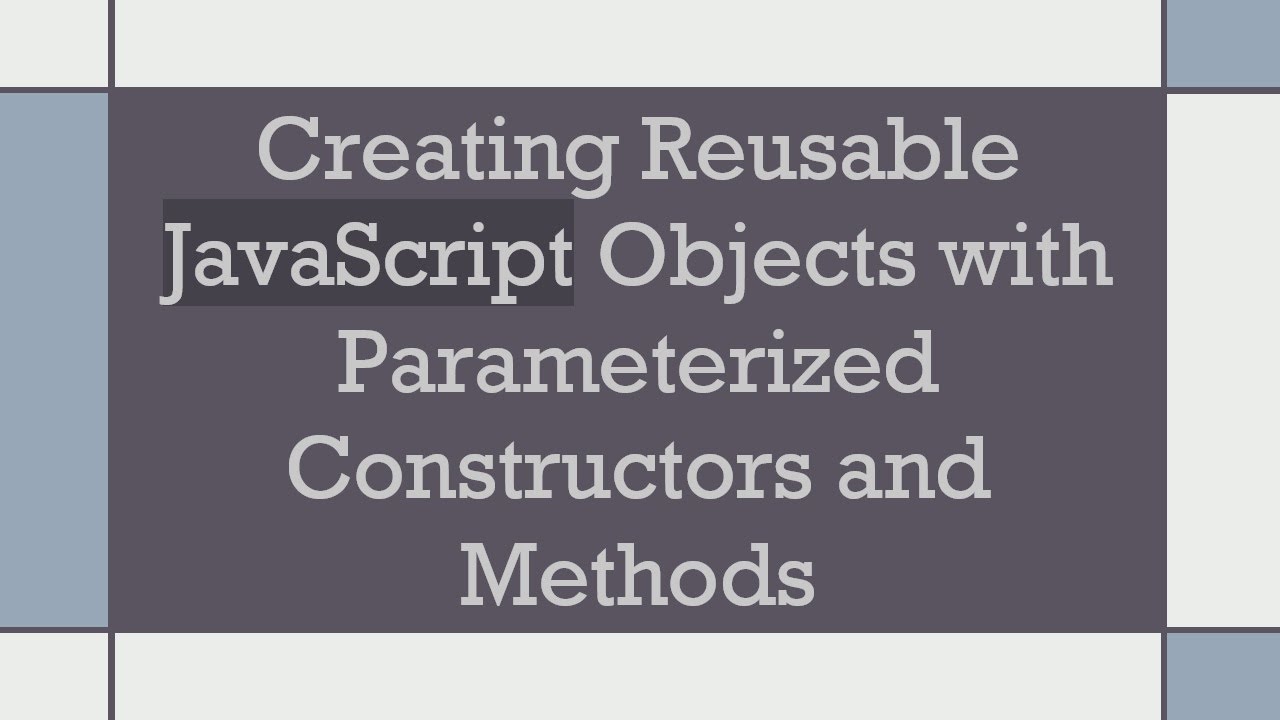
Показать описание
Summary: Learn about encapsulation in JavaScript by creating reusable objects with parameterized constructors and methods, enhancing code organization and maintainability.
---
In the world of programming, achieving code reusability and maintainability is essential for developing efficient and scalable software. One way to accomplish this in JavaScript is through encapsulation, which involves bundling the data (properties) and the methods that operate on the data into a single unit - the object.
Encapsulating JavaScript Objects
Encapsulation in JavaScript is a paradigm that emphasizes data hiding and method binding bundled in a single unit. This concept not only makes your code more maintainable but also increases the reusability of your code components. With encapsulation, you can shield internal object details from the external world, offering a public interface without exposing complexities.
Creating Reusable Objects with Parameterized Constructors
To create a reusable JavaScript object, we often use a parameterized constructor. This allows you to generate unique instances of an object with customized initial states. Here's a simple illustration:
[[See Video to Reveal this Text or Code Snippet]]
Key Points to Consider:
Parameterized Constructor: The Car constructor takes parameters make, model, and year, allowing you to create a car with a specific make, model, and year.
Encapsulation: The constructor encapsulates car properties and the getCarInfo method, providing a clean interface to interact with Car objects.
Reusability and Maintainability: The constructor function can be reused to create multiple car objects. If changes are needed, they can be made within the Car constructor. All instances automatically benefit from updates, enhancing maintainability.
Going Further with Prototypes
To avoid recreating methods for each instance, you can use JavaScript prototypes, which allow sharing methods across instances, thus saving memory:
[[See Video to Reveal this Text or Code Snippet]]
By using prototypes, the getCarInfo method is defined only once, and all Car instances share it, optimizing both memory usage and performance.
Conclusion
By employing encapsulation through parameterized constructors and methods, JavaScript developers can efficiently create reusable and maintainable objects. This practice not only aids in organizing code but also leverages prototypes to enhance performance. As you venture into more complex JavaScript applications, understanding these concepts will be invaluable for writing clean, efficient, and scalable code.
---
In the world of programming, achieving code reusability and maintainability is essential for developing efficient and scalable software. One way to accomplish this in JavaScript is through encapsulation, which involves bundling the data (properties) and the methods that operate on the data into a single unit - the object.
Encapsulating JavaScript Objects
Encapsulation in JavaScript is a paradigm that emphasizes data hiding and method binding bundled in a single unit. This concept not only makes your code more maintainable but also increases the reusability of your code components. With encapsulation, you can shield internal object details from the external world, offering a public interface without exposing complexities.
Creating Reusable Objects with Parameterized Constructors
To create a reusable JavaScript object, we often use a parameterized constructor. This allows you to generate unique instances of an object with customized initial states. Here's a simple illustration:
[[See Video to Reveal this Text or Code Snippet]]
Key Points to Consider:
Parameterized Constructor: The Car constructor takes parameters make, model, and year, allowing you to create a car with a specific make, model, and year.
Encapsulation: The constructor encapsulates car properties and the getCarInfo method, providing a clean interface to interact with Car objects.
Reusability and Maintainability: The constructor function can be reused to create multiple car objects. If changes are needed, they can be made within the Car constructor. All instances automatically benefit from updates, enhancing maintainability.
Going Further with Prototypes
To avoid recreating methods for each instance, you can use JavaScript prototypes, which allow sharing methods across instances, thus saving memory:
[[See Video to Reveal this Text or Code Snippet]]
By using prototypes, the getCarInfo method is defined only once, and all Car instances share it, optimizing both memory usage and performance.
Conclusion
By employing encapsulation through parameterized constructors and methods, JavaScript developers can efficiently create reusable and maintainable objects. This practice not only aids in organizing code but also leverages prototypes to enhance performance. As you venture into more complex JavaScript applications, understanding these concepts will be invaluable for writing clean, efficient, and scalable code.