filmov
tv
Write a program that will give you in hand monthly salary after deduction
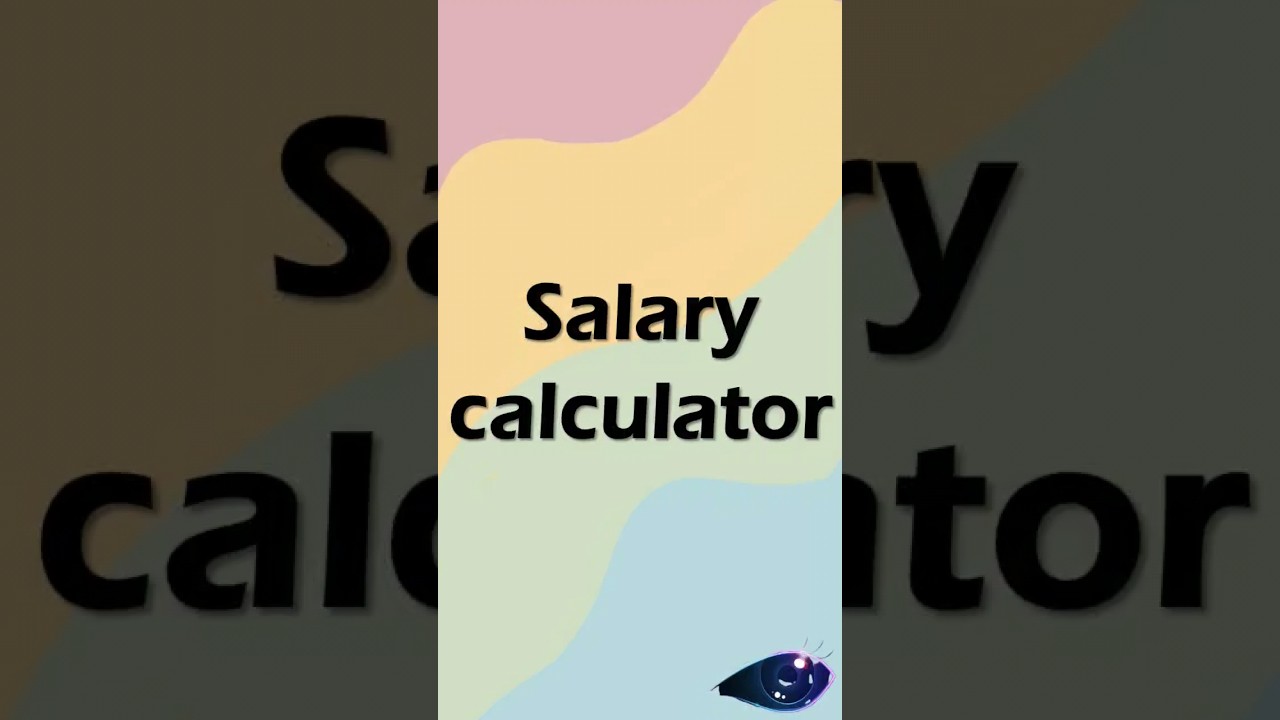
Показать описание
here's a Python program that calculates the monthly salary after deductions based on the given criteria:
python
Copy code
# Get input from user
ctc = float(input("Enter your CTC in Lakhs: "))
# Calculate HRA, DA, and PF
hra = ctc * 0.1
da = ctc * 0.05
pf = ctc * 0.03
# Calculate total deductions
total_deductions = hra + da + pf
# Calculate taxable income
taxable_income = ctc - total_deductions
# Calculate tax based on salary range
if taxable_income less than 5:
tax = 0
elif taxable_income greater than equal to 5 and taxable_income less than 10:
tax = 0.1
elif taxable_income greater than equal to 10 and taxable_income less than 20:
tax = 0.2
else:
tax = 0.3
# Calculate net salary
net_salary = ctc - total_deductions - (taxable_income * tax)
# Display the result
print("Your net monthly salary is:", net_salary, "Lakhs")
Note: This program assumes that the input provided by the user is a valid float value in Lakhs.
python
Copy code
# Get input from user
ctc = float(input("Enter your CTC in Lakhs: "))
# Calculate HRA, DA, and PF
hra = ctc * 0.1
da = ctc * 0.05
pf = ctc * 0.03
# Calculate total deductions
total_deductions = hra + da + pf
# Calculate taxable income
taxable_income = ctc - total_deductions
# Calculate tax based on salary range
if taxable_income less than 5:
tax = 0
elif taxable_income greater than equal to 5 and taxable_income less than 10:
tax = 0.1
elif taxable_income greater than equal to 10 and taxable_income less than 20:
tax = 0.2
else:
tax = 0.3
# Calculate net salary
net_salary = ctc - total_deductions - (taxable_income * tax)
# Display the result
print("Your net monthly salary is:", net_salary, "Lakhs")
Note: This program assumes that the input provided by the user is a valid float value in Lakhs.