filmov
tv
Image Recognition and Python Part 7
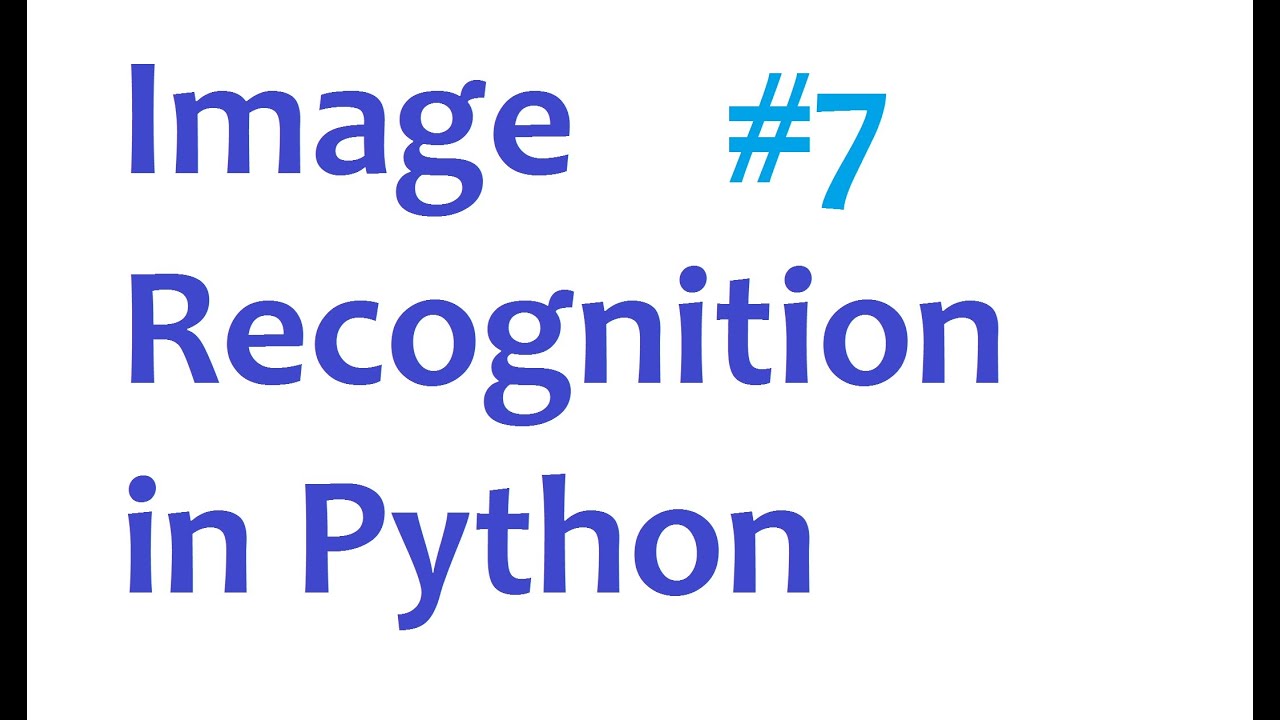
Показать описание
This is the seventh video to my image recognition basics series. Image recognition can be used for all sorts of things like facial recognition, identifying what is in pictures, character recognition, and more.
Image Recognition and Python Part 1
How to make advanced image recognition bots using python
Build Image Recognition Model In Python in 20 min
Machine Learning for Facial Recognition in Python in 60 Seconds #shorts
Image Processing with OpenCV and Python
Image Recognition and Python Part 2
AI Image Recognition Using Python | No Internet Required
Image Recognition and Python Part 8
Day 6 - Pooling Layer & CNN Architecture | Computer Vision for Developers
This Python module is your go-to for speech and image recognition!
Building a Face Detection Tool using Python! 🤖 #technology #tech #coding #programming
Image Recognition and Python Part 7
Image Recognition and Python Part 6
Image Recognition and Python Part 10
Image Recognition and Python Part 5
Image Recognition and Python Part 3
Multiple faces detection with Python and OpenCV
Image Recognition and Python Part 4
Object Detection in 60 Seconds using Python and YOLOv5 #shorts
Object Detection in 10 minutes with YOLOv5 & Python!
Image Recognition with LLaVa in Python
Face Recognition Using Python Programming
Face Recognition System
Employee Attendance Management System using Face Recognition Technology
Комментарии