filmov
tv
Understanding Why an Abstract Static Method Returns None in Python
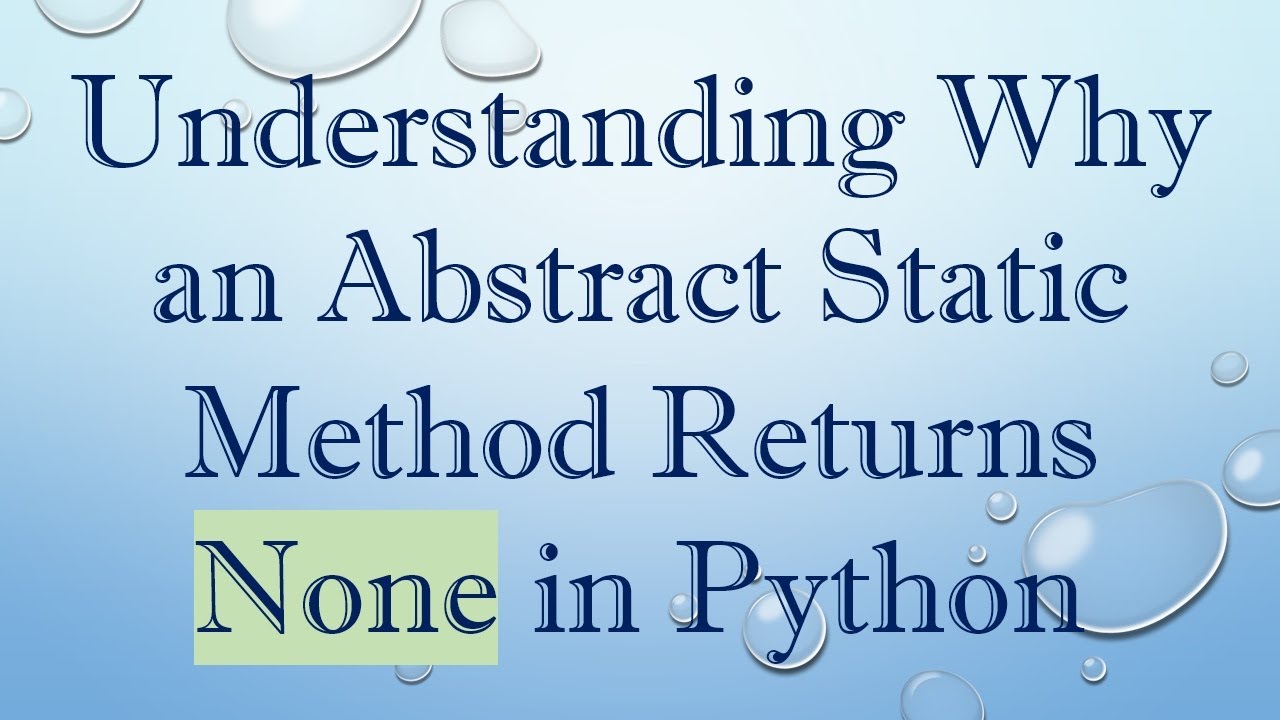
Показать описание
Discover the nuances of abstract static methods in Python and find out why they return `None` if not implemented in concrete classes.
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Why does this abstract static method return None?
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Understanding Why an Abstract Static Method Returns None in Python
In the world of Python programming, we've often come across the concept of abstract classes and methods. These constructs are fundamental for structuring our code effectively and forcing subclasses to implement certain functionalities. However, what happens when we have an abstract static method that is never implemented? You might be surprised to learn that it actually returns None. In this guide, we'll explore this puzzling behavior and how to properly handle it in your code.
The Problem: An Abstract Static Method That Returns None
Consider the following code snippet:
[[See Video to Reveal this Text or Code Snippet]]
Understanding the Behavior
Why Does It Return None?
When the abstract static method is defined with a body that consists of merely an ellipsis ..., it might be interpreted as an incomplete method that doesn't actually do anything. Hence, when you call it from the Concrete class, Python has no behavior to implement and simply defaults to returning None.
Expected Behavior Vs. Reality
You might logically think that the absence of an implementation would raise a NotImplementedError. However, because the foo() method is defined as an abstract class method without an explicit implementation, it allows the call to proceed, resulting in the return of None. To prevent this ambiguous outcome, it’s essential to ensure that you express the deliberate omission of an implementation clearly.
The Solution: How to Properly Implement Abstract Methods
To clarify intent and communicate that a method must be implemented by any subclass, we can use the NotImplementedError. Here’s how to adjust your class definition:
[[See Video to Reveal this Text or Code Snippet]]
Key Takeaways from the Solution
Explicit Error Raising: By raising a NotImplementedError, you're ensuring that anyone trying to use foo() without an implementation will receive a clear and explicit error message.
Clarity for Future Reference: This approach also serves documentation purposes. Future developers can immediately understand that they need to provide an implementation for foo() in any subclasses.
When Would You Get an Error?
You will encounter errors if you attempt to instantiate Concrete directly since it is still an abstract class until all abstract methods have been implemented:
[[See Video to Reveal this Text or Code Snippet]]
If Concrete does eventually provide its own implementation of foo(), that method will be invoked instead of raising an error:
[[See Video to Reveal this Text or Code Snippet]]
Conclusion
Understanding how abstract static methods work in Python is crucial for effective programming with abstract classes. By ensuring explicitly that an abstract method raises a NotImplementedError, you can prevent ambiguous behavior and improve code clarity. Next time you encounter this scenario, remember that raising errors can help avoid confusion in your program architecture and enhance overall code maintainability.
Remember, to create robust and error-free code, always provide the necessary implementations or communicate the absence of them clearly!
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Why does this abstract static method return None?
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Understanding Why an Abstract Static Method Returns None in Python
In the world of Python programming, we've often come across the concept of abstract classes and methods. These constructs are fundamental for structuring our code effectively and forcing subclasses to implement certain functionalities. However, what happens when we have an abstract static method that is never implemented? You might be surprised to learn that it actually returns None. In this guide, we'll explore this puzzling behavior and how to properly handle it in your code.
The Problem: An Abstract Static Method That Returns None
Consider the following code snippet:
[[See Video to Reveal this Text or Code Snippet]]
Understanding the Behavior
Why Does It Return None?
When the abstract static method is defined with a body that consists of merely an ellipsis ..., it might be interpreted as an incomplete method that doesn't actually do anything. Hence, when you call it from the Concrete class, Python has no behavior to implement and simply defaults to returning None.
Expected Behavior Vs. Reality
You might logically think that the absence of an implementation would raise a NotImplementedError. However, because the foo() method is defined as an abstract class method without an explicit implementation, it allows the call to proceed, resulting in the return of None. To prevent this ambiguous outcome, it’s essential to ensure that you express the deliberate omission of an implementation clearly.
The Solution: How to Properly Implement Abstract Methods
To clarify intent and communicate that a method must be implemented by any subclass, we can use the NotImplementedError. Here’s how to adjust your class definition:
[[See Video to Reveal this Text or Code Snippet]]
Key Takeaways from the Solution
Explicit Error Raising: By raising a NotImplementedError, you're ensuring that anyone trying to use foo() without an implementation will receive a clear and explicit error message.
Clarity for Future Reference: This approach also serves documentation purposes. Future developers can immediately understand that they need to provide an implementation for foo() in any subclasses.
When Would You Get an Error?
You will encounter errors if you attempt to instantiate Concrete directly since it is still an abstract class until all abstract methods have been implemented:
[[See Video to Reveal this Text or Code Snippet]]
If Concrete does eventually provide its own implementation of foo(), that method will be invoked instead of raising an error:
[[See Video to Reveal this Text or Code Snippet]]
Conclusion
Understanding how abstract static methods work in Python is crucial for effective programming with abstract classes. By ensuring explicitly that an abstract method raises a NotImplementedError, you can prevent ambiguous behavior and improve code clarity. Next time you encounter this scenario, remember that raising errors can help avoid confusion in your program architecture and enhance overall code maintainability.
Remember, to create robust and error-free code, always provide the necessary implementations or communicate the absence of them clearly!