filmov
tv
Arrays and Pointers in Pascal: Utilizing Pointers with Arrays
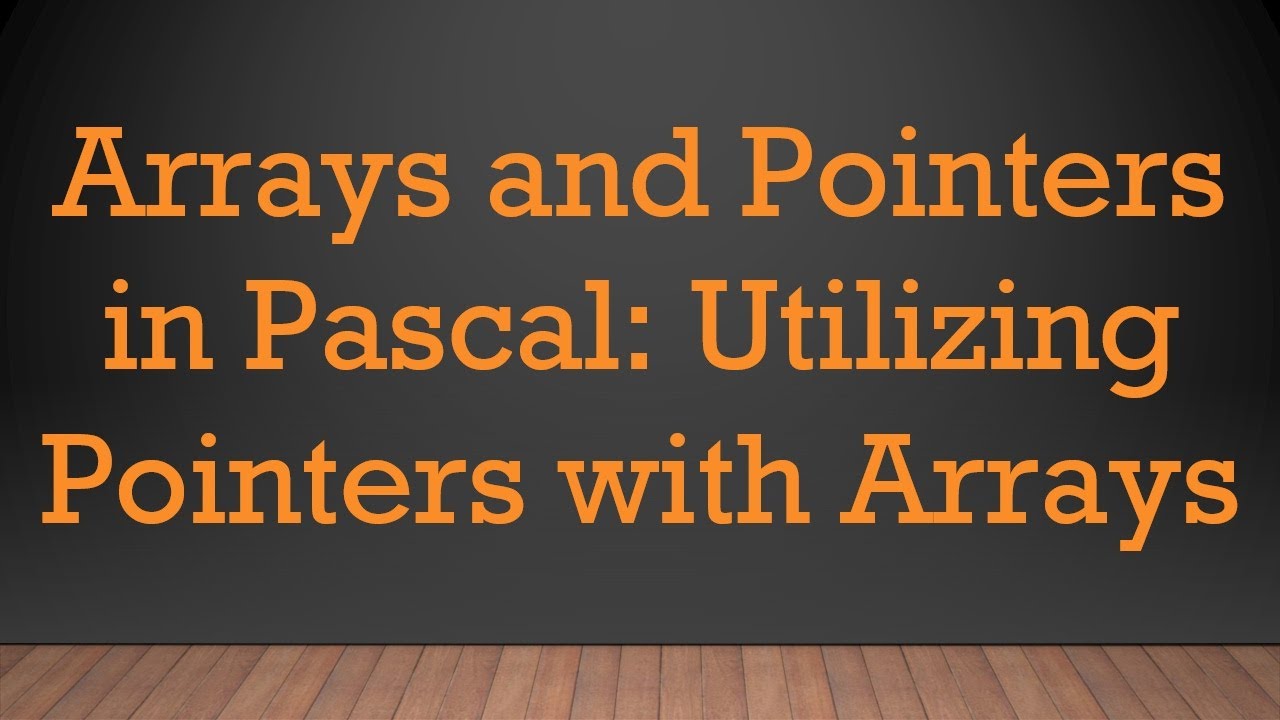
Показать описание
Explore the use of arrays and pointers in Pascal, including how to utilize pointers with arrays for efficient memory management and dynamic data structures.
---
Disclaimer/Disclosure: Some of the content was synthetically produced using various Generative AI (artificial intelligence) tools; so, there may be inaccuracies or misleading information present in the video. Please consider this before relying on the content to make any decisions or take any actions etc. If you still have any concerns, please feel free to write them in a comment. Thank you.
---
In Pascal, arrays and pointers are fundamental constructs that allow for the creation of complex and efficient data structures. Understanding how to utilize pointers with arrays can enhance your ability to manage memory dynamically and implement advanced algorithms. This guide delves into the concepts of arrays and pointers in Pascal, and demonstrates how to effectively use pointers with arrays.
Arrays in Pascal
An array in Pascal is a collection of elements of the same type, indexed by contiguous integers. Arrays can be declared in various sizes and dimensions, making them versatile for different types of data manipulation.
Declaring Arrays
To declare an array in Pascal, you specify the type and the range of indices. For example:
[[See Video to Reveal this Text or Code Snippet]]
This declaration creates an array named myArray with 10 integer elements, indexed from 1 to 10.
Accessing Array Elements
Array elements are accessed using their indices:
[[See Video to Reveal this Text or Code Snippet]]
This code assigns the value 5 to the first element of myArray and then prints it.
Pointers in Pascal
A pointer in Pascal is a variable that stores the address of another variable. Pointers are used for dynamic memory allocation and for creating complex data structures like linked lists and trees.
Declaring Pointers
To declare a pointer, you use the ^ symbol. For instance:
[[See Video to Reveal this Text or Code Snippet]]
This declaration creates a pointer ptr that can store the address of an integer variable.
Using Pointers
Before using a pointer, you need to allocate memory for it using the New procedure:
[[See Video to Reveal this Text or Code Snippet]]
In this example, memory is allocated for the pointer ptr, the value 10 is assigned to the memory location, the value is printed, and finally, the memory is deallocated using Dispose.
Utilizing Pointers with Arrays
Combining arrays and pointers allows for dynamic array manipulation, such as resizing arrays and creating arrays of pointers.
Dynamic Arrays
To create a dynamic array, you can use a pointer to an array type:
[[See Video to Reveal this Text or Code Snippet]]
This code dynamically allocates memory for an array of 10 integers, initializes it with values, prints the values, and then frees the allocated memory.
Arrays of Pointers
You can also create an array where each element is a pointer, allowing for dynamic and flexible data structures:
[[See Video to Reveal this Text or Code Snippet]]
This example demonstrates creating an array where each element is a pointer to an integer. Each pointer is allocated memory, assigned a value, and then deallocated.
Conclusion
Utilizing pointers with arrays in Pascal opens up a wide range of possibilities for dynamic memory management and complex data structures. By understanding and applying these concepts, you can create efficient and flexible programs capable of handling various data manipulation tasks.
---
Disclaimer/Disclosure: Some of the content was synthetically produced using various Generative AI (artificial intelligence) tools; so, there may be inaccuracies or misleading information present in the video. Please consider this before relying on the content to make any decisions or take any actions etc. If you still have any concerns, please feel free to write them in a comment. Thank you.
---
In Pascal, arrays and pointers are fundamental constructs that allow for the creation of complex and efficient data structures. Understanding how to utilize pointers with arrays can enhance your ability to manage memory dynamically and implement advanced algorithms. This guide delves into the concepts of arrays and pointers in Pascal, and demonstrates how to effectively use pointers with arrays.
Arrays in Pascal
An array in Pascal is a collection of elements of the same type, indexed by contiguous integers. Arrays can be declared in various sizes and dimensions, making them versatile for different types of data manipulation.
Declaring Arrays
To declare an array in Pascal, you specify the type and the range of indices. For example:
[[See Video to Reveal this Text or Code Snippet]]
This declaration creates an array named myArray with 10 integer elements, indexed from 1 to 10.
Accessing Array Elements
Array elements are accessed using their indices:
[[See Video to Reveal this Text or Code Snippet]]
This code assigns the value 5 to the first element of myArray and then prints it.
Pointers in Pascal
A pointer in Pascal is a variable that stores the address of another variable. Pointers are used for dynamic memory allocation and for creating complex data structures like linked lists and trees.
Declaring Pointers
To declare a pointer, you use the ^ symbol. For instance:
[[See Video to Reveal this Text or Code Snippet]]
This declaration creates a pointer ptr that can store the address of an integer variable.
Using Pointers
Before using a pointer, you need to allocate memory for it using the New procedure:
[[See Video to Reveal this Text or Code Snippet]]
In this example, memory is allocated for the pointer ptr, the value 10 is assigned to the memory location, the value is printed, and finally, the memory is deallocated using Dispose.
Utilizing Pointers with Arrays
Combining arrays and pointers allows for dynamic array manipulation, such as resizing arrays and creating arrays of pointers.
Dynamic Arrays
To create a dynamic array, you can use a pointer to an array type:
[[See Video to Reveal this Text or Code Snippet]]
This code dynamically allocates memory for an array of 10 integers, initializes it with values, prints the values, and then frees the allocated memory.
Arrays of Pointers
You can also create an array where each element is a pointer, allowing for dynamic and flexible data structures:
[[See Video to Reveal this Text or Code Snippet]]
This example demonstrates creating an array where each element is a pointer to an integer. Each pointer is allocated memory, assigned a value, and then deallocated.
Conclusion
Utilizing pointers with arrays in Pascal opens up a wide range of possibilities for dynamic memory management and complex data structures. By understanding and applying these concepts, you can create efficient and flexible programs capable of handling various data manipulation tasks.