filmov
tv
Python 3D Graphics Tutorial 20: Annotating Analog Clock with Numeric Values
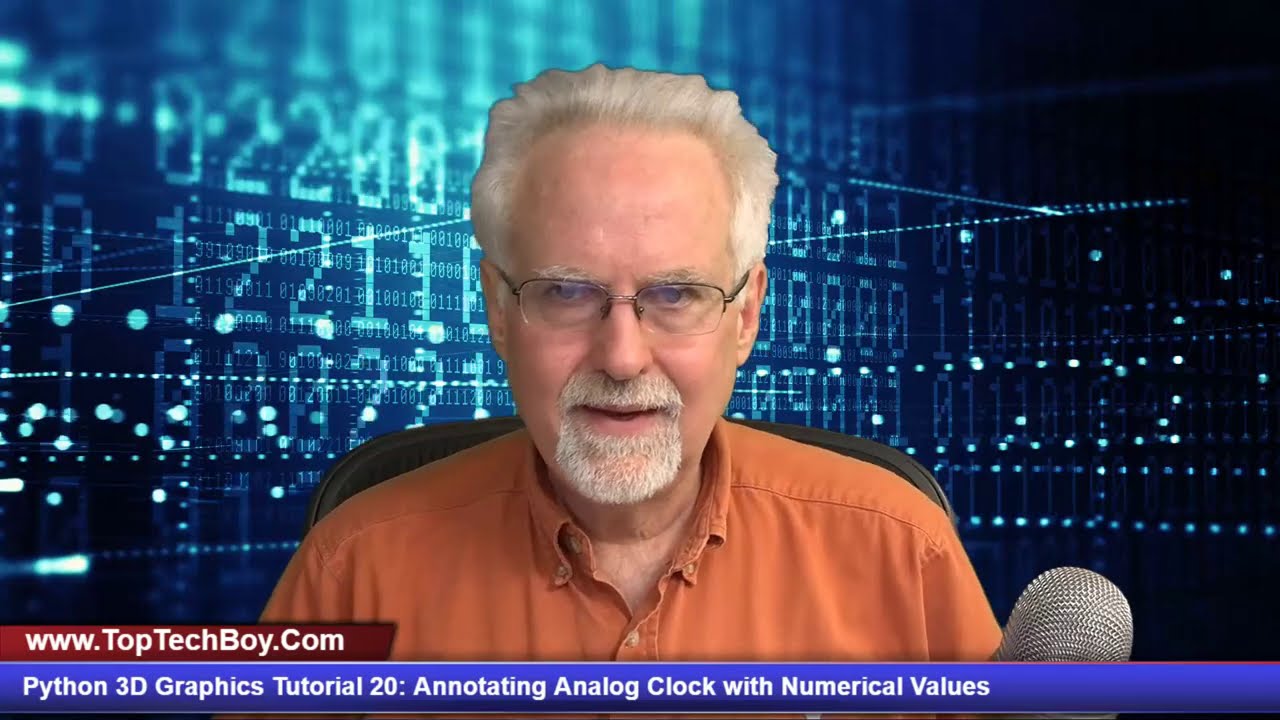
Показать описание
You guys can help me out over at Patreon, and that will help me keep my gear updated, and help me keep this quality content coming:
In this video we show step-by-step instructions on how to build a 3D clock face model in Vpython. We will show how to place text on the graphic and how to properly align the numbers on the clock face.
#Python
#Lessons
#Vpython
In this video we show step-by-step instructions on how to build a 3D clock face model in Vpython. We will show how to place text on the graphic and how to properly align the numbers on the clock face.
#Python
#Lessons
#Vpython
Python 3D Graphics Tutorial 20: Annotating Analog Clock with Numeric Values
Python 3D Graphics Tutorial 21: Understanding and Using Widgets in Vpython
Python 3D Graphics Tutorial 15: Three Dimensional Clock Animation
Python 3D Graphics Tutorial 17: Creating Accurate 3D Clock in Vpython
Python 3D Graphics Tutorial 13: Understanding Orientation in Three Dimensional Parameter Space
Python 3D Graphics Tutorial 12: Understanding Orientation and Axis Parameters
Python 3D Graphics Tutorial 4: Understanding 3D Graphic Parameters
Python 3D Graphics Tutorial 6: Animating 3D Objects by Changing Dimensions in Visual Python
Python 3D Graphics Tutorial 10: Program for Orb With Continuously Varying Color Rainbow
Python 3D Graphics Tutorial 3: Designing 3D Models with Parameters
How to create graphics using Python turtle 🐍🐢 #coding
Amazing Flower Design using Python turtle 🐢 #python #coding #funny #viral #trending #design
Python Turtle 🐢 || Graphics Design || #python #turtle #design #coding #shorts #shortvideo
Python 3D Graphics Tutorial 1: Installation and Demonstration of Vpython
New Python Coders Be Like...
Can You Draw this 🤔 Amazing Python Turtle Moving Graphics Design #shorts #shortsbreak
Superb graphics with python turtle | Python Turtle Graphics 20 | Awesome python turtle graphics
Rangoli Design {Colourful Ball} using Python Turtle Graphics 🔥🔥#shorts
Robert Downey Jr. Sketch using Python Turtle 🐢 Graphics in Python Coding #shorts #python #coding
Superb graphics with python turtle | Python Turtle Graphics 21 | Awesome python turtle graphics
Normal People VS Programmers #coding #python #programming #easy #funny #short
Python 3D Graphics Tutorial 19: Adding Text to Your Visual Python Models
Make Zombie Running Animation With 5 Lines Of Python #Shorts
Generative Cubes in #python #processing 🧊
Комментарии