filmov
tv
Unlocking the Power of Destructuring in JavaScript: Conditionally Assigning Object Properties
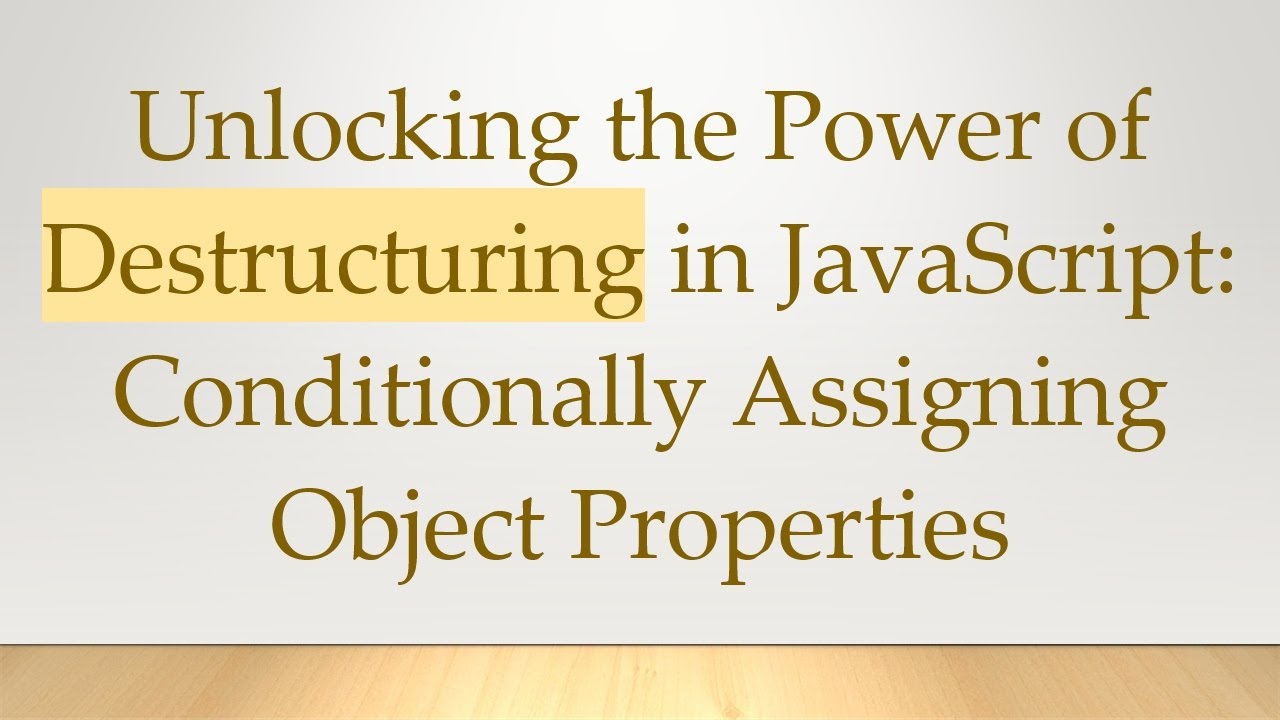
Показать описание
Discover an elegant solution to conditionally assign properties from one object to another in JavaScript using destructuring. Learn how to simplify your code with this efficient technique!
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Conditionally assign from part of an object
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Unlocking the Power of Destructuring in JavaScript: Conditionally Assigning Object Properties
In the world of JavaScript, working with objects is an everyday task for developers. However, there are times when you need to conditionally extract only a subset of properties from a larger object. This can lead to tedious and repetitive code. The good news is – there’s a neat solution using destructuring!
The Problem: Conditionally Assigning Object Properties
Consider the following scenario: You have a function that returns a large object with many keys and values. You need to create a new object that only includes certain keys from this large object based on a boolean condition.
Here’s a simplified version of your initial approach:
[[See Video to Reveal this Text or Code Snippet]]
In this code, you may run into issues because relying on destructuring directly in this manner results in reference errors. Plus, it creates separate variables instead of constructing the desired smallObject.
The Solution: Using Inline Functions with Destructuring
Now, let’s focus on a robust solution that simplifies your code and achieves your goal efficiently. The method involves using inline functions to perform the destructuring within an expression. Here’s how you can implement it:
Step-by-Step Breakdown
Define Your Function:
First, you will retain your bigObject function that returns the complete object.
Conditional Assignment:
Use a ternary operator to check the condition, utilizing an inline arrow function for destructuring.
Construct the New Object:
The inline function takes the output of bigObject() as its argument, destructures it, and returns a new object with the selected properties.
Implementation
Here is the refined implementation:
[[See Video to Reveal this Text or Code Snippet]]
Explanation of the Code
The bigObject() Function: This simulates your original object with multiple properties.
Destructuring and Arrow Function: The inline function takes care of destructuring the specific keys (keyA and keyB) you need when aBool is true. If the condition is false, smallObject remains empty.
Final Output: When you run the code with aBool set to true, smallObject will output { keyA: 1, keyB: 2 }. Otherwise, it will be {}.
Benefits of This Approach
Conciseness: This one-liner is much shorter and easier to read than doing multiple assignments.
Flexibility: You can easily modify which properties to include just by changing the destructured keys in the function.
Maintainability: Reduced complexity leads to easier maintenance in the future.
Conclusion
Using destructuring for conditionally assigning properties from one object to another can significantly streamline your JavaScript code. This elegant solution not only makes your intent clear but also enhances the readability and efficiency of your code. Give it a try in your next project!
Feel free to explore more about JavaScript and its features as you become adept at manipulating objects!
---
Visit these links for original content and any more details, such as alternate solutions, latest updates/developments on topic, comments, revision history etc. For example, the original title of the Question was: Conditionally assign from part of an object
If anything seems off to you, please feel free to write me at vlogize [AT] gmail [DOT] com.
---
Unlocking the Power of Destructuring in JavaScript: Conditionally Assigning Object Properties
In the world of JavaScript, working with objects is an everyday task for developers. However, there are times when you need to conditionally extract only a subset of properties from a larger object. This can lead to tedious and repetitive code. The good news is – there’s a neat solution using destructuring!
The Problem: Conditionally Assigning Object Properties
Consider the following scenario: You have a function that returns a large object with many keys and values. You need to create a new object that only includes certain keys from this large object based on a boolean condition.
Here’s a simplified version of your initial approach:
[[See Video to Reveal this Text or Code Snippet]]
In this code, you may run into issues because relying on destructuring directly in this manner results in reference errors. Plus, it creates separate variables instead of constructing the desired smallObject.
The Solution: Using Inline Functions with Destructuring
Now, let’s focus on a robust solution that simplifies your code and achieves your goal efficiently. The method involves using inline functions to perform the destructuring within an expression. Here’s how you can implement it:
Step-by-Step Breakdown
Define Your Function:
First, you will retain your bigObject function that returns the complete object.
Conditional Assignment:
Use a ternary operator to check the condition, utilizing an inline arrow function for destructuring.
Construct the New Object:
The inline function takes the output of bigObject() as its argument, destructures it, and returns a new object with the selected properties.
Implementation
Here is the refined implementation:
[[See Video to Reveal this Text or Code Snippet]]
Explanation of the Code
The bigObject() Function: This simulates your original object with multiple properties.
Destructuring and Arrow Function: The inline function takes care of destructuring the specific keys (keyA and keyB) you need when aBool is true. If the condition is false, smallObject remains empty.
Final Output: When you run the code with aBool set to true, smallObject will output { keyA: 1, keyB: 2 }. Otherwise, it will be {}.
Benefits of This Approach
Conciseness: This one-liner is much shorter and easier to read than doing multiple assignments.
Flexibility: You can easily modify which properties to include just by changing the destructured keys in the function.
Maintainability: Reduced complexity leads to easier maintenance in the future.
Conclusion
Using destructuring for conditionally assigning properties from one object to another can significantly streamline your JavaScript code. This elegant solution not only makes your intent clear but also enhances the readability and efficiency of your code. Give it a try in your next project!
Feel free to explore more about JavaScript and its features as you become adept at manipulating objects!