filmov
tv
Basic Python 17: Python Namespace and Scope
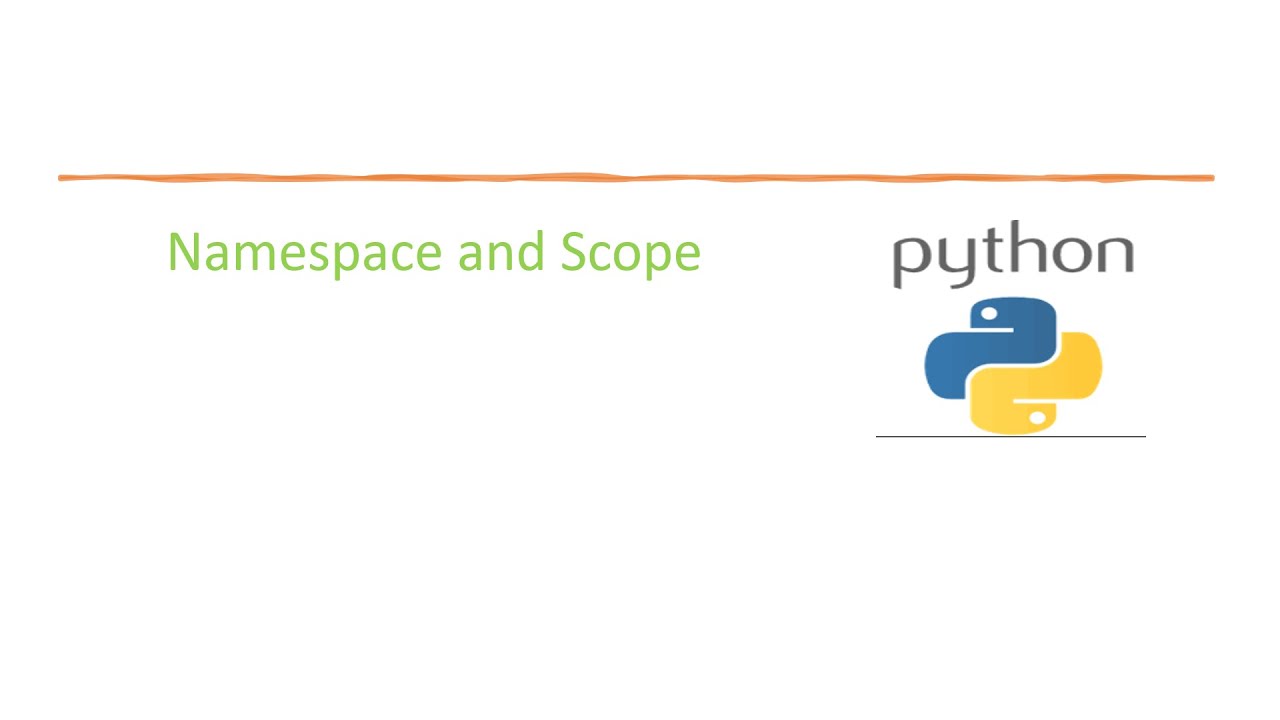
Показать описание
Python Namespace and Scope:
---------------------------
What is Name in Python?
Name (also called identifier) is simply a name given to objects. Everything in Python is an object. Name is a way to access the underlying object.
ex:
when we do the assignment a = 2, 2 is an object stored in memory and a is the name we associate it with. We can get the address (in RAM) of some object through the built-in function id().
ex:
a = 2
print('id(2) =', id(2))
print('id(a) =', id(a))
a = 2 a = a+1 b = 2
= 2 b - 2
a - 2 a - 3 a - 3
What is a Namespace in Python?
- a namespace is a collection of names.
- you can imagine a namespace as a mapping of every name you have defined to corresponding objects.
- Different namespaces can co-exist at a given time but are completely isolated.
- A namespace containing all the built-in names is created when we start the Python interpreter and exists as long as the interpreter runs.
- This is the reason that built-in functions like id(), print() etc. are always available to us from any part of the program. Each module creates its own global namespace.
Built-in Namespace
Module: Global Namespace
Functional: Local Namespace
Python Variable Scope:
- Scope of the current function which has local names
- Scope of the module which has global names
- Outermost scope which has built-in names
ex:
def outer_function():
b = 20
def inner_func():
c = 30
a = 10
Here, the variable a is in the global namespace. Variable b is in the local namespace of outer_function() and c is in the nested local namespace of inner_function().
When we are in inner_function(), c is local to us, b is nonlocal and a is global. We can read as well as assign new values to c but can only read b and a from inner_function().
other Ex:
def outer_function():
a = 20
def inner_function():
a = 30
print('a =', a)
inner_function()
print('a =', a)
a = 10
outer_function()
print('a =', a)
other ex:
def outer_function():
global a
a = 20
def inner_function():
global a
a = 30
print('a =', a)
inner_function()
print('a =', a)
a = 10
outer_function()
print('a =', a)
___________________ API Automation __________________
______________ Programing Language _____________________
____________ Performances Testing ____________________
____________ Git and GitHub ______________________________
______________Manual Testing _____________________
_______________Automation Testing ___________________
_________________ Beginner Jenkins _____________________
---------------------------
What is Name in Python?
Name (also called identifier) is simply a name given to objects. Everything in Python is an object. Name is a way to access the underlying object.
ex:
when we do the assignment a = 2, 2 is an object stored in memory and a is the name we associate it with. We can get the address (in RAM) of some object through the built-in function id().
ex:
a = 2
print('id(2) =', id(2))
print('id(a) =', id(a))
a = 2 a = a+1 b = 2
= 2 b - 2
a - 2 a - 3 a - 3
What is a Namespace in Python?
- a namespace is a collection of names.
- you can imagine a namespace as a mapping of every name you have defined to corresponding objects.
- Different namespaces can co-exist at a given time but are completely isolated.
- A namespace containing all the built-in names is created when we start the Python interpreter and exists as long as the interpreter runs.
- This is the reason that built-in functions like id(), print() etc. are always available to us from any part of the program. Each module creates its own global namespace.
Built-in Namespace
Module: Global Namespace
Functional: Local Namespace
Python Variable Scope:
- Scope of the current function which has local names
- Scope of the module which has global names
- Outermost scope which has built-in names
ex:
def outer_function():
b = 20
def inner_func():
c = 30
a = 10
Here, the variable a is in the global namespace. Variable b is in the local namespace of outer_function() and c is in the nested local namespace of inner_function().
When we are in inner_function(), c is local to us, b is nonlocal and a is global. We can read as well as assign new values to c but can only read b and a from inner_function().
other Ex:
def outer_function():
a = 20
def inner_function():
a = 30
print('a =', a)
inner_function()
print('a =', a)
a = 10
outer_function()
print('a =', a)
other ex:
def outer_function():
global a
a = 20
def inner_function():
global a
a = 30
print('a =', a)
inner_function()
print('a =', a)
a = 10
outer_function()
print('a =', a)
___________________ API Automation __________________
______________ Programing Language _____________________
____________ Performances Testing ____________________
____________ Git and GitHub ______________________________
______________Manual Testing _____________________
_______________Automation Testing ___________________
_________________ Beginner Jenkins _____________________