filmov
tv
Pascal's Triangle - Leetcode 118 - Python
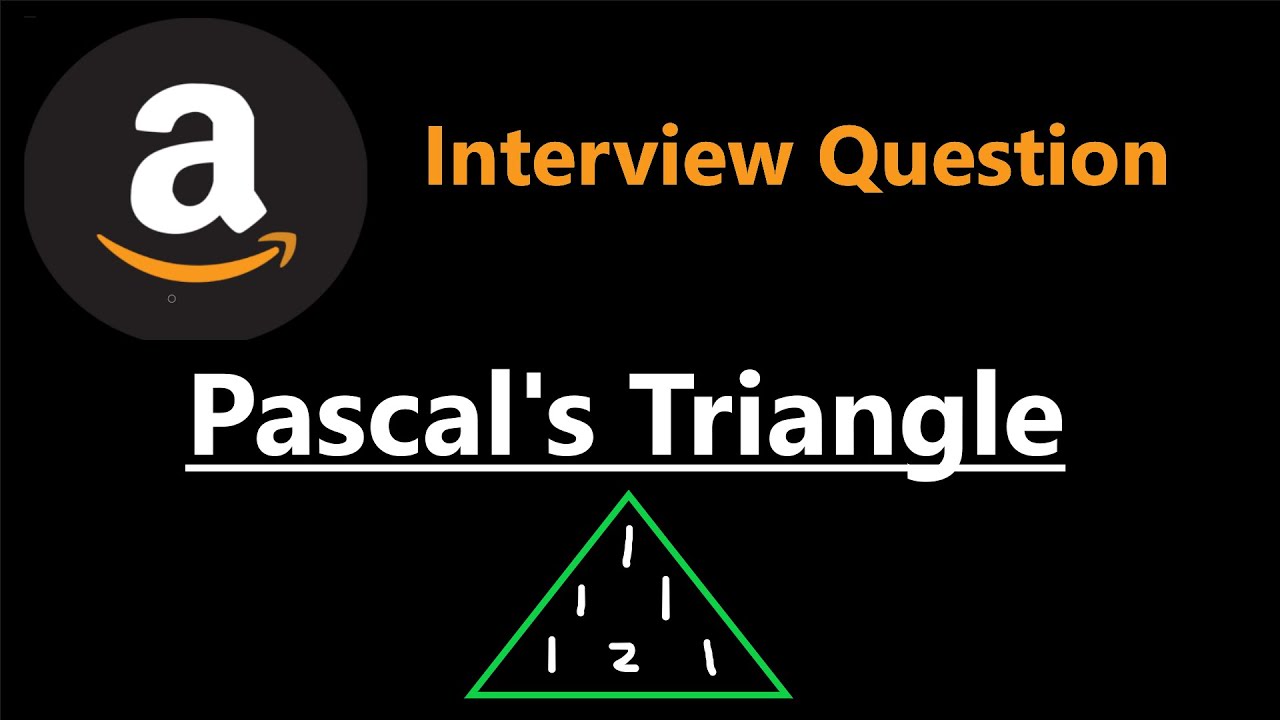
Показать описание
0:00 - Read the problem
1:40 - Drawing Explanation
5:55 - Coding Explanation
leetcode 118
#pascals #triangle #python
Disclosure: Some of the links above may be affiliate links, from which I may earn a small commission.
Pascal's Triangle - Leetcode 118 - Python
Pascal's Triangle (LeetCode 118) | Full solution w/ implementation | Fun patterns | Hidden insi...
Pascal's Triangle - Leetcode 118
LeetCode Pascal's Triangle Solution Explained - Java
Pascal Triangle | Finding nCr in minimal time
Pascal's Triangle LeetCode 118. Easy Code + Example + Explanation
Pascal's Triangle | LeetCode 118 | Easy
Pascals Triangle| Live Coding with Explanation | Leetcode - 118
Pascal’s Triangle | LeetCode 118 | Coding Interview Tutorial
LeetCode 118: Pascal's Triangle - Interview Prep Ep 34
Famous Google Coding Question! | Leetcode 118 - Pascal's Triangle
Unlocking the Secrets LeetCode 118. Pascal's Triangle The Fastest Way to Generate It in Go
Pascal's Triangle - Leetcode 118
118 Pascal's triangle | Leetcode | Interview Preparation | English | code io
Pascal's Triangle | Leetcode 118 | Live coding session 🔺🔺🔺
[Java] Leetcode 118. Pascal's Triangle [Arrays #3]
Pascal's Triangle (Explanation + Code: LeetCode 118)
Pascal's Triangle - LeetCode 118 - JavaScript
Leetcode 118 | Pascal's Triangle (Java Solution)
Pascal's Triangle | Simplest Approach | Application | DRY RUN | Adobe | Amazon | Leetcode - 118
Day 28/100 Leetcode Challenge. 118. Pascal's Triangle #coding #leetcode #code
Leetcode 118 | Pascal's triangle using dynamic programming
Pascal's Triangle: LeetCode #118 Easy Java Solution
Pascal's Triangle solution Explanation w/ Easy Code + Example | LeetCode 118 | Learn Overflow
Комментарии